In a previous tutorial, we showed you how to send simple Whatsapp text messages to your account with ESP32 using Whatabot and callmebot free APIs. In this guide, we will take that a step ahead and send sensor readings using callmebot and WhataBot API and HTTP client library in Arduino IDE and send the sensor data to our WhatsApp number after every few seconds. Any appropriate sensor such as BME280, BME680, DS18B20, DHT22 and SHT31 can be used but for this article, we will use a BME280 sensor which is used to measure temperature, pressure, and humidity.

We have a similar guide with ESP8266 NodeMCU:
WhatsApp Messenger Introduction
WhatsApp Messenger is a famous, free, and most widely used platform to share instant messages, voice messages, voice calls, video calls, and shared documents owned by an American company known as Meta or Facebook. It is available to download and install
for Android and iOS based smartphones. Anyone can sign up for this service by using his mobile phone number, and users must have an active mobile internet connection to use this service.
This application was initially developed by WhatsApp Inc. of Mountain View, California, and later on, acquired by Facebook (Meta).
CallMeBot and Whatabot WhatsApp API Introduction
The Whatabot and CallMeBot are free and easy-to-use APIs to send WhatsApp messages by only calling an API from our ESP32 using an HTTP client. These APIs are a service that allows you to send yourself messages in real-time. You only have to register your phone and take note of your api key! To send a message is necessary to execute an http GET and POST from your microcontroller/app. It’s easy to use and you can receive real-time alerts in all your projects.
In case of Whatabot, we need to send HTTP GET request and with Callmebot, we send an HTTP POST request.
We will use this service to send messages on our WhatsApp number. It is a gateway service that allows us to send messages to ourselves from ESP32 or any other HTTP client.
How to setup Whatabot API Key
In order to use this service, we need an ApiKey before calling API from the ESP32 development board. Now let’s see how to get API key:
Follow these steps:
- 1) Create the Whatabot contact in your smartphone.
- The phone number is: +54 9 1123998026
- 2) Send: “I allow whatabot to send me messages”
- 3) Copy the link that Whatabot sent you and change the “text” tag of the URL for whatever you want.
- 4) Enjoy Whatabot!
Note: If you don’t receive the ApiKey in 2 minutes, please try again after 24hs.

The WhatsApp message from the bot will contain the apikey needed to send messages using the API. You can send text messages using the API after receiving the confirmation.
If you forgot your api key, send “I allow whatabot to send me messages” again and Whatabot will send you the key you had
- If you want to change your api key, send “I want to update my apikey” and Whatabot will send you a new one. Note that you have to change the key in all your apps!
- If you want to delete your Whatsapp Whatabot account, sent “I want to delete my account”
How to Get CallMeBot API Key
You need to get the API key from the bot before using the API:
- 1. Add the phone number +34 621073245 to your Phone Contacts. (Name it as you wish)
- Send this message “I allow callmebot to send me messages“ to the new Contact created (using WhatsApp of course)
- Wait until you receive the message “API Activated for your phone number. Your APIKEY is 123123” from the bot.
- Note: If you don’t receive the ApiKey in 2 minutes, please try again after 24hs.
The WhatsApp message from the bot will contain the API key needed to send messages using the API.
How to send a WhatsApp text message with Whatabot and CallMeBot ApiKey
Now let’s see the APIs of both services one by once.
WhataBot API
To send a WhatsApp message with Whatabot API, we need to call the following API inside ESP32 code with an HTTP GET request. In later sections of this article, we will see how to call an HTTP GET request with ESP32.
http://api.whatabot.net/whatsapp/sendMessage?text=" + (message) + "&apikey=" + api_key + "&phone=" + mobile_number;
CallMeBot API
To send a WhatsApp message with Callmebot API, we need to call the following API inside ESP32 code with an HTTP POST request.
https://api.callmebot.com/whatsapp.php?phone=[phone_number]&text=[message]&apikey=[your_apikey]
We need to change the following things before calling above APIs:
[phone_number]: This is the phone number which you used to sign-up for WhatsApp. Make sure to include your country code along with your mobile phone number. For example 92 301 22 222 22
[text]: This field is a message that you want to send on your WhatsApp number and it should be URL encoded. We will see later on how to convert messages into URL encoded format. For example in URL encoded format %20 is for space and %0A is for new lines).
