In this tutorial, we will create soft access point web server with Raspberry Pi Pico W using MicroPython. Firstly, we will learn to use Pico W as a soft access point or in SoftAP mode. The soft access point mode allows devices to connect with our board without a wireless router. At the end, we will create an example web server which can access by device which are connected to this access point.
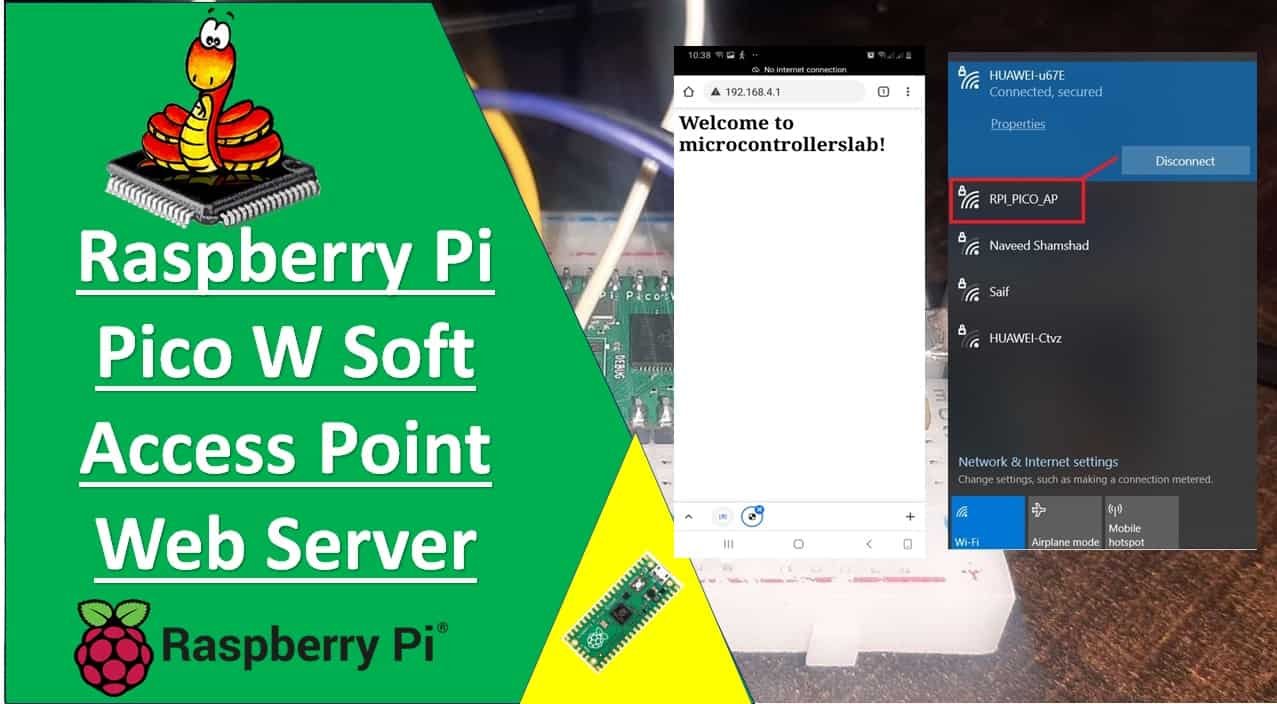
Prerequisites
Before we start this tutorial, make sure you are familiar with and have the latest version Python 3 in your system, have set up MicoPython in Raspberry Pi Pico W, and have a running Integrated Development Environment(IDE) in which we will be doing the programming. We will be using the same Thonny IDE as we have done previously in the getting started guide. If you have not followed our previous tutorial, you check here:
Soft Access Point Introduction
The Soft AP is known as a “software-enabled access point” A soft access point which is also known as a ‘virtual router,’ combines software that sets up an Raspberry Pi Pico W into a wireless access point. There is no connection with a wired network involved hence called SoftAP.
