In this user guide, we will learn how to create a web server that will send email alerts based on a certain temperature threshold set by the user. The web server will allow the user to change the threshold value, set the recipient’s email address and a choice to enable the email notification. The emails will be sent using the ESP32 development board through an SMTP server. We also have another dedicated tutorial related to ESP32 Send Emails (Plain text, HTML, and Attachments) through SMTP Server in Arduino IDE.
We will connect the DS18B20 temperature sensor with our ESP32 board to access the current temperature readings. One of the advantages of using this sensor is that we will only require a single pin of our ESP32 board to transfer data. Therefore, it is extremely convenient to use it with the micro-controller as we can measure multiple temperatures by using the least number of pins on our development board. You can use any other temperature sensor as well including LM35, thermistor, DS18S20, DHT11/DHT22 and various others.

Project Overview
We will build a web server that will allow us to set a temperature threshold, recipient email and enablement of email notifications. We aim to send email alerts when the temperature goes over or under the threshold value. The ESP32 module will be connected to a DS18B20 sensor which will display current temperature readings on the web server.

Inside the web page, there will heading ‘DS18B20 Current Temperature Reading’. The current temperature value in degrees Celsius will be displayed beneath it. Then there will be another heading called ‘Email Alert Notifications’. Underneath it, there will be various options for the user relating to the email alerts. Firstly, there will be a box in which the user will specify the recipient’s email address. Secondly, the user will have the option to enable the email alert notifications. Lastly, there will be an input field where the user will specify the threshold temperature. Whenever the temperature value will go above or below this value, an email alert will be sent to the recipient. After you click the submit button, the settings will then come in place.
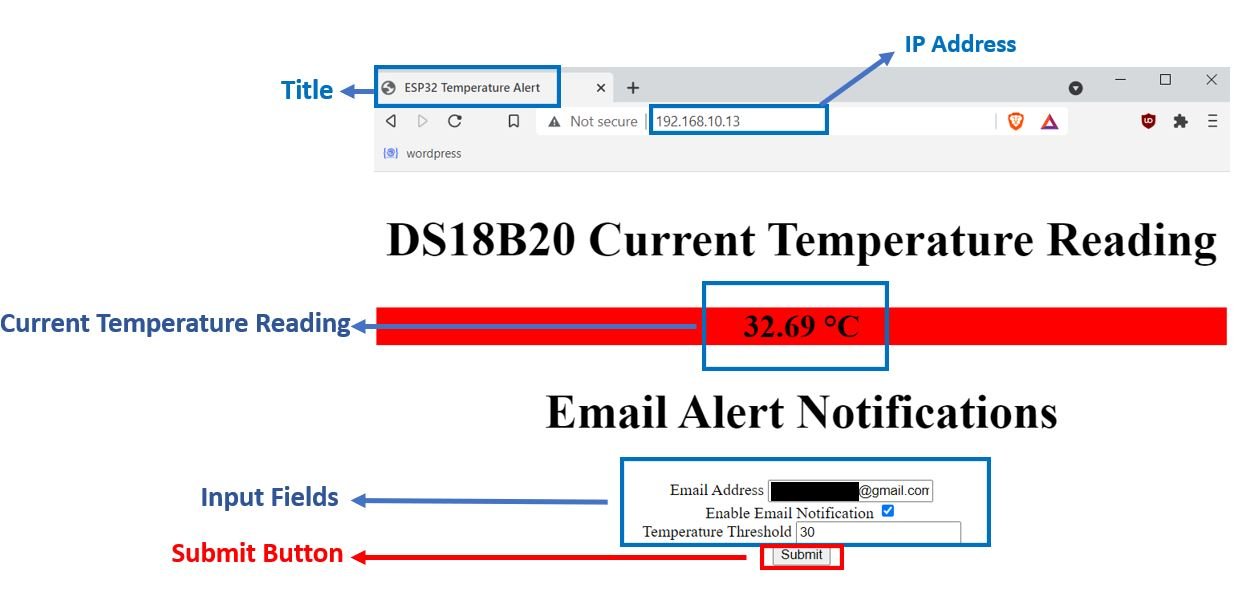
Required Components
For this project we will require the following components:
- ESP32 board
- DS18B20 temperature sensor
- 4.7k ohm resistor
- Connecting wires
- Breadboard
Schematic Diagram
Follow the schematic diagram below, to connect the DS18B20 sensor with your ESP32 module:

As you can see above, we have powered the sensor using the normal mode. The DS18B20 sensor has three terminals. The first terminal is grounded with the ESP32 board. The data line of the sensor, which is the middle terminal, is connected through GPIO14 through a pull-up resistor of 4.7k-ohm on one side and the other side from 3.3V. You can choose any other GPIO pin as well. The third terminal is powered by 3.3V from the ESP board.
Setting up Arduino IDE
We will use Arduino IDE to program our ESP32 development board. Thus, you should have the latest version of Arduino IDE. Additionally, you also need to install the ESP32 plugin. If your IDE does not have the plugin installed you can visit the link below:
Installing ESP32 library in Arduino IDE and upload code.
Installing DS18B20 Libraries
To use the Dallas DS18B20 sensor we will have to install two libraries.
- OneWire library
- DallasTemperature library
Follow the steps below to successfully install them.
We will use the Library Manager in our Arduino IDE to install the latest versions of the libraries. Open your Arduino IDE and go to Sketch > Include Libraries > Manage Libraries. Type each library name in the search bar and install them both.