[your_apikey]: The apikey is a key that we get from Whatabot mebot API in the last section during the activation process.
Introduction to BME280 sensor
The BME280 sensor is used to measure readings regarding ambient temperature, barometric pressure, and relative humidity. It is mostly used in web and mobile applications where low power consumption is key. This sensor uses I2C or SPI to communicate data with the micro-controllers. Although there are several different versions of BME280 available in the market, the one we will be studying uses the I2C communication protocol and SPI.
Connecting BME280 sensor with the ESP32 development board
The connection of BME280 with the ESP32 board is very easy. We have to connect the VCC terminal with 3.3V, ground with the ground (common ground), SCL of the sensor with SCL of the module, and SDA of the sensor with the SDA pin of the ESP32 module.
The I2C pin in ESP32 for SDA is GPIO21 and for SCL is GPIO22
Required Components
We will need the following components to connect our ESP32 board with the BME280 sensor.
- ESP32 board
- BME280 Sensor
- Connecting Wires
- Breadboard
Schematic Diagram
Connect the ESP32 device with BME280 as shown in the schematic diagram below:

Setting up Arduino IDE
Before we proceed further, you should make sure that you have the latest version of Arduino IDE installed on your system. Moreover, you should also install an ESP32 add-on in Arduino IDE. If your IDE does not have the plugins installed you can visit the link below:
For this project, we will have to install libraries for the BME280 sensor and URL Encoder.
Installing URL Encode Library
To send messages through Whatabot API, the messages should be URLEncoded. URL encoding technique converts ASCII characters into a format that can be sent over the internet. For this purpose, we will use URLEncoder library available in Arduino IDE.
We will use the Library Manager in our Arduino IDE to install the latest versions of the libraries. Open your Arduino IDE and go to Sketch > Include Libraries > Manage Libraries. Type URLEncode in the search bar and install the latest version of the library by Masayuki Sugahara as shown below:
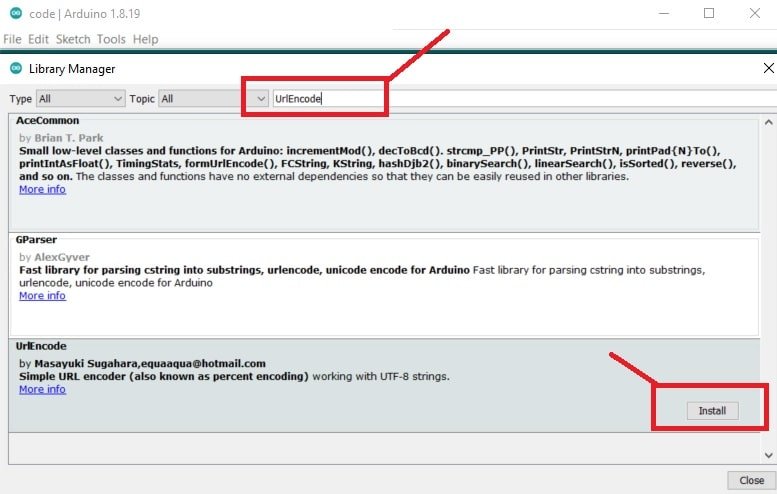
Installing BME280 Libraries
As we are connecting the BME280 sensor with ESP32 so we will have to install BME280 libraries to our module. We will require two libraries for this project:
- Adafruit_BME280 library
- Adafruit_Sensor library
We will use the Library Manager in our Arduino IDE to install the latest versions of the libraries. Open your Arduino IDE and go to Sketch > Include Libraries > Manage Libraries. Type each library name in the search bar and install them both.

