In this getting started tutorial, we will learn about the 28BYJ-48 stepper motor and how to interface it with the ESP32 development board using a UNL2003 driver. The 28BYJ-48 stepper motor is inexpensive and one of the most commonly used stepper motors out there. It comes with a UL2003 motor driver attached to it that is responsible for driving a stepper motor. The reason for using a driver is that the ESP32 GPIO pins can not provide enough driving current to a 28BYJ-48 stepper motor. The second important reason is that it protects ESP32 GPIO pins from getting damaged due to the high current requirement of a stepper motor than a maximum current that ESP32 GPIO pins can provide.
We have a similar guide with ESP8266 NodeMCU and Arduino:
- ESP8266 NodeMCU with Stepper Motor (28BYJ-48 and ULN2003 Motor Driver)
- Control 28BYJ-48 Stepper Motor with ULN2003 Motor Driver and Arduino
Prerequisites
We will program our ESP32 using the Arduino IDE. Make sure you are familiar with and have the latest version of Arduino IDE installed. Moreover, you will also require the ESP32 add-on installed in Arduino IDE:
We will require the following components for this user guide:
Required Components
- ESP32 development board
- One 28BYJ-48 Stepper Motor with ULN2003 motor driver
- External 5V power supply
- Connecting Wires
Stepper Motors Introduction
Stepper motors are DC brushless and synchronous motors. They rotate in discrete steps of predefined values and are able to rotate both clockwise and anticlockwise. Unlike other DC motors, they provide a precise position control according to the number of steps per revolution for which the motor is designed. That means a complete one revolution of a stepper motor is divided into a discrete number of steps. They are commonly used in CNC machines, Robotics, 2D and 3D printers.
For this guide, we will use a 28BYJ-48 stepper motor and control it through ULN2003 motor driver.
28BYJ-48 Stepper Motor
This is the most commonly used stepper motor in low power industrial and most famously in hobbyist projects.
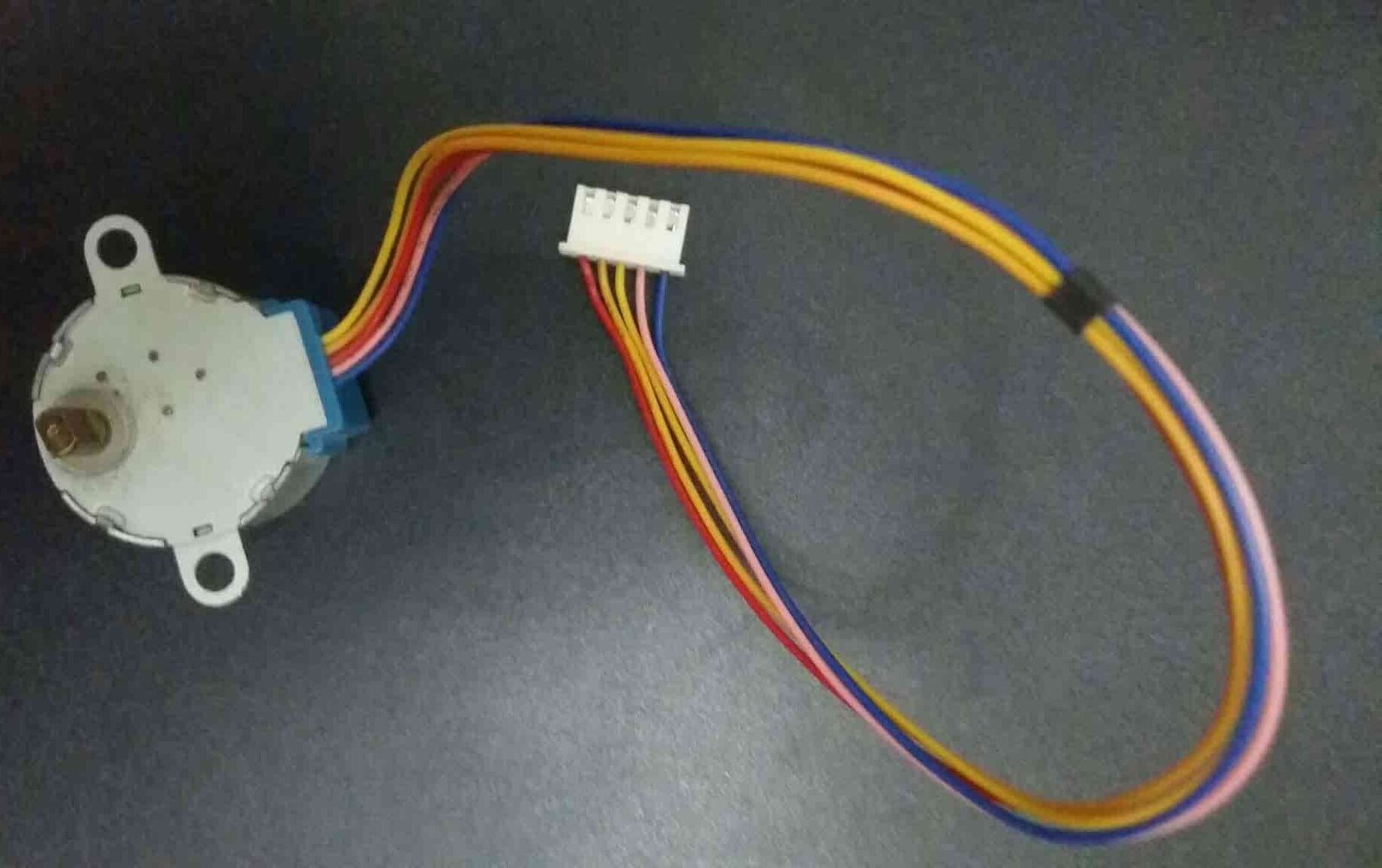
28BYJ-48 is a uni-polar 5V stepper motor that takes electrical signals as input and rotates by converting these input signals into mechanical rotation. It consists of 4 stationary coils rated at +5V. These coils are known as a stator and make a ring around the rotor. Because of 5 volts operating voltage, we can easily drive this motor from any microcontroller such as ESP32, ESP8266, Arduino or TM4C123 Tiva Launchpad, etc. It has a 1/64 reduction gear set and therefore moves in precise 512 steps per revolution. These motors are silent in comparison to other DC motors and servo motors. You can achieve positional control easily without needing extra circuitry and components.
