In this tutorial, we will see different integer division instructions supported by 8086 microprocessors. We will also provide assembly program examples of each divide instruction.
In this series of 8086 microprocessor tutorials, we previously discussed; 8086 Microprocessor Addressing Modes, 8086 Data Transfer Instructions, 8086 Integer Arithmetic Instructions, 8086 Integer Multiplication Instructions.
8086 Microprocessor Division Instructions
The following instructions are supported by 8086 microprocessor that are used to perform the division operation:
- DIV (unsigned numbers)
- IDIV (signed numbers)
- AAD
8086 DIV Instruction ( Unsigned Operands)
The DIV instruction performs the division of two unsigned operands. The denominator resides in a source operand and it should not be immediate. However, it can be register or a memory location. There are four division cases depending on the number of bits. The division can be:
- Byte with byte
- Word with word
- Word with byte
- Doubleword with word
Byte with Byte Divsion
In this case, the nominator and denominator operands are bytes. The nominator resides in the AL register and AH is set to zero. After division, the instruction stores quotient in AL and the remainder in AH register.
Assembly Example Code
ORG 100h
.MODEL SMALL
.DATA
NUM_1 DB 0F2H
NUM_2 DB 4H
.CODE
MOV BH, NUM_2 ;Load numerator in BH
MOV AL, NUM_1 ;Load denominator in AL
DIV BH ;Divide BH by AL
RET
Output
The DIV instruction divides BH by AL. F2 divided by 04 gives quotient of 3C and give 02 as a remainder. AL stores the quotient and remainder is stored in AH register.
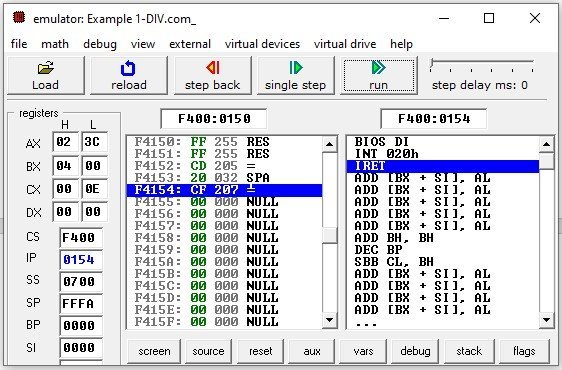
Word with word Division
In this case, the AX register holds the numerator. After division, the quotient is stored in the AX register and the remainder goes to the DX register.
Assembly Example Code
ORG 100h
.MODEL SMALL
.DATA
NUM_1 DW 0F213H
NUM_2 DW 41A8H
.CODE
MOV AX, NUM_1 ;Load numerator in AX
DIV NUM_2 ;Divide AX by NUM_2
RET
Output
The output window shows that the division of F213H by 41A8 gives the remainder of 2D1B and 03 as a quotient.

Word with Byte
The numerator is a 16-bit word stored in AX which is divided with an 8-bit denominator. After division, the AL contains the quotient and AH will contain the remainder.
Assembly Example Code
ORG 100h
.MODEL SMALL
.DATA
NUM_1 DW 1B25H
NUM_2 DB 24H
.CODE
MOV AX, NUM_1 ;Load denominator in AX
DIV NUM_2 ;Divide AX by NUM_2
RET
Output
The NUM_1 is divided by NUM_2 which gives a quotient of C1 and remainder of 01. You can see from the contents of register AX that AH contains the remainder and AL stores the quotient.

Double word by word Divsion
It is the last case of division in which a numerator is a 32-bit number and a denominator is a 16-bit number. In this case, AX and DX stores the numerator. The most significant part resides in the DX register and the least significant bits of numerator are in the AX register.
After the execution of DIV instruction, the remainder goes to DX register and the quotient lie in AX register.
Assembly Example Code
ORG 100h
.MODEL SMALL
.DATA
NUM_1 DW 2413H
.CODE
MOV AX, 5670 ;Load lower bytes of numerator in AX
MOV DX,4531 ;Load highe bytes of numerator in DX
DIV NUM_1 ;Divide AX by NUM_1
RET
Output
Here, the numerator in DX and AX which is 45315670. It is divided by 4531 to give quotient of 7D9A which is in AX register.