Installing ESPAsyncWebServer Library and Async TCP Library
To build our asynchronous web server we will require the following libraries:
- ESPAsyncWebServer library
- AsyncTCP library
The ESPAsyncWebServer library will help us in creating our web server easily. With this library, we will set up an asynchronous HTTP server. AsyncTCP library will also be incorporated as it a dependency for the ESPAsyncWebServer library. Both of these libraries are not available in the Arduino library manager. Therefore, we will have to download and load them on our ESP32 board ourselves.
- To install the ESPAsyncWebServer library for free, click here to download. You will download the library as a .zip folder which you will extract and rename as ‘ESPAsyncWebServer.’ Then, transfer this folder to the installation library folder in your Arduino IDE.
- To install the Async TCP library for free, click here to download. You will download the library as a .zip folder which you will extract and rename as ‘AsyncTCP.’ Then, transfer this folder to the installation library folder in your Arduino IDE.
Likewise, you can also go to Sketch > Include Library > Add .zip Library inside the IDE to add the libraries as well.
Installing ESP Mail Client Library
We will use an SMTP server with our ESP32 board to send emails. Simple Mail Transfer Protocol server or SMTP server for short is responsible for sending and receiving emails between the senders and the receivers. Each email provider e.g., Gmail, Yahoo, Hotmail, Outlook etc. has a unique SMTP address and settings.
To use SMTP with our ESP board we will require a library. We will install the ESP Mail Client library from Arduino Library Manager. Go to Sketch > Include Libraries > Manage Libraries. Type ‘ESP32 mail client’ in the search bar and press enter. Install the library highlighted below.

With the help of this library, the user can send/receive emails using the ESP32 module easily.
Setting up Gmail Account
To send emails using our ESP32 board we would require two email accounts. One for the sender and one for the recipient. You can use your email account as the sender account but it is recommended to create a new one just in case something goes wrong in the program code. For this article, we will use Gmail as the primary email provider. You can use any other provider according to your preference.
Creating a new account in Gmail (Sender side) for ESP32
For this tutorial, we have created a new Gmail account and we also recommend you create a new Gmail account. Because if something wrong happens with your ESP32 code and you may incidentally make too many email requests from your personal account, Google may ban your account or block it temporarily. The receiver’s email address can be your personal email address.
To create a Google account:
- Go to the Google Sign Up page: https://www.google.com/gmail/about/ in the required information, such as first and last name, desired email address, password, phone number, and date of birth.
- Click “Next” to proceed with the verification process.
- Read and accept the terms of service and privacy policy.
- Click “Create Account.”

Now type all the relevant information and click ‘Next’. You can give in details according to your preference.

Proceed with all the steps accordingly to create your account successfully.
Create an App Password
To send an email with ESP32, first, you need to create an App password using your Gmail account. An app password in Gmail is a unique password generated for an app or device that does not support 2-step verification. When 2-step verification is enabled for a Gmail account, users are required to enter a verification code in addition to their password when signing in. However, some apps or devices may not be able to prompt for the verification code. In these cases, users can generate an app password to use instead of their regular password for accessing Gmail on the app or device. The app password is only valid for a specific app or device and can be revoked at any time from the Google Account security settings.
First, enable 2-step verification on your account by following these steps:
- Log in to your Google Account.
- Go to the “Security” section in your Google Account settings.
- Scroll down to “Signing in to Google.”
- Under “2-Step Verification,” click “Get Started.”
- Follow the prompts to set up 2-step authentication, which may include adding a phone number to receive verification codes via text or voice call.
- After setting up 2-step authentication, you will be prompted to enter a verification code whenever you sign in to your Google Account on a new device or browse
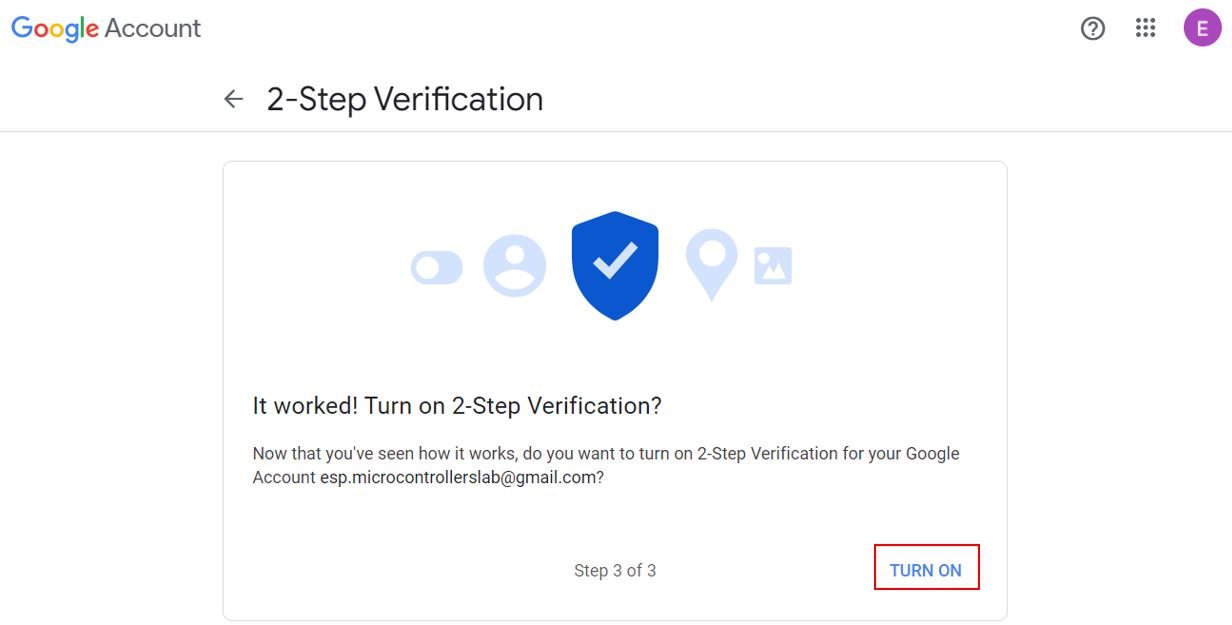
To get an app password:
Log in to your Google Account and Go to the “Security” section in your Google Account settings.

Scroll down to “Signing in to Google.” and Click “App Passwords.”
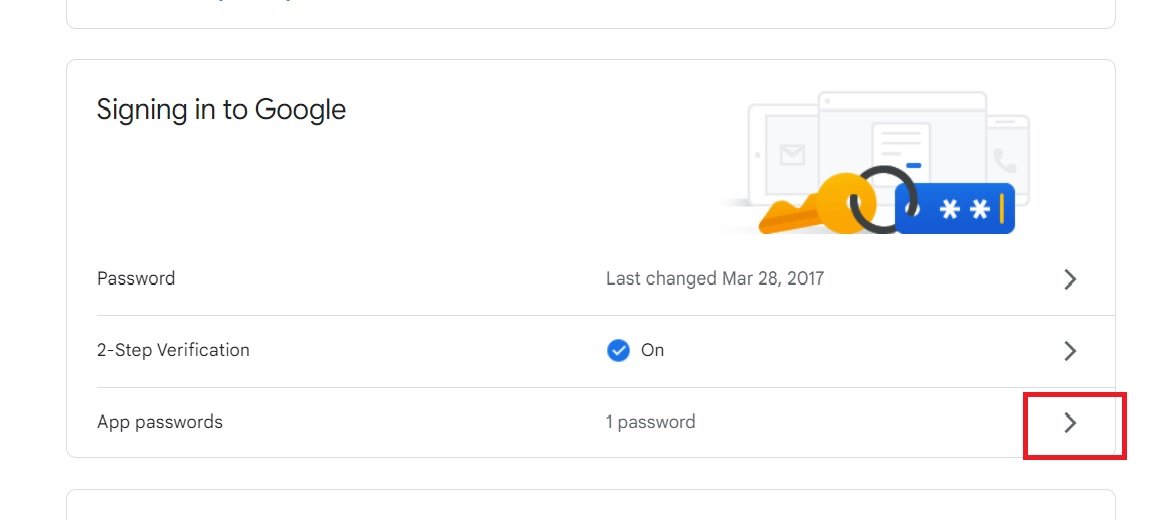
Follow the prompts to generate a unique password for the app you want to use with your Google Account. From the select app field, chose mail and device as other. Give any name to this device. After that click on generate.

It will generate an app password for this specific device. Save this for later use. Because we will use it inside our code instead of the Gmail password.

SMTP Server Settings for Gmail Server
You can view the Gmail server settings as shown below. Knowing this is important because we have to include them in our program code to configure our account properly.
- Server: smtp.gmail.com
- Sender Username: the complete email address of the sender account.
- Sender Password: the password of the sender account
- Server port (TLS): 587
- Server port (SSL): 465
- SMTP TLS/SSL required: yes
If you are using a different email provider e.g., outlook or yahoo you can access its SMTP server settings online.
Arduino Sketch for ESP32 Email Alerts Web Server
Open your Arduino IDE and go to File > New. A new file will open. Copy the code given below in that file and save it. You need to enter your network credentials. Additionally, you also have to provide your SMTP server parameters, the sender’s email details, the recipient’s email address and the threshold temperature value.
#include <WiFi.h>
#include <AsyncTCP.h>
#include <ESPAsyncWebServer.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#include "ESP32_MailClient.h"
const char* ssid = "Your_SSID;
const char* password = "Your_Password";
#define sender_email " Write_Sender_Email_address "
#define sender_email_password " Write_Sender_Email_Password "
#define SMTP_Server "smtp.gmail.com"
#define SMTP_Server_Port 465
#define email_subject "ESP32 Temperature ALERT!"