Stride Angle
This stepper motor has a stride angle of 5.625 degrees. That means 28BYJ-48 will complete one revolution in (360/5.625) 64 steps by taking one step at a time and in one step it covers a 5.625-degree distance. However, the stepper motor can also be used in full-step mode. In full-step mode, the angle of each step is 11.25 degrees. That means the motor completes its one revolution in 32 steps instead( 360/11.25).
Therefore, in order to move one step forward or backward, the coils of the motor energize with a particular sequence.
Steps per Revolution & Step Angle
The output shaft of this particular stepper motor is driven through a gear ratio of 64:1 that is also known as the speed variation ratio. This suggests that after the inside motor rotates 64 times then the shaft will complete one rotation.
Therefore we can conclude that:
- In order to complete one full rotation of the shaft a total of 2048 steps will be required. This is known as the steps per revolution calculated by multiplying 32 and 64 (32×64=2048).
- Moreover, this will enable the motor to have a step angle of 360º/2048 steps = 0.18º/step.
Specifications
- It is a unipolar 5 pin coil with a rated DC voltage of 5V.
- Has 4 phases with a stride angle of 5.625°/64.
- Speed variation ratio is 1/64
- The frequency of this stepper motor is 100Hz and insulated power is 600VAC/1mA/1s.
- The half-step method is recommended for driving this stepper motor.
- The value of pull in torque for a stepper motor is 300 gf-cm.
Pinout
The following figure shows the pinout diagram of 28BYJ-48 stepper motor. It consists of 5 pins. Out of these 5 pins, four pins are used to provide sequence logic to the coils and one pin is a +5 volts supply pin.
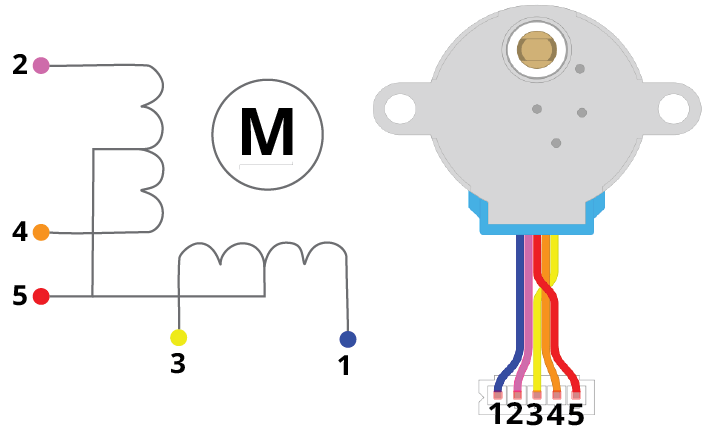
Pin Configuration Details
Pin Number | Coil Number | Colour |
1 | 4 | Blue |
2 | 2 | Pink |
3 | 3 | Yellow |
4 | 1 | Orange |
5 | Vcc | Red |
Coil 1-Coil 4: These are coils used to control the step sequence of the stepper motor. One end of each coil is connected with +5V and the other end will be connected with ULN2003 driver output.
Vcc: Used to apply +5 volt supply to the stepper motor. This voltage appears across the coils when a specific coil is ground through a control sequence.
ULN2003 Stepper Motor Driver Module
To use a 28BYJ-28 stepper motor with ESP32 board we will be required to attach it with the ULN2003 motor driver. This is necessary because the current consumption of 28BYJ-48 is around 240mA. That means the current required to drive coils by applying a sequence of control signals is also almost 200mA. GPIO pins of ESP development boards can not provide current of this magnitude. Therefore, we need a ULN2003 driver which translates low current output of ESP32 GPIO pins into higher current that meets the requirement of stepper motor control signals.
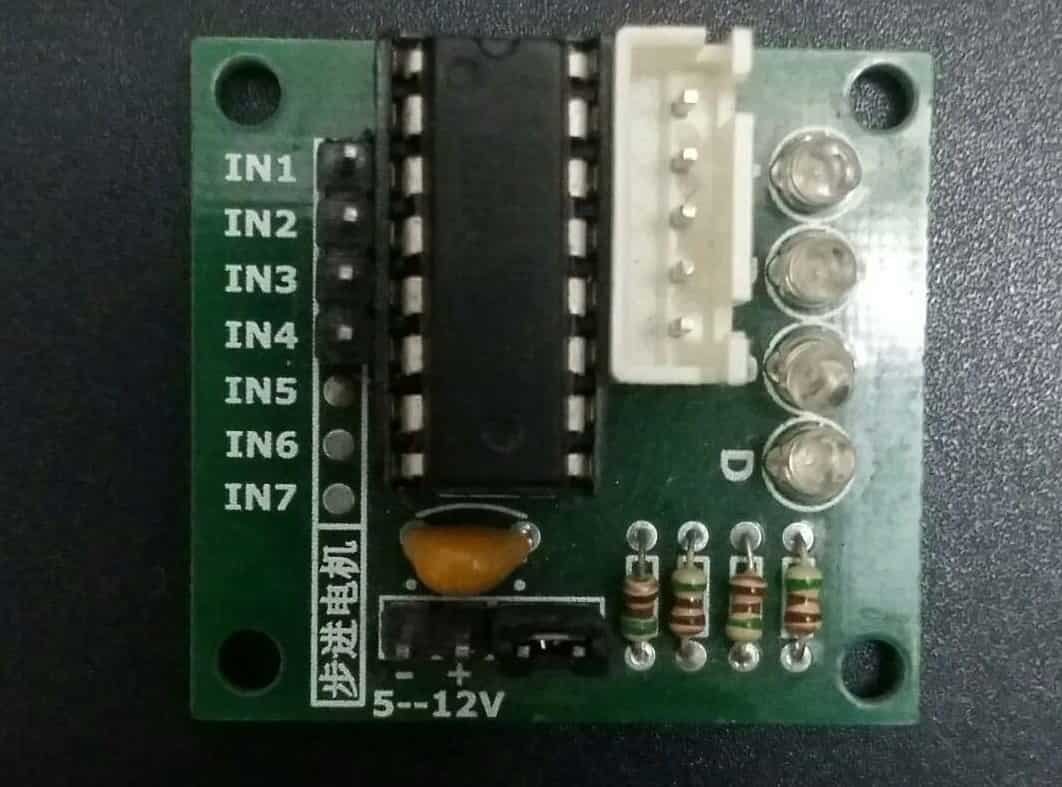
The ULN2003 breakout board has high current and voltage than a single transistor and therefore it can drive a stepper motor easily by enabling our ESP board.
Recommneded reading: ULN2003 introduction, pinout, example and features
Stepper Motor Driver Module Pinout
ULN2003 driver IC consists of 7 Darlington pair transistor outputs. Each output can drive 500mA and 50V load. The input to each 7 Darlington pair transistor will be a signal from our microcontroller. To drive a stepper motor, this driver board uses only four input pins (IN1, IN2, IN3, and IN4).
The following diagram shows the ULN2003 motor driver board:
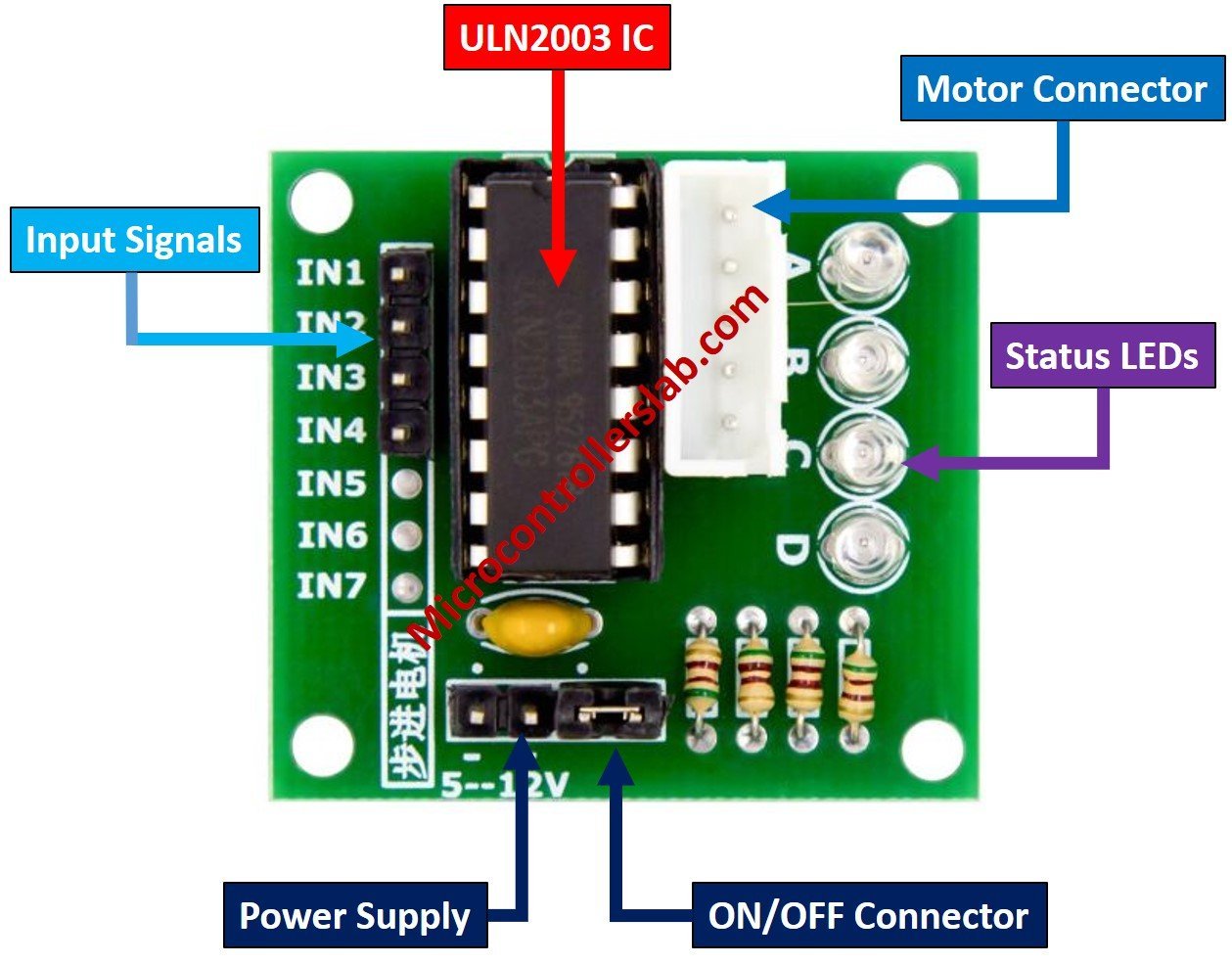
Motor Connector Header is used to connect the stepper motor. It provides output from four Darlington pair transistors.