Station Mode vs Soft AP Mode
To create a web server with Raspberry Pi Pico W, we can use either soft access point or station mode as follows:
Raspberry Pi Pico W in Station Mode
In this mode, Raspberry Pi Pico W board connects to your Wi-Fi network through a router. Therefore, the medium of communication between the web client (web browser) and Raspberry Pi Pico W (web server) is the router. Raspberry Pi Pico W boards get the IP address from the Wi-Fi router. With this IP address, web clients can access the Web server through an existing local network.
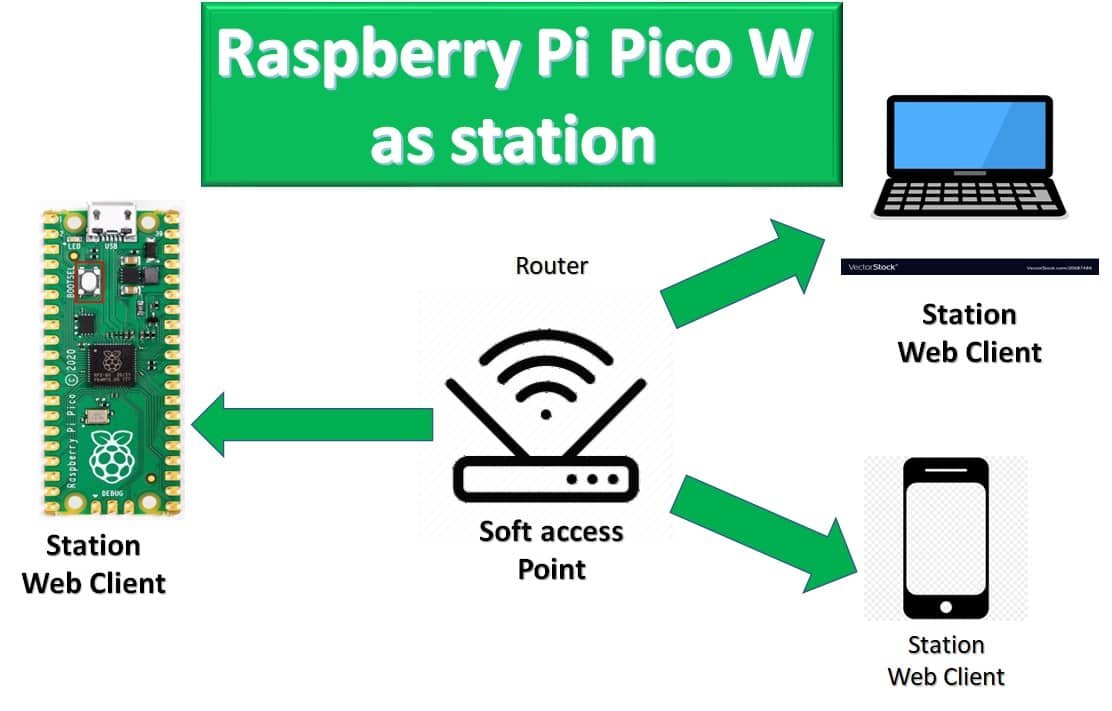
In our previous web server projects with Raspberry Pi Pico W board, we used a router to connect this boards to get access to the local network. In that case, the Pico W module acted as a station client and the router was set up as an access point as shown in the figure below:
Raspberry Pi Pico W in Soft Access Point Mode
In this mode, Raspberry Pi Pico W creates its own wireless Wi-Fi network similar to your existing Wi-Fi router. In this mode, we do not need to connect Raspberry Pi Pico W to the Wi-Fi network. Any user who wants to access the web server should first connect to this wireless Wi-Fi network. Up to 5 devices can connect to this Wi-Fi network.
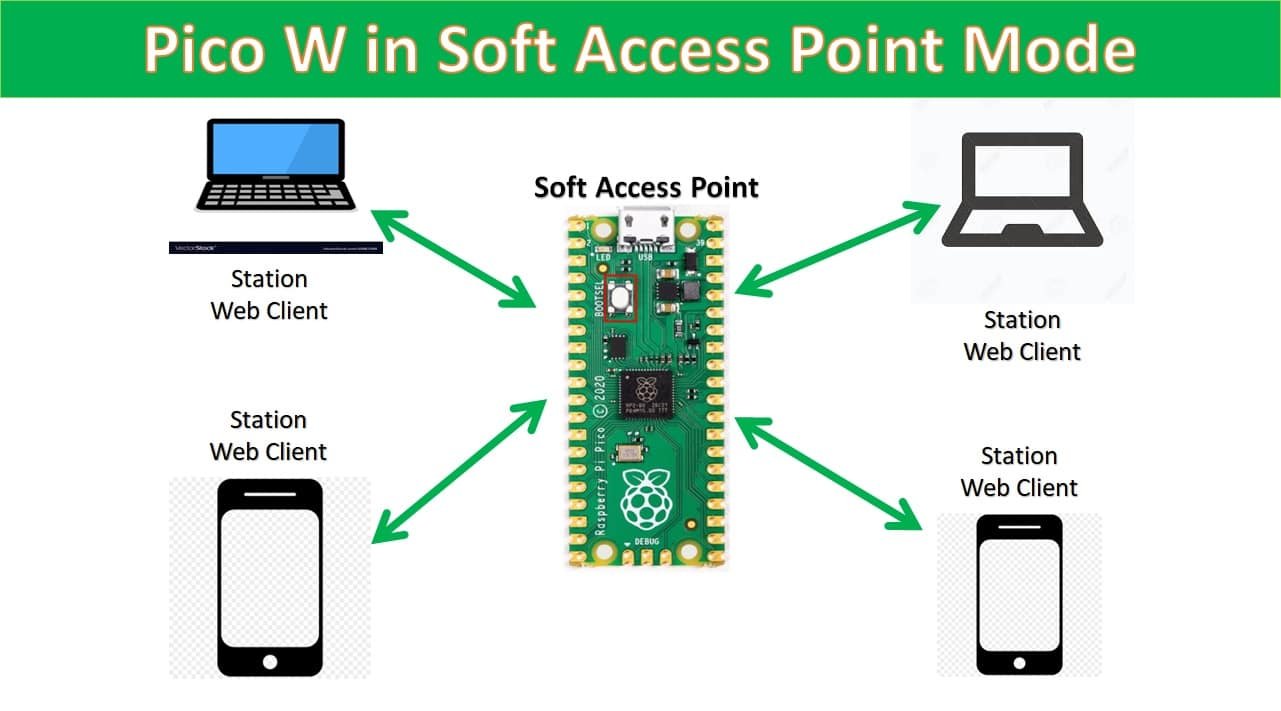
This time we will learn to use Raspberry Pi Pico W as an Access Point. There is a significant advantage of using SoftAP mode because a network router is not required. By setting the Raspberry Pi Pico W board as an Access point, we are basically setting it up as a hotspot. Thus, we can connect all the devices which are supported by Wi-Fi and the router is not even involved. All the Wi-Fi supported devices which are nearby including cell phone, laptop or your personal computer (PC) will be able to connect with our board.
MicroPython API to Set Raspberry Pi Pico W as an Access Point
MicroPython provides network interface module. This network module provides two types of Wi-Fi interfaces. One for the station, when the Raspberry Pi Pico W connects to a router. Similarly, one for the access point that is for other devices to connect to SSID broadcasted by Raspberry Pi Pico W. Because we want to use Pico W in soft access point mode. Therefore, we will use the access point mode interface. We create an instance of this object using the station interface network.WLAN(network.AP_IF).
ap = network.WLAN(network.AP_IF)
The network.WLAN() is used to create a.WLAN network interface object.
Supported interfaces are:
- network.STA_IF (station mode)
- network.AP_IF (Soft access point mode)
After that, activate the access point by passing the “True” argument to the ap.active() method. Here ‘ap’ is an instance of the object which we created in the last step. We will use the same instance to access all methods of network class.
ap .active(True)
We define two variables that hold the name and password of the Raspberry Pi Pico W access point network. The following variables hold SoftAP credentials:
ssid= 'Enter_Acess_Point_Name_Here'
password = 'Enter_Acess_Point_Passoword_Here'
The config() function is used to configure with a given ssid and password.
ap
.config
(essid
=ssid
,password
=password
)
In short, the following MicroPython script set up softAP mode:
ap = network.WLAN(network.AP_IF)
ap.config(essid=ssid, password=password)
ap.active(True)
Raspberry Pi Pico W Access point Web Server using MicroPython
In this section, we will create an example to use Raspberry Pi Pico W in Soft Access Point mode by creating an “Microcontrollerlab” web server.
We will configure Raspberry Pi Pico W in the soft access point mode by going through a simple demonstration of a web server.
MicroPython Script
In your Thonny IDE, create a New File and write down the following code with the name of main.py file. This SoftAP web server prints “Welcome to microcontrollerslab!” on a web page.
try:
import usocket as socket #importing socket
except:
import socket
import network #importing network
import gc
gc.collect()
ssid = 'RPI_PICO_AP' #Set access point name
password = '12345678' #Set your access point password
ap = network.WLAN(network.AP_IF)
ap.config(essid=ssid, password=password)
ap.active(True) #activating
while ap.active() == False:
pass
print('Connection is successful')
print(ap.ifconfig())
def web_page():
html = """<html><head><meta name="viewport" content="width=device-width, initial-scale=1"></head>
<body><h1>Welcome to microcontrollerslab!</h1></body></html>"""
return html
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) #creating socket object
s.bind(('', 80))
s.listen(5)
while True:
conn, addr = s.accept()
print('Got a connection from %s' % str(addr))
request = conn.recv(1024)
print('Content = %s' % str(request))
response = web_page()
conn.send(response)
conn.close()
How Code Works
Setting up the Wi-fi & password
First, set up the name and password of an access point in MicroPython code to let other devices connect to Raspberry Pi Pico W. You can change the ssid and password according to your choice. We have set it as RPI_PICO_AP and the password to an 8-digit number.
ssid = 'RPI_PICO_AP' #Set access point name
password = '12345678' #Set your access point password
Creating an Access point
Now, we will be creating an access point using a MicroPython script. Because we are setting up an Raspberry Pi Pico W board as an access point to which other devices will be able to connect. Therefore, we will select the access point (AP) network interface. We will create an instance of this object using the AP interface which we will call network.AP_IF. The network.WLAN() creates a network interface object in our case network.AP_IF.
ap = network.WLAN(network.AP_IF)
Now, activate softAP mode bypassing the True as a parameter in the ap.active() method.
ap.active(True) #activating
The config() function is used to configure with a given SSID and password.
ap.config(essid=ssid, password=password)
The following lines check whether the AP is activated or not. If not then it will not move to the next step. Otherwise, it will display the IP address of the softAP.
while ap.active() == False:
pass
print('Connection is successful')
print(ap.ifconfig())
Creating a Web page
We will be defining a web page which would display ‘Welcome to microcontrollerslab!’
def web_page():
html = """<html><head><meta name="viewport" content="width=device-width, initial-scale=1"></head>
<body><h1>Welcome to microcontrollerslab!</h1></body></html>"""
return html
Socket Server
To provide a response to web client requests, create a socket server on Raspberry Pi Pico W using Micrpython socket API. We will use a socket.socket() function to initialize our socket server. This function takes in two arguments. The first is the socket_family and the second argument is the socket_type. We will then create a socket object called ‘s’ and pass the first parameter in the function AF_INET transport mechanism and the second parameter as SOCK_STREAM which is the type of the socket.
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Next, we will bind the socket to an IP address and a port number. This is achieved by using the bind() method. In our case, we will pass an empty string followed by ‘80’ to the bind() function. The empty string portrays the default IP address of Raspberry Pi Pico W board and ‘80’ specifies the port number set for the Raspberry Pi Pico W web servers.
s.bind(('', 80))
Then, we will be creating a listening socket to receive requests from the web clients.
s.listen(5)
The accept() method accepts the connection whenever a web client makes a request.
conn,addr=s.accept()
We will be providing the reference of the web_page() function to a variable which we will call ‘response.’ After that, send the content of the web page to a socket client. This will be achieved through send() method whose parameter we will specify as ‘response.’ In the end, in order to stop the connection, we will use the close() method. Keep in mind all of this is occurring inside an infinite while loop.
response = web_page()
conn.send(response)
conn.close()
For in-depth guide on how to create socket server using MicroPython and Raspberry Pi Pico W, you can read our previously published post:
Soft Access Point Web Server Demo
To test SoftAP MicroPython web server code, click on play button on Thonny IDE and you will see the following IP address on Thonny IDE shell console:

That’s mean Raspberry Pi Pico W board has setup as a access point and started broadcasting RPI_PICO_AP ssid. Note down this IP address. Because we will use it to access web page through a browser.
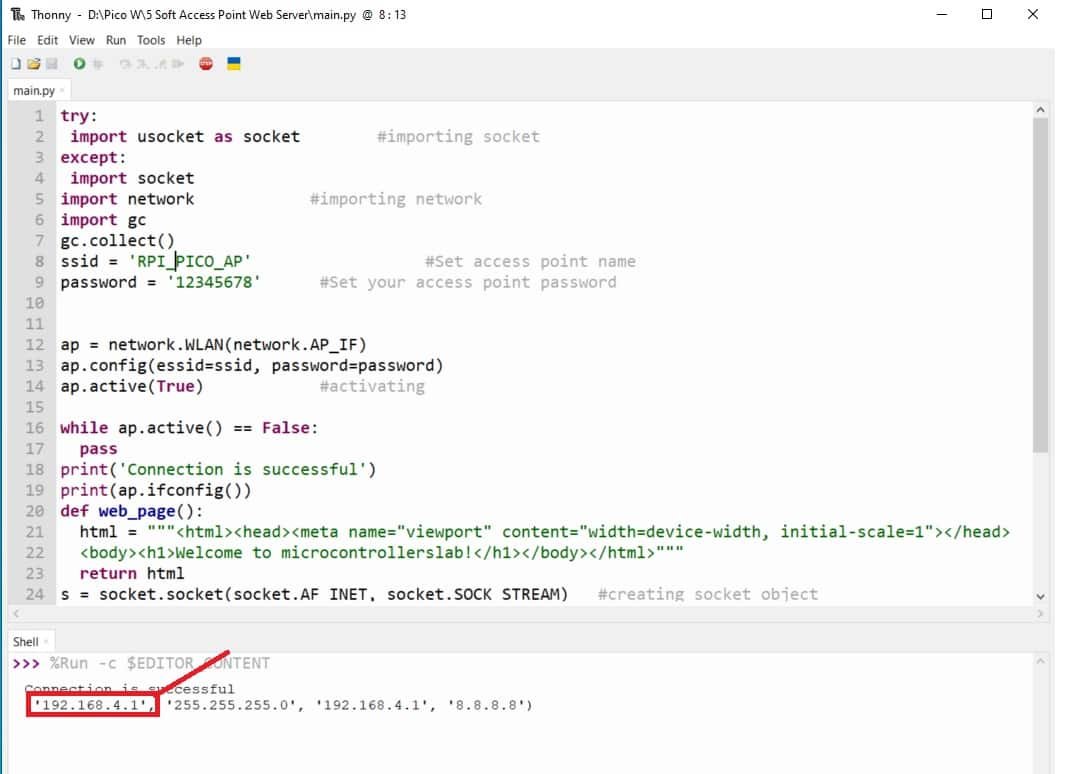
Now connect to soft access point using either your desktop computer or mobile phone. Open Wi-Fi settings in your mobile and you will see RPI_PICO_AP as wireless connection.

Now go to any web browser and enter the IP address http://192.168.4.1 which we noted in last section. You will get the response from Raspberry Pi Pico W as follows:
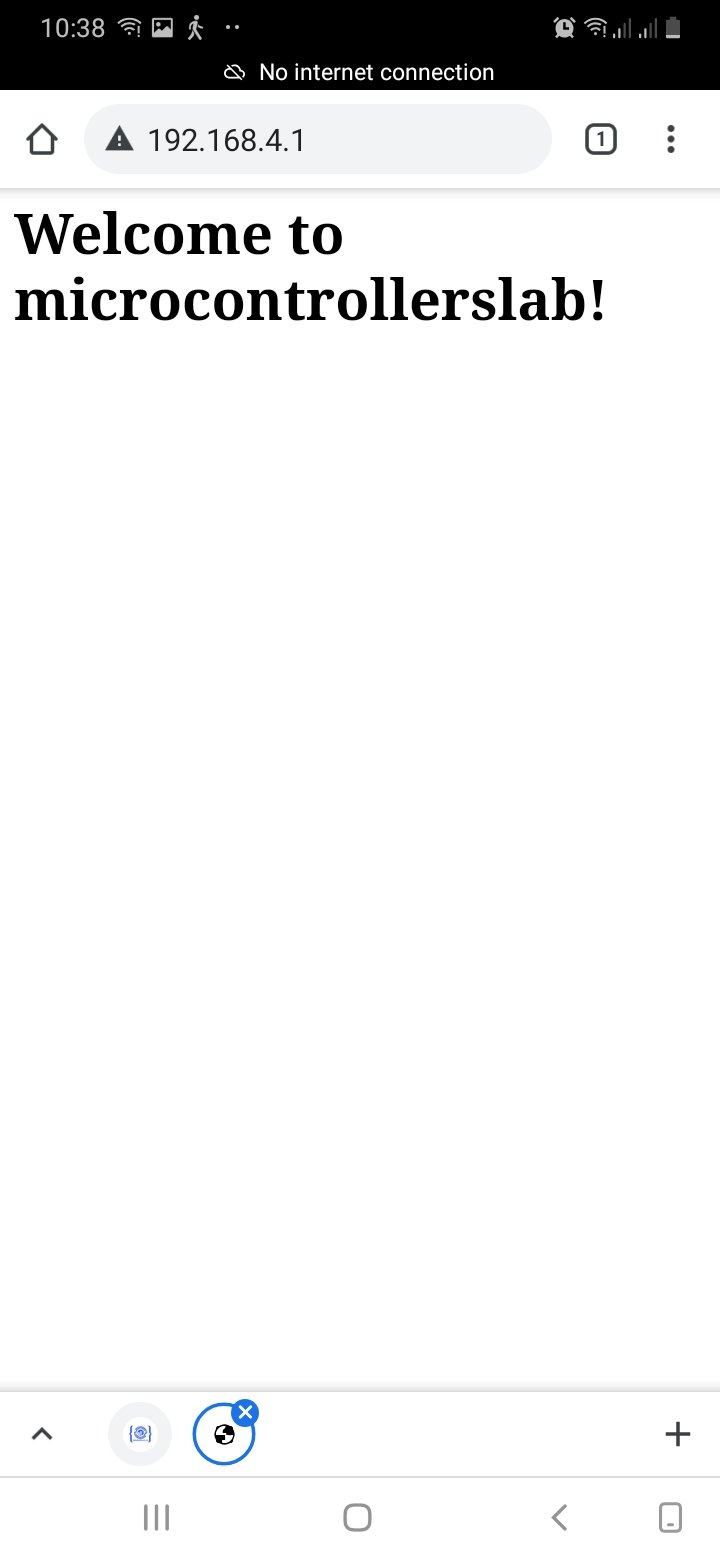
In summary, we have learned to setup Access Point on Raspberry Pi Pico W using MicroPython.
You may also like to read:
Hello, great guide. Very helpful. Only thing is the webpage loads fine for PCs and android devices, but not natively with ios device browsers (Safari, DDG, Firefox) which demand a more secure connection experience.
Anyone has ideas on how to get around this?