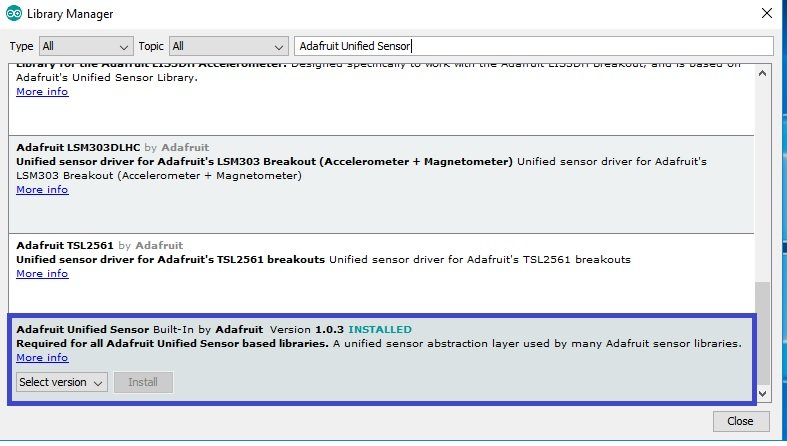
ESP32 Code – Sending BME280 Sensor Data as WhatsApp Messages
The following ESP32 code sends the current temperature, pressure and humidity readings acquired from the BME280 sensor to your WhatsApp number using Whatabot API and HTTPClient GET requests after every 5 seconds.
WhataBot API Code
#include <WiFi.h>
#include <HTTPClient.h>
#include <UrlEncode.h>
#include <Wire.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_BME280.h>
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASSWORD";
// add +international_country_code + phone number
// Pakistan +92, example: 913012222222
String mobile_number = "REPLACE_WITH_YOUR_PHONE_NUMBER";
String api_key = "REPLACE_WITH_API_KEY";
Adafruit_BME280 bme;
int Delay = 5000;
unsigned long last_time;
String getReadings(){
float temperature, humidity, pressure;
temperature = bme.readTemperature();
humidity = bme.readHumidity();
pressure = bme.readPressure() / 100.0F;
String readings = "Temperature: " + String(temperature) + " ºC \n";
readings += "Humidity: " + String (humidity) + " % \n";
readings += "Pressure: " + String (pressure) + " hPa \n";
return readings;
}
void send_whatsapp_message(String message){
String API_URL = "http://api.whatabot.net/whatsapp/sendMessage?text=" + urlEncode(message) + "&apikey=" + api_key + "&phone=" + mobile_number;
HTTPClient http;
http.begin(API_URL);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int http_response_code = http.GET();
if (http_response_code == 200){
Serial.println("Whatsapp message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(http_response_code);
}
http.end();
}
void setup() {
Serial.begin(115200);
bool status;
status = bme.begin(0x76);
if (!status) {
Serial.println("Could not detect a BME280 sensor, Fix wiring Connections!");
while (1);
}
delay(5000);
WiFi.begin(ssid, password);
Serial.println("Connecting to Wi-Fi Access Point");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.print("\nConnected to Wi-Fi Access Point network with IP Address: ");
Serial.println(WiFi.localIP());
}
void loop() {
if (millis() > last_time + Delay)
{
String readings = getReadings();
send_whatsapp_message(readings);
last_time = millis();
}
}
CallMeBot API Code
#include <WiFi.h>
#include <HTTPClient.h>
#include <UrlEncode.h>
#include <Wire.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_BME280.h>
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASSWORD";
// add +international_country_code + phone number
// Pakistan +92, example: 913012222222
String mobile_number = "REPLACE_WITH_YOUR_PHONE_NUMBER";
String api_key = "REPLACE_WITH_API_KEY";
Adafruit_BME280 bme;
int Delay = 5000;
unsigned long last_time;
String getReadings(){
float temperature, humidity, pressure;
temperature = bme.readTemperature();
humidity = bme.readHumidity();
pressure = bme.readPressure() / 100.0F;
String readings = "Temperature: " + String(temperature) + " ºC \n";
readings += "Humidity: " + String (humidity) + " % \n";
readings += "Pressure: " + String (pressure) + " hPa \n";
return readings;
}
void send_whatsapp_message(String message){
String API_URL = "https://api.callmebot.com/whatsapp.php?phone=" + mobile_number + "&api_key=" + api_key + "&text=" + urlEncode(message);
HTTPClient http;
http.begin(API_URL);
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
int http_response_code = http.POST(API_URL);
if (http_response_code == 200){
Serial.print("Whatsapp message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(http_response_code);