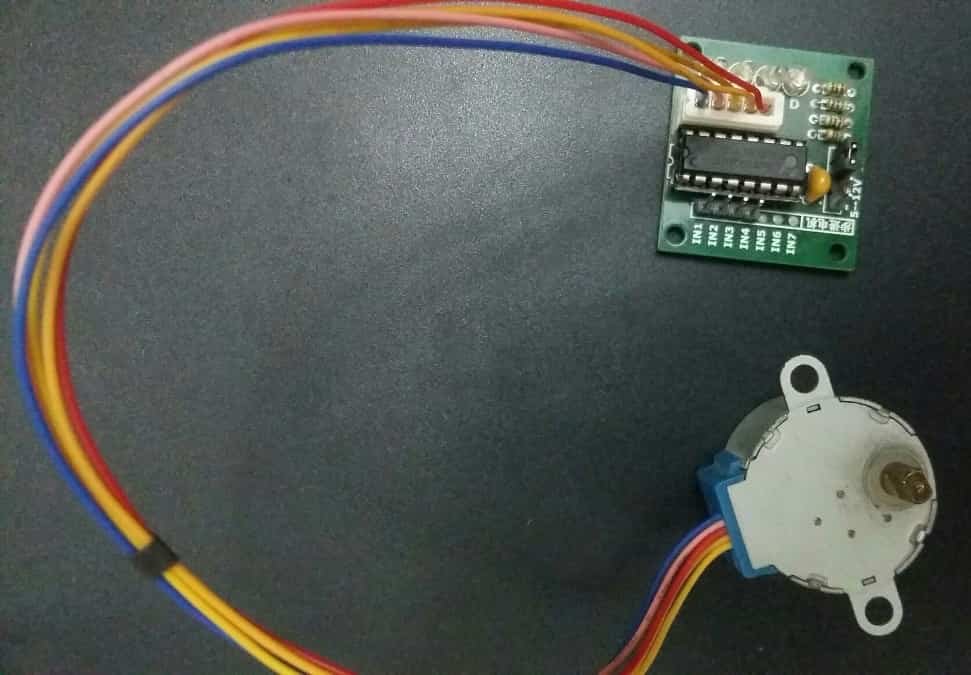
Pin | Description |
1N1 to IN4 | These are input pins used to provide control signals to the stepper motor such as control sequences. We will connect these pins with the GPIO pins of ESP32. |
Vcc and GND | Vcc is a power supply pin and it is used to provide 5 volts power to the stepper motor from an external power source. |
ESP32 does not have an onboard 5 volts signal. Therefore, we will need to provide an external power supply of 5V to the motor driver in order for it to work.
Additionally, the 28BYJ-48 stepper motor requires 240mA current to operate and it also consumes power at an idle condition. Therefore, it is recommended not to power the 28BYJ-48 stepper motor directly from any microcontroller instead use an external 5 volts power supply.
Interfacing ESP32 with 28BYJ-48 Stepper Motor and ULN2003 motor driver
To connect the ESP32 development board with the stepper motor and driver we will use the input pins IN1-IN4, the power supply pins, and the motor connection header. The 28BYJ-48 stepper motor already comes attached with the motor driver via the motor connector header. Now we will connect four GPIO pins of our ESP32 board with the input pins (IN1-IN4) of the driver. We have used the following GPIO pins to connect with each input pin.
ESP32 | Motor Driver |
---|---|
GPIO26 | IN1 |
GPIO25 | IN2 |
GPIO33 | IN3 |
GPIO32 | IN4 |
You can use any suitable ESP32 GPIO pins as well.
Additionally, we will power the motor driver with a 5V external power supply. Both the grounds of the power supply and the ESP32 board will be in common.
The connection diagram is shown in the picture below.
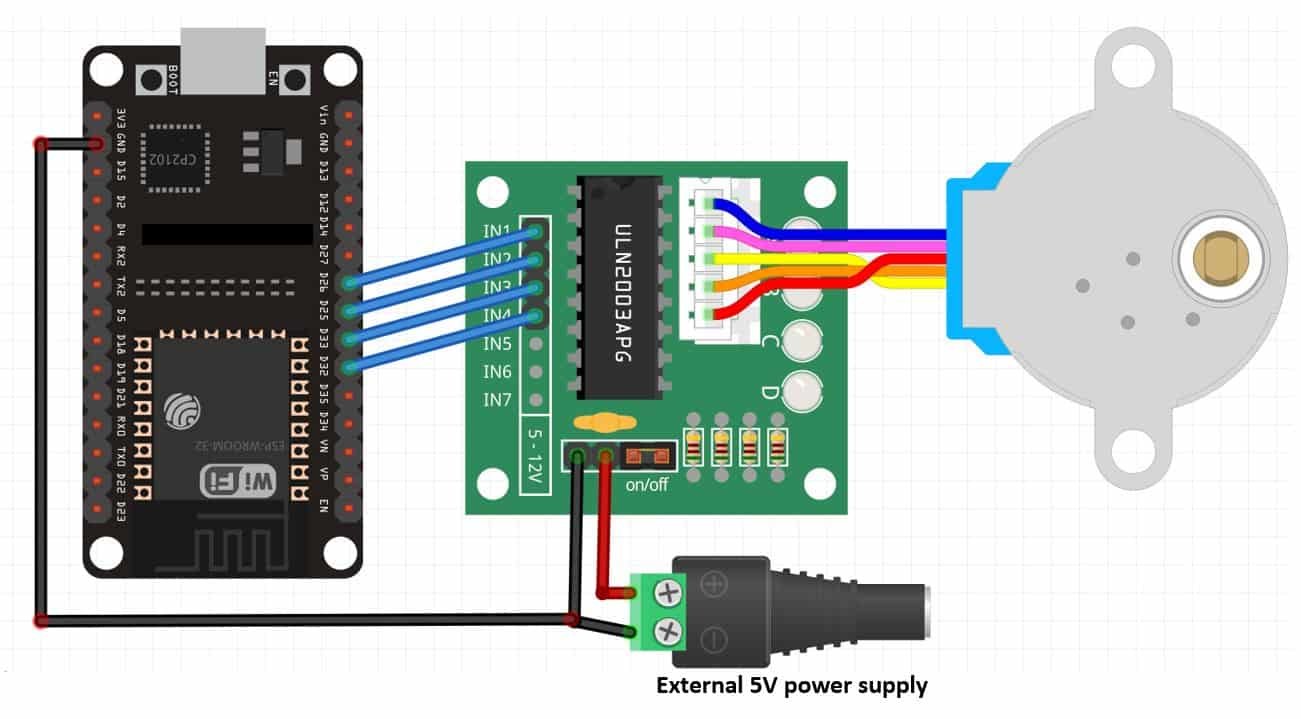
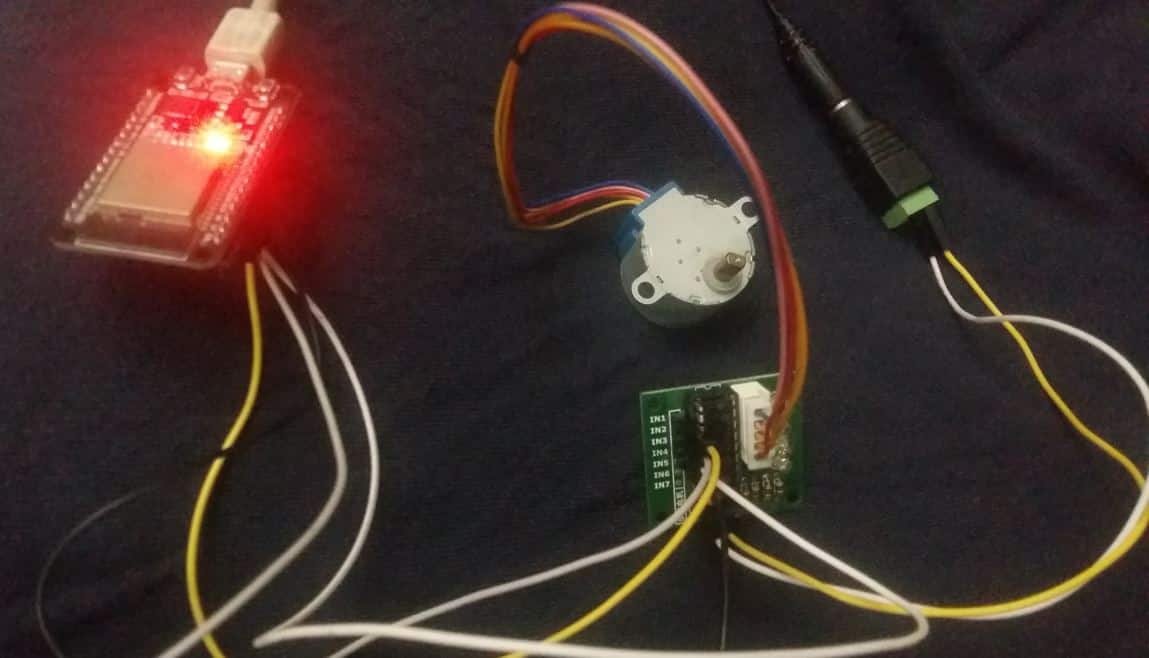
ESP32 Arduino Sketch Controlling 28BYJ-48 Stepper Motor
Open your Arduino IDE and go to File > New. A new file will open. Copy the code given below in that file and save it.
This code will help us control the stepper motor by setting the speed and steps per revolution. We will show you how to rotate the motor in both directions.
#include <Stepper.h>
const int steps_per_rev = 2048;
#define IN1 26
#define IN2 25
#define IN3 33
#define IN4 32
Stepper motor(steps_per_rev, IN1, IN3, IN2, IN4);
void setup() {
motor.setSpeed(10);
Serial.begin(115200);
}
void loop() {
Serial.println("Rotating Clockwise...");
motor.step(steps_per_rev);
delay(1000);
Serial.println("Rotating Anti-clockwise...");
motor.step(-steps_per_rev);
delay(1000);
}
How the Code Works?
Firstly, we will include the Stepper.h library. This library provides useful functions that make it easy to control the stepper motor.
#include <Stepper.h>
The next step is to define the steps per revolution. This is the number of steps our motor requires to move one complete revolution. Previously, we showed the calculations for obtaining this value.
const int steps_per_rev = 2048;
Next, we will define the input pins of the motor connections with the ESP32 board. As you can see we have used the GPIO pins 26,25,33 and 32 to connect with IN1, IN2, IN3 and IN4 respectively. However, you can use any other suitable ESP32 GPIO pins as well.
#define IN1 26
#define IN2 25
#define IN3 33
#define IN4 32
Now we will create an instance of the Stepper library called motor() and pass the steps per revolution and the individual motor input pins as arguments. Make sure you specify the input pins in their correct sequence. It is IN1, IN3, IN2, and IN4 for 28BYJ-48 stepper motor.
Stepper motor(steps_per_rev, IN1, IN3, IN2, IN4);
Inside the setup() function, Serial.begin() is used to establish the serial connection between the development board at a baud rate of 115200. We will use the setSpeed() method on the motor instance and pass the speed of the motor in rpm as an argument inside it. In our case we are setting the stepper motor speed to 10 revolutions per minute.
void setup() {
motor.setSpeed(10);
Serial.begin(115200);
}
Inside the loop() function, we will first rotate the motor clockwise by using the step() method and passing the steps per revolution as the argument inside it. Hence the motor will rotate at steps of 2048 per revolution. Likewise, to rotate the motor anti-clockwise we will pass the steps per revolution with a negative sign inside the step() method. Between the two types of rotations we will have a delay of 1 second. Additionally, we will print the type of rotation in the serial monitor as well.
void loop() {
Serial.println("Rotating Clockwise...");
motor.step(steps_per_rev);
delay(1000);
Serial.println("Rotating Anti-clockwise...");
motor.step(-steps_per_rev);
delay(1000);
}
Demonstration
Make sure you choose the correct board and COM port before uploading your code to the board. Go to Tools > Board and select ESP32 Dev Module.
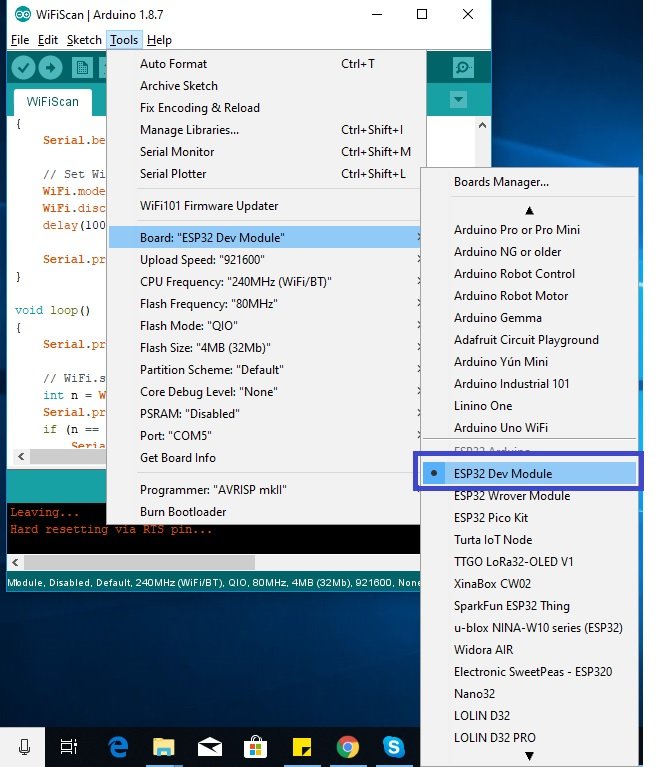
Next, go to Tools > Port and select the appropriate port through which your board is connected.
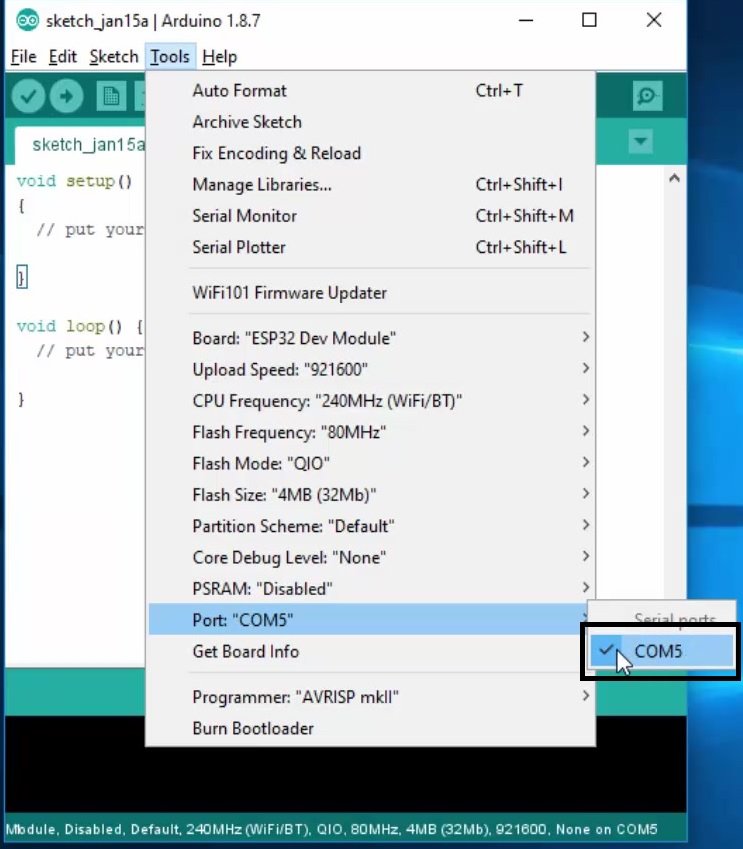
Click on the upload button to upload the code into the ESP32 development board.
After you have uploaded your code to the development board, press its ENABLE button.
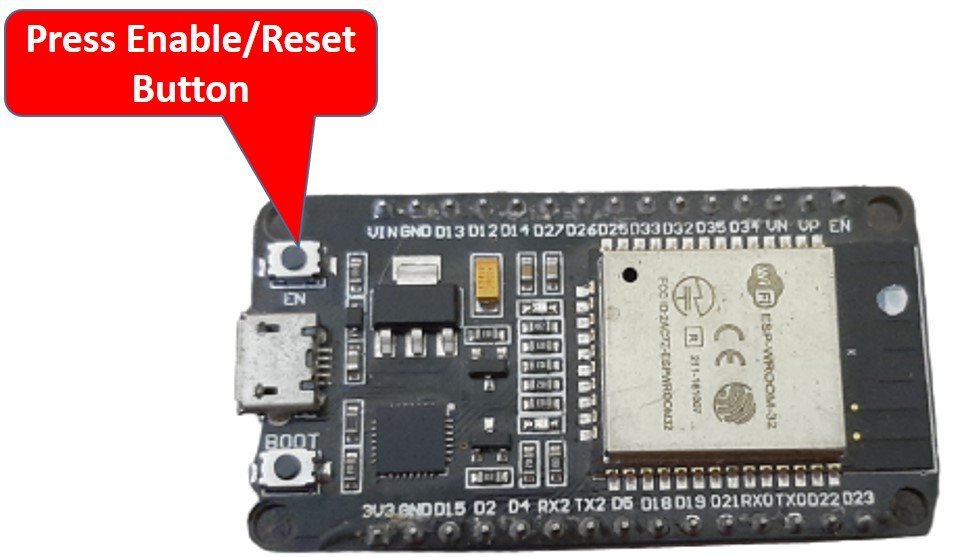
The stepper motor will start rotating clockwise and then anti-clockwise repeatedly.
In your Arduino IDE, open up the serial monitor and you will be able to see the status of the motor rotation as well.

Below you can view the demonstration of the stepper motor as well.
Conclusion
In conclusion, in this introductory guide to 28BYJ-48 stepper motor with ULN2003 motor driver, we have learned about the stepper motor and its driver ULN2007. We saw its introduction, specifications pinout, and interfacing with the ESP32 development board. Then we controlled the stepper motor by programming our ESP32 board in Arduino IDE using the Stepper.h library. This included rotating it both clockwise and in an anti-clockwise direction. In the next tutorial, we will show you how to create a WebSocket web server to control the stepper motor.
Related tutorials and projects:
- Stepper Motor Interfacing with TM4C123 Tiva Launchpad
- Stepper Motor Control with L293D Motor Driver IC and Arduino
- 28BYJ-48 5 volt Stepper Motor Guide
- NEMA 23 Stepper Motor Pinout, features and example with Arduino
- STEPPER MOTOR INTERFACING WITH 8051 MICROCONTROLLER
- TMC2209 Stepper Motor Driver module – SilentStepStick
- NEMA 34 Stepper Motor
- STEPPER MOTOR INTERFACING WITH PIC16F877A MICROCONTROLLER
Great tutorial bro
I’ve find out stepper motor control tutorial, and all i have is zonk, my stepper can’t turn in counter clockwise direction
but when i found this tutor, its work well with my stepper
it can turn clockwise and counter clockwise
Thank You,
Have a good day