Divide Error or Overflow Error
The emulator would generate a type 0 interrupt if:
- In case of a byte with byte and word with byte division, the quotient is greater than FFH.
- In case of a word with word and doubleword by word division, if the quotient is greater than FFFFH.
- When the denominator is 0.
Suppose an example in which numerator is 5BBDH and denominator is 21H.
Example Assembly Code
ORG 100h
.MODEL SMALL
.DATA
NUM_1 DW 5BBDH
NUM_2 DB 21H
.CODE
MOV AX, NUM_1 ;Load denominator in AX
DIV NUM_2 ;Divide AX by NUM_2
RET
Output
In this case, the output window generates an error because the quotient is 2C7 which is greater than the maximum limit of the AL register. The AL register can only hold data up to FFH.

8086 IDIV Instruction ( Signed Operands)
IDIV is an arithmetic instruction that performs a division operation between two signed numbers. The source operand in the instruction is a signed divisor. It can be specified using any addressing mode except immediate mode of addressing. Just like DIV instruction, the IDIV instruction also has four cases which are:
- Byte with byte
- Word with word
- Word with byte
- Doubleword with word
1. Byte with Byte:
For division of a signed byte by signed byte, the numerator is stored in AL register and then it is sign extended to AH register. After division, the quotient goes to AL register and remainder is in AH register. The quotient value should in between +127 to 127 range otherwise it will generate divide error interrupt.
2. Word with word:
In this case the numerator is in AX register and the leftover most significant bits are filled with copies of signed bit. The division generate quotient in AX and remainder in DX. Here, the quotient range is -32767 to +32767.
3. Word with byte:
If the numerator is a 16-bit word and denominator is a byte, then AL and AH registers will store the quotient and remainder. But now in this case, the whole AX register will store the numerator.
4.Doubleword by word:
The last case involves the division of a signed double word with a signed single word. As a double word has 32 bits therefore 16-bit AX register cannot store it. So, DX register will store the most significant bits of the numerator and least significant bits are in AX register. After division AX will store quotient and DX will store remainder.
Example Assembly Code
This example illustrates how all of these instructions modify the data of registers.
ORG 100h
.MODEL SMALL
.DATA
NUM_1 DD 0C250A91H
NUM_2 DW -0B25H
NUM_3 DB 24H
.CODE
;Byte by Byte Signed Division
MOV AL, NUM_3
MOV BL,3
IDIV BL
;Word by word Signed Division
MOV AX, -265H
IDIV NUM_2
;Word by byte Signed Division
MOV AX, NUM_2
IDIV NUM_3
;Doubleword by single word signed division
MOV AX,NUM_1
MOV DX, NUM_1+2
MOV BX, 0A234H
IDIV BX
RET
Output

8086 AAD Instruction
The AAD is a mnemonic for “ASCII Adjust for Division”. This instruction is used before division of two unpacked BCD numbers so that after division, the quotient and remainder produced would be in unpacked BCD form. The AAD instruction executes and multiply AH by 10. Then add the AH to AL and after that set AH to 0. The AAD instruction can modify PF, SF and ZF flags.
Example Assembly Code
ORG 100h
.MODEL SMALL
.CODE
MOV AX,3730H ;ASCII for 70
MOV BL,03H ;Load 03H into BL
AAD
DIV BL
RET
The “MOV AX,3730H” instruction store 37 into the AH register and 30 into the AL register. The instruction AAD will multiply AH by 10 and will add to AL. The AL becomes:
AL=(10)(37)+1C=3
And AH is cleared to 0. After that division instruction is executed to generate quotient and remainder both in unpacked BCD forms.

Which emulator are you using?
emu8086
in division of word by word, it says AX holds the denominator. Well it should be numerator.
Hi Mehak,
Thanks for pointing out a typo, we will fix it.