}
http.end();
}
void setup() {
Serial.begin(115200);
bool status;
status = bme.begin(0x76);
if (!status) {
Serial.println("Could not detect a BME280 sensor, Fix wiring Connections!");
while (1);
}
delay(5000);
WiFi.begin(ssid, password);
Serial.println("Connecting to Wi-Fi Access Point");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.print("\nConnected to Wi-Fi Access Point network with IP Address: ");
Serial.println(WiFi.localIP());
}
void loop() {
if (millis() > last_time + Delay)
{
String readings = getReadings();
send_whatsapp_message(readings);
last_time = millis();
}
}
How Code Works?
As mentioned earlier, sending messages with Whatabot API is simply equal to an HTTP GET request by using above given URL. Therefore, we need to include HTTP client and also URL encoded libraries header files.
Start by including header files of required libraries:
#include <WiFi.h>
#include <HTTPClient.h>
#include <UrlEncode.h>
#include <Wire.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_BME280.h>
Add your router/Wi-Fi credentials in the ssid and password variables respectively:
const char* ssid = "ADD_YOUR_SSID";
const char* password = "ADD_YOUR_PASSWORD";
Add your phone number and API key of Whatabot which you got from the last section. Please note that your phone number should be in an international format including country and mobile number.
// add +international_country_code + phone number
// Pakistan +92, example: 913012222222
String mobile_number = "REPLACE_WITH_YOUR_PHONE_NUMBER";
String api_key = "REPLACE_WITH_API_KEY";
Create an object of Adafruit_BME280 called ‘bme’ which we will use later on to initialize the sensor.
Adafruit_BME280 bme;
The Delay variable will monitor the time between new messages which is specified in milliseconds. We have specified 5000 milliseconds (5 seconds). As a result, after every 5 seconds the ESP32 board will acquire new sensor data from BME280 sensor and send it as a Whatsapp message.
int Delay = 5000;
unsigned long last_time;
getReadings()
Inside the getReadings() function, we will first define three variables of type float which will hold the sensor values. Then, we will use the bme object on readTemperature(), readHumidity() and readPressure() to access the readings individually. These sensor readings will be saved in the variables which we defined above: temperature, humidity and pressure.
This function will return a string variable ‘readings’ with all the three sensor readings which will be sent as a WhatsApp message.
String getReadings(){
float temperature, humidity, pressure;
temperature = bme.readTemperature();
humidity = bme.readHumidity();
pressure = bme.readPressure() / 100.0F;
String readings = "Temperature: " + String(temperature) + " ºC \n";
readings += "Humidity: " + String (humidity) + " % \n";
readings += "Pressure: " + String (pressure) + " hPa \n";
return readings;
}
send_whatsapp_message()
Create a function name send_whatsapp_message() which we will call whenever we want to send a WhatsApp message on our WhatsApp number. The input argument to this function is a message that we want to send.
void send_whatsapp_message(String message){
The next step is to modify the URL API according to our mobile number, apikey, and message which we want to send to our Whatsapp number. We created a string that holds API_URL containing your mobile number, apikey and URLEncoded message. The urlEncode() function takes a UTF-8 string and generates a percent-encoded string.