String Recipient_email = " Write_recipient_Email_address @gmail.com ";
String enableEmailChecked = "checked";
String Notification_enabled = "true";
String Threshold_temp = "30";
String last_temperature;
const char index_html[] PROGMEM = R"rawliteral(
<!DOCTYPE HTML><html><head>
<title>ESP32 Temperature Alert</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
html {font-family: Times New Roman; display: inline-block; text-align: center;}
h2 {font-size: 3.0rem;}
h3 {font-size: 2.0rem; background-color: #FF0000;}
</style>
</head><body>
<h2>DS18B20 Current Temperature Reading</h2>
<h3>%TEMPERATURE% °C</h3>
<h2>Email Alert Notifications</h2>
<form action="/get">
Email Address <input type="email" name="email_input" value="%EMAIL_INPUT%" required><br>
Enable Email Notification <input type="checkbox" name="enable_email_input" value="true" %ENABLE_EMAIL%><br>
Temperature Threshold <input type="number" step="0.1" name="threshold_input" value="%THRESHOLD%" required><br>
<input type="submit" value="Submit">
</form>
</body></html>)rawliteral";
void notFound(AsyncWebServerRequest *request) {
request->send(404, "text/plain", "Not found");
}
AsyncWebServer server(80);
String processor(const String& var){
if(var == "TEMPERATURE"){
return last_temperature;
}
else if(var == "EMAIL_INPUT"){
return Recipient_email;
}
else if(var == "ENABLE_EMAIL"){
return enableEmailChecked;
}
else if(var == "THRESHOLD"){
return Threshold_temp;
}
return String();
}
bool Email_sent = false;
const char* input_parameter1 = "email_input";
const char* input_parameter2 = "enable_email_input";
const char* input_parameter3 = "threshold_input";
unsigned long previousMillis = 0;
const long interval = 10000; //after every 10 seconds new sensor readings will be updated
const int oneWireBus = 14;
OneWire oneWire(oneWireBus);
DallasTemperature sensors(&oneWire);
SMTPData smtpData;
void setup() {
Serial.begin(115200);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
if (WiFi.waitForConnectResult() != WL_CONNECTED) {
Serial.println("Connecting...");
return;
}
Serial.println();
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
sensors.begin();
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/html", index_html, processor);
});
server.on("/get", HTTP_GET, [] (AsyncWebServerRequest *request) {
if (request->hasParam(input_parameter1)) {
Recipient_email = request->getParam(input_parameter1)->value();
if (request->hasParam(input_parameter2)) {
Notification_enabled = request->getParam(input_parameter2)->value();
enableEmailChecked = "checked";
}
else {
Notification_enabled = "false";
enableEmailChecked = "";
}
if (request->hasParam(input_parameter3)) {
Threshold_temp = request->getParam(input_parameter3)->value();
}
}
else {
Recipient_email = "No message sent";
}
Serial.println(Recipient_email);
Serial.println(Notification_enabled);
Serial.println(Threshold_temp);
request->send(200, "text/html", "HTTP GET request sent to your ESP.<br><a href=\"/\">Return to Home Page</a>");
});
server.onNotFound(notFound);
server.begin();
}
void loop() {
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
sensors.requestTemperatures();
float temperature = sensors.getTempCByIndex(0);
Serial.print(temperature);
Serial.println(" *C");
last_temperature = String(temperature);
if(temperature > Threshold_temp.toFloat() && Notification_enabled == "true" && !Email_sent){
String emailMessage = String("Temperature above threshold. Current temperature: ") +
String(temperature) + String("C");
if(sendEmailNotification(emailMessage)) {
Serial.println(emailMessage);
Email_sent = true;
}
else {
Serial.println("Email failed to send");
}
}
else if((temperature < Threshold_temp.toFloat()) && Notification_enabled == "true" && Email_sent) {
String emailMessage = String("Temperature below threshold. Current temperature: ") +
String(temperature) + String(" C");
if(sendEmailNotification(emailMessage)) {
Serial.println(emailMessage);
Email_sent = false;
}
else {
Serial.println("Email failed to send");
}
}
}
}
bool sendEmailNotification(String emailMessage){
smtpData.setLogin(SMTP_Server, SMTP_Server_Port, sender_email, sender_email_password);
smtpData.setSender("ESP32", sender_email);
smtpData.setPriority("High");
smtpData.setSubject(email_subject);
smtpData.setMessage(emailMessage, true);
smtpData.addRecipient(Recipient_email);
if (!MailClient.sendMail(smtpData)) {
Serial.println("Error sending Email, " + MailClient.smtpErrorReason());
return false;
}
smtpData.empty();
return true;
}
How the Code Works?
Including Libraries
Firstly, we will include the necessary libraries required for this project. WiFi.h will help in establishing the connection between the ESP32 module to a wireless network. We will include the relevant libraries which we previously installed to create the web server and also for the DS18B20 sensor’s functionality. Additionally, with the help of the ESP32 Mail Client library, we will successfully send emails via the SMTP server.
#include <WiFi.h>
#include <AsyncTCP.h>
#include <ESPAsyncWebServer.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#include "ESP32_MailClient.h"
Defining Network credentials
Next, we will define the network credentials. One for the SSID and the other for the password. These will be our network credentials which will be used to connect to our wireless network. Replace both of them with your credentials to ensure a successful connection.
const char* ssid = "Your_SSID";
const char* password = "Your_Password";
Defining Server Settings
Next, we will define the server settings as shown below. We will specify the SMTP server, port, the sender’s email address, and password. The sender’s account details are the ones which we newly created. Make sure that you have granted access to less secure apps for the sender account.
We will also specify the subject of the email. You can use your preferred text. In our case, we have set the subject to ‘ESP32 Temperature ALERT!’.
#define sender_email " Write_Sender_Email_address "
#define sender_email_password " Write_Sender_Email_Password "
#define SMTP_Server "smtp.gmail.com"
#define SMTP_Server_Port 465
#define email_subject "ESP32 Temperature ALERT!"
Setting Input Variables
The following variables will be used to save the data the user will input on the webserver. This includes the recipient’s email address in ‘Recipient_email.’ Whether the email notification is enabled or not in the ‘enableEmailChecked.’ By default, we will save it as ticked thus the variable ‘Notification_enabled’ is set to ‘true.’ The ‘Threshold_temp’ will hold the value for the threshold temperature which we are saving as 30 at the moment. All of these three categories could be changed through user input on the web server.
String Recipient_email = " Write_recipient_Email_address @gmail.com ";
String enableEmailChecked = "checked";
String Notification_enabled = "true";
String Threshold_temp = "30";
Additionally, we will create a variable ‘last_temperature’ which will hold the last temperature reading which we will use to compare with the current value.
String last_temperature;
Creating the Web page
We will create the index_html variable to store the HTML text. We will start with the title of the web page. The <title> tag will indicate the beginning of the title and the </tile> tag will indicate the ending. In between these tags, we will specify “ESP32 Temperature Alert” which will be displayed in the browser’s title bar.
<title>ESP32 Temperature Alert</title>
Next, we will create a meta tag to make sure our web server is available for all browsers e.g., smartphones, laptops, computers etc.
<meta name="viewport" content="width=device-width, initial-scale=1">
CSS Styling Web Page
CSS is used to give styles to a web page. To add CSS files in head tags, we will use <style></style> tags to mark the beginning and the end. We will set the display text to font type Times New Roman and align it in the center of the webpage. The font size of the headings as indicated by h2 and h3 will also be set. We are using red as the background colour on which the current temperature reading will be displayed.
<style>
html {font-family: Times New Roman; display: inline-block; text-align: center;}
h2 {font-size: 3.0rem;}
h3 {font-size: 2.0rem; background-color: #FF0000;}
</style>
HTML Web Page Body
The next step will be to define the HTML web page body. This will go inside the <body></body> tags which mark the beginning and the ending of the script. This part will include the headings of the web page and the input fields. We will include the first heading of our webpage inside the <h2></h2> tags and it will be “DS18B20 Current Temperature Reading.”
<h2>DS18B20 Current Temperature Reading</h2>
Then, we will create another heading underneath it which will display the current temperature reading. We will use a placeholder to monitor the current temperature reading. %TEMPERATURE% will be used as the placeholder which will be replaced by the current temperature reading in the processor() function. Degree Celsius will also be displayed with the reading.
<h3>%TEMPERATURE% °C</h3>
The third heading will be “Email Alert Notifications.”
<h2>Email Alert Notifications</h2>
Underneath the heading, we will add the user inputs which need to be filled. This will be of the type ‘form.’ We will define its action as “/get” which means an HTTP GET request will be sent when the user will input all the fields and press the submit button. The form will consist of three input boxes and a submit button. The first input box is the “Email Address” and of type email. The second input box is “Enable Email Notification” and of type checkbox. Lastly, the third input box is “Temperature Threshold” and of type number.
<form action="/get">
Email Address <input type="email" name="email_input" value="%EMAIL_INPUT%" required><br>
Enable Email Notification <input type="checkbox" name="enable_email_input" value="true" %ENABLE_EMAIL%><br>
Temperature Threshold <input type="number" step="0.1" name="threshold_input" value="%THRESHOLD%" required><br>
<input type="submit" value="Submit">
</form>
Creating the AsyncWebServer Object
The AsyncWebServer object will be used to set up the ESP32 web server. We will pass the default HTTP port which is 80, as the input to the constructor. This will be the port where the server will listen to the requests.
AsyncWebServer server(80);
processor() function
Inside the processor() function all the placeholders will get replaced with values saved in their particular variables. Through if-elseif statements, it will check for all three input fields placeholders (EMAIL_INPUT, ENABLE_EMAIL, THRESHOLD) and TEMPERATURE. These will be replaced accordingly with the actual values.
String processor(const String& var){
if(var == "TEMPERATURE"){
return last_temperature;
}
else if(var == "EMAIL_INPUT"){
return Recipient_email;
}
else if(var == "ENABLE_EMAIL"){
return enableEmailChecked;
}
else if(var == "THRESHOLD"){
return Threshold_temp;
}
return String();
}
Setting Input Parameters
We will pass three global variables of type char. These will be the input parameters for the three input fields on our web server. They will be used to monitor if an HTTP GET request was received from any of the input fields. If it was the case then the new updated values will get saved in each variable accordingly. They are ‘email_input,’ ‘enable_email_input,’ and ‘threshold_input’ respectively.
const char* input_parameter1 = "email_input";
const char* input_parameter2 = "enable_email_input";
const char* input_parameter3 = "threshold_input";
Delay
We will incorporate a delay of 10 seconds between accessing new temperature readings from the DS18B20 sensor. This is saved in the variable ‘interval.’ Basically, after every 10 seconds, an updated temperature value will display on the web server.
unsigned long previousMillis = 0;
const long interval = 10000;
Defining DS18B20 sensor parameters
Next, we will create a variable to store the GPIO pin through which the DS18B20 sensor’s data pin is connected. We have used GPIO14 in this example.
const int oneWireBus = 14;
We will require the following instances as well to access the temperature readings. First, we will create a oneWire instance and use the ‘SensorDataPin’ as an argument inside it. Then we will call the DallasTemperature sensor and pass the oneWire reference which we created as an argument inside it.
OneWire oneWire(oneWireBus);
DallasTemperature sensors(&oneWire);
Creating SMTPData object
Furthermore, we will create a SMTPData object called ‘smtpData.’ This will be used later on in the program code while setting the email configurations.
SMTPData smtpData;
Setup() function
Inside the setup() function, we will open a serial connection at a baud rate of 115200.
Serial.begin(115200);
The following section of code will connect our ESP32 board with the local network whose network credentials we already specified above. We will first set the ESP32 module in station mode and then use the WiFi.begin() function. The arguments will be the SSID and the password which we defined earlier in the code. After a successful connection is established, the IP address gets displayed on the web server. We will use this IP address to access our web server.
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
if (WiFi.waitForConnectResult() != WL_CONNECTED) {
Serial.println("Connecting...");
return;
}
Serial.println();
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
Moreover, we will call sensors.begin() to initialize the DS18B20 sensor as well.
sensors.begin();
ESP32 Handling Requests
In this section, we will discuss how our ESP board will handle the requests on the different URLs.
/root URL
Firstly, we will deal with the /root URL request which the ESP board will receive.
We will use the send_P() method. This method will take in four parameters. The first is 200 which is the HTTP status code for ‘ok’. The second is “text/html” which will correspond to the content type of the response. The third input is the text saved on the index_html variable which will be sent. We are replacing the placeholders with current values which will be displayed in the processor() function thus both the HTML page as well as the processor() function will be sent.
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/html", index_html, processor);
});
/get URL
Whenever the user enters any data in the input fields and presses the submit button, an HTTP GET request is sent on the ‘/get’ URL. The following lines of code will check whether the request which was received on the particular URL contains the input parameters which we initially defined. The values entered by the user for the recipient’s email address, threshold temperature and notification enablement get saved in their respective variables.
server.on("/get", HTTP_GET, [] (AsyncWebServerRequest *request) {
if (request->hasParam(input_parameter1)) {
Recipient_email = request->getParam(input_parameter1)->value();
Notification_enabled = request->getParam(input_parameter2)->value();
enableEmailChecked = "checked";
}
else {
Notification_enabled = "false";
enableEmailChecked = "";
}
if (request->hasParam(input_parameter3)) {
Threshold_temp = request->getParam(input_parameter3)->value();
}
}
else {
Recipient_email = "No message sent";
}
Serial.println(Recipient_email);
Serial.println(Notification_enabled);
Serial.println(Threshold_temp);
request->send(200, "text/html", "HTTP GET request sent to your ESP.<br><a href=\"/\">Return to Home Page</a>");
});
When the user clicks the submit button the send() method will be used to return the HTTP response. It takes in three parameters. The first parameter is the response code which we will specify as 200. It is the HTTP response code for ok. The second parameter is the content type of the response which we will specify as “text/html” and the third parameter is the actual message which we will be sent as the HTTP response. This is set as “HTTP GET request sent to your ESP.<br><a href=\”/\”>Return to Home Page</a>”. The arrow operator will be used to call the send method on the AsyncWebServerRequest object. A new web page will open which will contain a link to go back to the main web page.
request->send(200, "text/html", "HTTP GET request sent to your ESP.<br><a href=\"/\">Return to Home Page</a>");
To start the server, we will call begin() on our server object.
server.begin();
loop() function
Inside the loop() function, we will access temperature readings after every 10 seconds and send email alerts based on the temperature threshold.
The following lines of code check for the delay between the sensor readings and display them on the serial monitor after every 10 seconds. Firstly, we will call the requestTemperatures() method. Then we will use the getTempCByIndex() function to retrieve the temperature in degree Celsius. Notice that we are passing 0 as a parameter inside the function. This is because we are using a single DS18B20 sensor.
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
sensors.requestTemperatures();
float temperature = sensors.getTempCByIndex(0);
Serial.print(temperature);
Serial.println(" *C");
last_temperature = String(temperature);
Now, through if-else statements, we will check if the current temperature reading is above or below the threshold temperature set by the user. An email alert will only be sent if all three conditions are met. Firstly, the current temperature should be above or below the threshold temperature. Secondly, the email notification should be enabled i.e., the user ticks it on the web server. Lastly, the email is not yet sent.
The content of the email will depend on whether the current temperature reading was above or below the threshold set by the user. If the temperature is above the threshold value the email will state ‘Temperature above threshold’ with the current temperature reading beside it. Likewise, if the temperature is below the threshold value the email will state ‘Temperature below threshold’ with the current temperature reading beside it.
if(temperature > Threshold_temp.toFloat() && Notification_enabled == "true" && !Email_sent){
String emailMessage = String("Temperature above threshold. Current temperature: ") +
String(temperature) + String("C");
if(sendEmailNotification(emailMessage)) {
Serial.println(emailMessage);
Email_sent = true;
}
else {
Serial.println("Email failed to send");
}
}
else if((temperature < Threshold_temp.toFloat()) && Notification_enabled == "true" && Email_sent) {
String emailMessage = String("Temperature below threshold. Current temperature: ") +
String(temperature) + String(" C");
if(sendEmailNotification(emailMessage)) {
Serial.println(emailMessage);
Email_sent = false;
}
else {
Serial.println("Email failed to send");
}
}
}
}
sendEmailNotification() function
The sendEmailNotification() function sets all the parameters for the email and configures the session. It contains details of the email such as sender’s name, sender’s email, priority, and the subject of the email. Through an, if statement it will check if the email was sent successfully or not. If it was then it will return true otherwise it will return false.
bool sendEmailNotification(String emailMessage){
smtpData.setLogin(SMTP_Server, SMTP_Server_Port, sender_email, sender_email_password);
smtpData.setSender("ESP32", sender_email);
smtpData.setPriority("High");
smtpData.setSubject(email_subject);
smtpData.setMessage(emailMessage, true);
smtpData.addRecipient(Recipient_email);
if (!MailClient.sendMail(smtpData)) {
Serial.println("Error sending Email, " + MailClient.smtpErrorReason());
return false;
}
smtpData.empty();
return true;
}
ESP32 Email Alter Web Server Demonstration
Choose the correct board and COM port before uploading your code to the board. Go to Tools > Board and select ESP32 Dev Module.
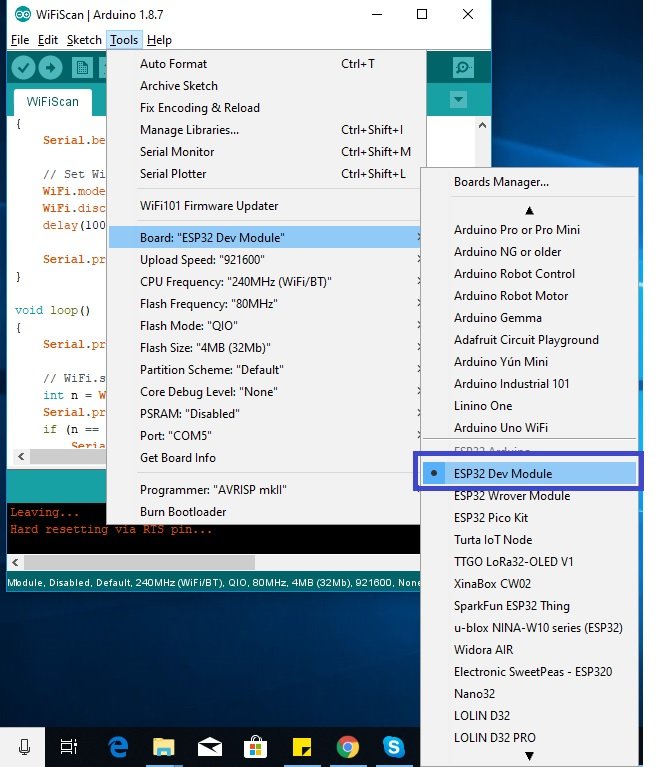
Next, go to Tools > Port and select the appropriate port through which your board is connected.
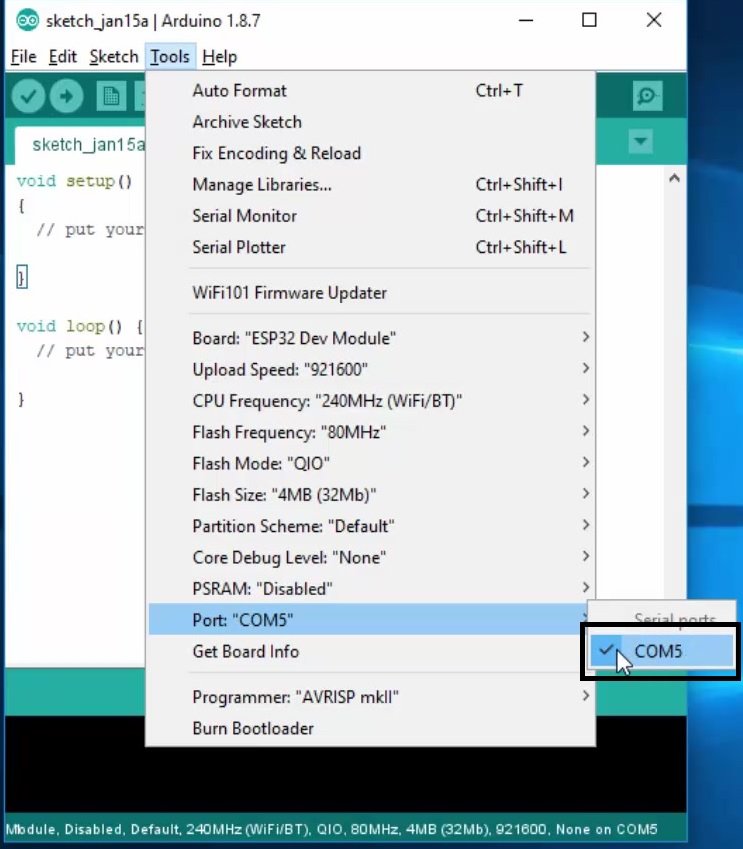
Click on the upload button to upload the code into the ESP32 development board. After you have uploaded your code to the development board, press its ENABLE button.
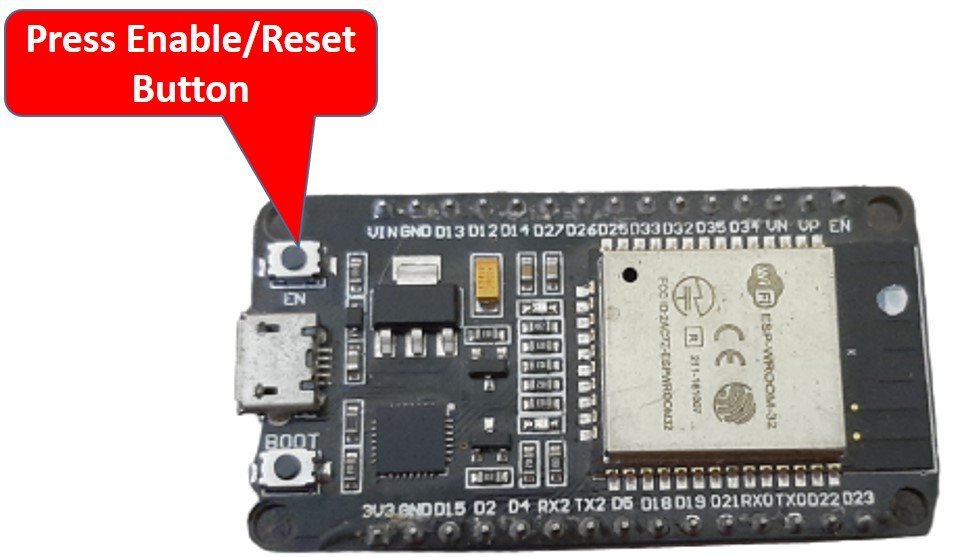
In your Arduino IDE, open up the serial monitor and you will be able to see the IP address of your ESP module. New temperature readings will also display after every 10 seconds.

Type that IP address in a web browser and press enter.
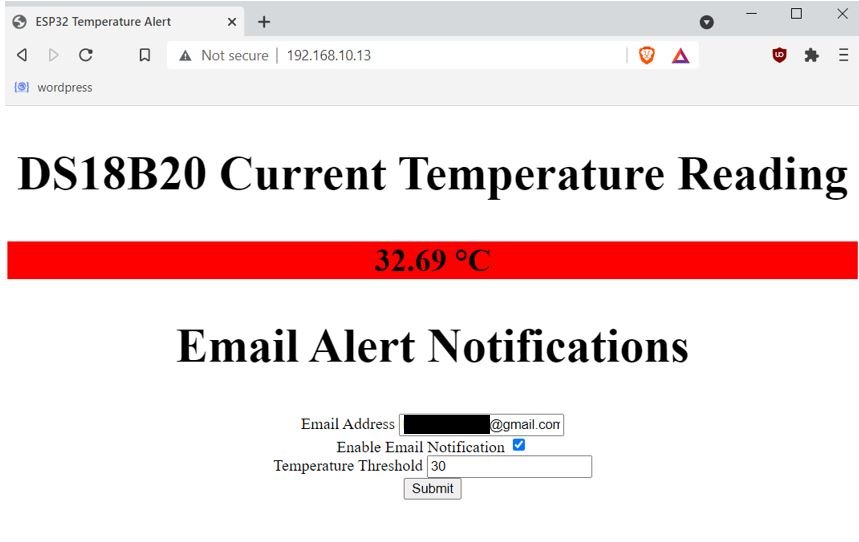
Now, fill the input fields or leave them as default. As our current temperature is higher than the set threshold value and we had enabled the email notification hence we received an email.

Now, we changed the threshold value to 45 degrees Celsius keeping the other fields the same. Press the submit button for the changes to take place.
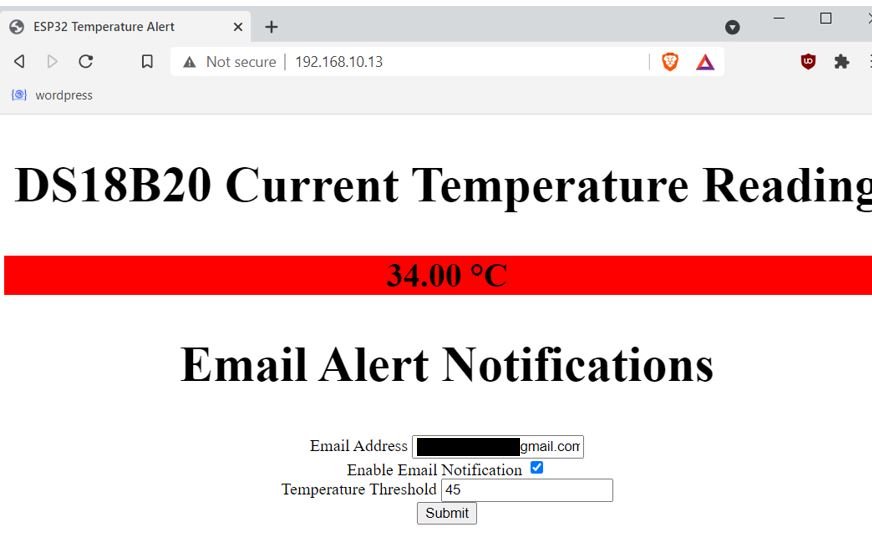
We will be redirected to a new web page as shown below. Click the link below to redirect to the main web page.

In the serial monitor, you can view the changes which took place.
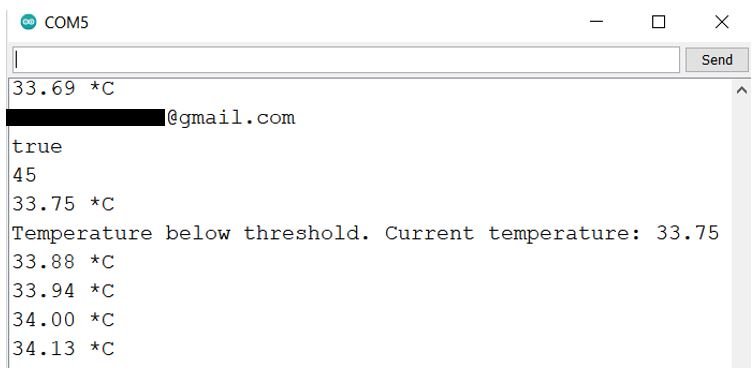
Additionally, we will receive another email stating that the temperature is below the threshold value.

Conclusion
In conclusion, we were able to send email alerts via the SMTP server and ESP32 whenever the current temperature went above or below the threshold temperature. You can use any appropriate sensor with your ESP32 board to receive similar alert messages such as BME680, BME280, DHT11, MPU6050 and DHT22.
You may also like to read:
- ESP32/ESP8266 Control Outputs with Web Server and Push Button Simultaneously
- ESP32 Send Sensor Readings to Google Firebase and Build an Android app to display Data
- Control ESP32 Outputs using Google Assistant and Adafruit IO with Arduino IDE
- ESP32 Send Sensor Readings to ThingSpeak using Arduino IDE (BME280)
- Google Firebase: Control ESP32 Outputs with Arduino IDE