String API_URL = "http://api.whatabot.net/whatsapp/sendMessage?text=" + urlEncode(message) + "&apikey=" + api_key + "&phone=" + mobile_number;
Create an object of HTTPClient class which will initialize its constructor:
HTTPClient http;
Start HTTPClient by passing URL_API as a string to member function begin() of object http:
http.begin(API_URL);
We specify a content type in the header whenever we make an HTTP request to any server. This addHeader member function sets
the content-type header.
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
Finally, call a member function GET() of object http to send a get request to the Whatabot API server. If it returns http response 200 that means http get sent request was executed successfully and the Whatsapp message was sent successfully.
int http_response_code = http.GET();
if (http_response_code == 200){
Serial.print("Whatsapp message sent successfully");
}
else{
Serial.println("Error sending the message");
Serial.print("HTTP response code: ");
Serial.println(http_response_code);
}
At the end call, the end() member function frees the resources allocated to http request object.
http.end();
Setup() Function
Inside the setup() function initialize the serial communication with a baud rate of 115200 which will be used to show logs on the serial monitor of Arduino.
Serial.begin(115200);
Then we will initialize the BME280 sensor. If the connection between the module and the sensor is incorrect it will print that message on the serial monitor.
bool status;
status = bme.begin(0x76);
if (!status) {
Serial.println("Could not detect a BME280 sensor, Fix wiring Connections!");
while (1);
}
delay(5000);
To make an HTTTP request, ESP32 should have an internet connection. Hence, make a connection to your router or access point and get an assigned IP address. The following code tries to make a connection with your router and once connected, prints the assigned IP address on Arduino serial monitor.
WiFi.begin(ssid, password);
Serial.println("Connecting to Wi-Fi Access Point");
while(WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.print("\nConnected to Wi-Fi Access Point network with IP Address: ");
Serial.println(WiFi.localIP());
loop()
Inside the infinite loop() function, we call the getReadings() function and save the sensor readings in the string variable ‘readings.’ Then we call the send_whatsapp_message() function to send the WhatsApp message which is the sensor readings to our number. This is repeated after every 5 seconds.
void loop() {
if (millis() > last_time + Delay)
{
String readings = getReadings();
send_whatsapp_message(readings);
last_time = millis();
}
}
Demonstration
Now let’s see the demo of the above code. Insert the Wi-Fi credentials of your router into the above code and replace the API key. After that upload the code to ESP32.
After uploading code, open the serial monitor in Arduino IDE and select baud rate of 115200.
Now press the reset button on ESP32, you will see that ESP32 will connect with Wi-Fi network and print an IP address. Finally, it will print a message that the message was successfully sent. After every 5 seconds, you will receive the notification that the message was successfully sent.

Open your Whatsapp and you will see the messages received from the WhatsApp Bot.
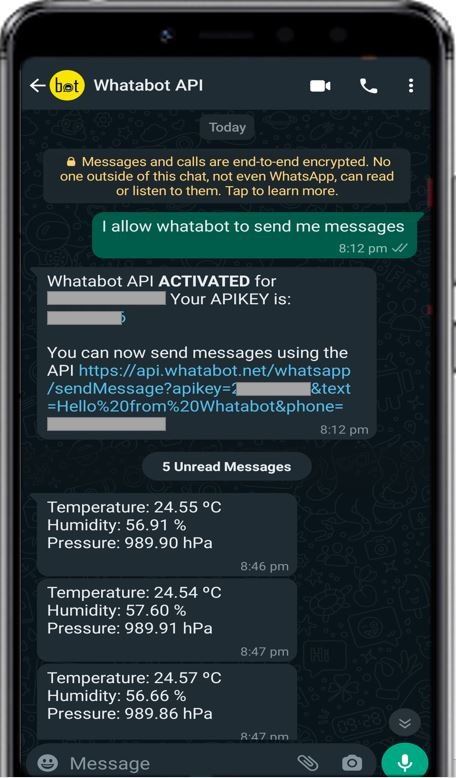
You may also like to read: