This tutorial teaches you how to interface an OLED display with TM4C123 Tiva Launchpad. In this article, we will show how to use SSD1306 0.96 inch I2C OLED with TM4C123 Tiva Launchpad. This modern organic light emitting diode based display can be used to write simple text, scrolling text, display bitmap images, draw different shapes, digital and analog clock. Firstly, we will see how to connect it with TM4C123. In the end, we will see programming examples of TM4C123 with SSD1306 0.96 inch I2C OLED using Keil uvision for ARM.

OLED Interfacing diagram with TM4C123
Do you want to give a perfect look to your microcontroller project which traditional liquid crystal displays (LCDs) do not promise? In this case, OLED displays will be the best choice for you. Because they offer a good view angle and pixel density which makes it the perfect choice for graphics display projects at low cost.
SSD1306 OLED Types
There are different types of OLED displays available in the market. But the common thing in most displays is the SSD1306 CMOS OLED driver controller. The main component of OLED is an SSD1306 controller which is used to communicate with microcontrollers, such as TM4C123 Tiva Launchpad, using either SPI or I2C communication. But usually, I2C communication is preferred because it requires only two wires to communicate with TM4C123.
I2C OLED Display
These displays come in different colors, sizes and shapes. In this tutorial, we will use an I2C based OLED display having size of 128×64 as shown in the figure below. But the good thing is programming doesn’t change much with the change of OLED size and color.
The following picture shows the pin wiring of the OLED display. As you can see from its pinout diagram, it consists of four pins such as Vcc, GND, SCL, and SDA. Vcc and GND pins are used to power OLED displays and the operating voltage range is between 3.3-5V. That means we can easily power it from the same source and connect directly with microcontrollers
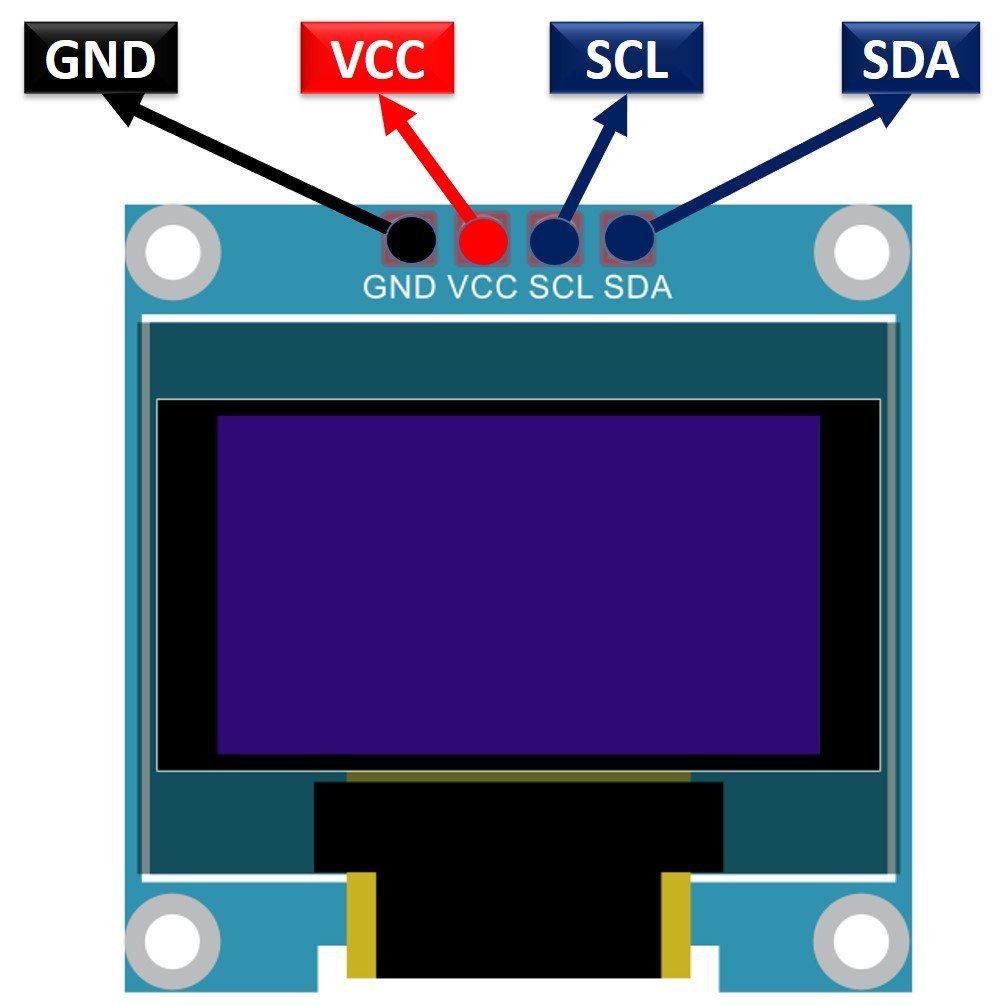
SCL and SDA are the serial clock and serial data pins respectively. They connect with I2C pins of microcontrollers (TM4C123) to perform I2C communication.
SSD1306 Connections with TM4C123G Tiva
Before diving into the interfacing diagram of OLED with TM4C123G Tiva Launchpad, we need to understand the power supply and current consumption requirement of OLED. By knowing about voltage range and current requirement, we will determine that either we can drive this display directly with TM4C123G Tiva Launchpad or we need to connect any interfacing circuitry?
According to the datasheet of SDS1306 OLED display, the operating voltage range is between 3.3-5V and maximum current requirement is 20mA. That means we can directly interface the OLED display with TM4C123G Tiva Launchpad. Because the TM4C123 development board has onboard 3.3V power source signal and GPIO pins can sink and source upto 20mA current. Hence, we can directly interface OLED with TM4C123G.
Interfacing Diagram
To interface an OLED display with TM4C123G Tiva Launchpad, we use four pins only such as Vcc, GND, and I2C communication pins such as SCL and SDA. TM4C123GH6PM microcontroller which comes with Tiva Launchpad has four built-in I2C modules such as I2C0, I2C1, I2C2, and I2C3. The following table shows the GPIO pins of TM4C123GH6PM associated with these I2C modules.
I2C Module | SCL Pin | SDA Pin |
---|---|---|
I2C0 | PB2 | PB3 |
I2C1 | PA6 | PA7 |
I2C2 | PE4 | PE5 |
I2C3 | PD0 | PD1 |
In this tutorial, we will use the I2C3 module of TM4C123 to communicate with OLED. For I2C3 port, PD0 and PD1 (GPIOD) are SDA and SCL pins respectively. Now make connection with TM4C123 and OLED display according to the diagram shown below:
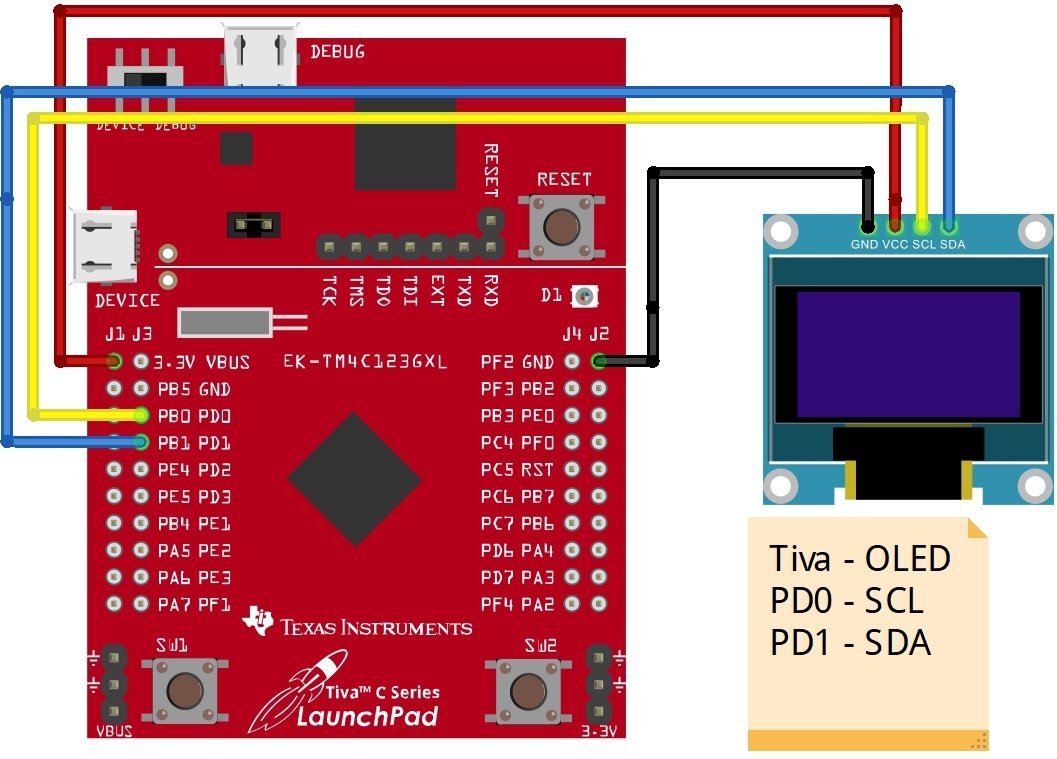
TM4C123 | SSD1306 OLED |
---|---|
PD0/SCL3/I2C3 | SCL |
PD1/SDA3/I2C3 | SDA |
3.3V | Vcc |
GND | GND |
OLED Programming with TM4C123 and Keil uvision
To write programming of OLED displays with TM4C123, we will use an I2C3 port of TM4C123GH6PM microcontroller. Because, the SDS1306 controller communicates with microcontrollers through I2C communication.
In our previously published article on I2C communication between TM4C123 and Arduino, we developed a library for I2C3 port of TM4C123GH6PM. These three functions are developed:
// Initialize the I2C3 module of TM4C123GM6PM Microcontroller
void I2C3_Init ( void )
// Wait until I2C buffer is not free
static int I2C_wait_till_done(void)
// Write single byte to a selected slave address and memory address of slave
char I2C3_Wr(int slaveAddr, char memAddr, uint8_t data);
// Write Multiple bytes to a selected slave address and memory address of slave
char I2C3_Write_Multiple(int slave_address, char slave_memory_address, int bytes_count, char* data);
For further information on these functions, check this in-depth tutorial:
Hence, we will use these I2C3 functions to send commands and data to an OLED display.
Code
This code displays text on the SSD1306 OLED display. Firstly it prints some strings and after that displays the counter value from 0-100. But you can modify it according to your requirement.
This code is written in Keil uvision. You can simply copy this code and create a new project in keil uvision. If you are a beginner with TM4C123 and did not use Keil IDE before, you can read these getting started guides:
#include "TM4C123GH6PM.h"
#include <stdint.h>
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
#include "math.h"
const uint8_t OledFont[][8] =
{
{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00},
{0x00,0x00,0x5F,0x00,0x00,0x00,0x00,0x00},
{0x00,0x00,0x07,0x00,0x07,0x00,0x00,0x00},
{0x00,0x14,0x7F,0x14,0x7F,0x14,0x00,0x00},
{0x00,0x24,0x2A,0x7F,0x2A,0x12,0x00,0x00},
{0x00,0x23,0x13,0x08,0x64,0x62,0x00,0x00},
{0x00,0x36,0x49,0x55,0x22,0x50,0x00,0x00},
{0x00,0x00,0x05,0x03,0x00,0x00,0x00,0x00},
{0x00,0x1C,0x22,0x41,0x00,0x00,0x00,0x00},
{0x00,0x41,0x22,0x1C,0x00,0x00,0x00,0x00},
{0x00,0x08,0x2A,0x1C,0x2A,0x08,0x00,0x00},
{0x00,0x08,0x08,0x3E,0x08,0x08,0x00,0x00},
{0x00,0xA0,0x60,0x00,0x00,0x00,0x00,0x00},
{0x00,0x08,0x08,0x08,0x08,0x08,0x00,0x00},
{0x00,0x60,0x60,0x00,0x00,0x00,0x00,0x00},
{0x00,0x20,0x10,0x08,0x04,0x02,0x00,0x00},
{0x00,0x3E,0x51,0x49,0x45,0x3E,0x00,0x00},
{0x00,0x00,0x42,0x7F,0x40,0x00,0x00,0x00},
{0x00,0x62,0x51,0x49,0x49,0x46,0x00,0x00},
{0x00,0x22,0x41,0x49,0x49,0x36,0x00,0x00},
{0x00,0x18,0x14,0x12,0x7F,0x10,0x00,0x00},
{0x00,0x27,0x45,0x45,0x45,0x39,0x00,0x00},
{0x00,0x3C,0x4A,0x49,0x49,0x30,0x00,0x00},
{0x00,0x01,0x71,0x09,0x05,0x03,0x00,0x00},
{0x00,0x36,0x49,0x49,0x49,0x36,0x00,0x00},
{0x00,0x06,0x49,0x49,0x29,0x1E,0x00,0x00},
{0x00,0x00,0x36,0x36,0x00,0x00,0x00,0x00},
{0x00,0x00,0xAC,0x6C,0x00,0x00,0x00,0x00},
{0x00,0x08,0x14,0x22,0x41,0x00,0x00,0x00},
{0x00,0x14,0x14,0x14,0x14,0x14,0x00,0x00},
{0x00,0x41,0x22,0x14,0x08,0x00,0x00,0x00},
{0x00,0x02,0x01,0x51,0x09,0x06,0x00,0x00},
{0x00,0x32,0x49,0x79,0x41,0x3E,0x00,0x00},
{0x00,0x7E,0x09,0x09,0x09,0x7E,0x00,0x00},
{0x00,0x7F,0x49,0x49,0x49,0x36,0x00,0x00},
{0x00,0x3E,0x41,0x41,0x41,0x22,0x00,0x00},
{0x00,0x7F,0x41,0x41,0x22,0x1C,0x00,0x00},
{0x00,0x7F,0x49,0x49,0x49,0x41,0x00,0x00},
{0x00,0x7F,0x09,0x09,0x09,0x01,0x00,0x00},
{0x00,0x3E,0x41,0x41,0x51,0x72,0x00,0x00},
{0x00,0x7F,0x08,0x08,0x08,0x7F,0x00,0x00},
{0x00,0x41,0x7F,0x41,0x00,0x00,0x00,0x00},
{0x00,0x20,0x40,0x41,0x3F,0x01,0x00,0x00},
{0x00,0x7F,0x08,0x14,0x22,0x41,0x00,0x00},
{0x00,0x7F,0x40,0x40,0x40,0x40,0x00,0x00},
{0x00,0x7F,0x02,0x0C,0x02,0x7F,0x00,0x00},
{0x00,0x7F,0x04,0x08,0x10,0x7F,0x00,0x00},
{0x00,0x3E,0x41,0x41,0x41,0x3E,0x00,0x00},
{0x00,0x7F,0x09,0x09,0x09,0x06,0x00,0x00},
{0x00,0x3E,0x41,0x51,0x21,0x5E,0x00,0x00},
{0x00,0x7F,0x09,0x19,0x29,0x46,0x00,0x00},
{0x00,0x26,0x49,0x49,0x49,0x32,0x00,0x00},
{0x00,0x01,0x01,0x7F,0x01,0x01,0x00,0x00},
{0x00,0x3F,0x40,0x40,0x40,0x3F,0x00,0x00},
{0x00,0x1F,0x20,0x40,0x20,0x1F,0x00,0x00},
{0x00,0x3F,0x40,0x38,0x40,0x3F,0x00,0x00},
{0x00,0x63,0x14,0x08,0x14,0x63,0x00,0x00},
{0x00,0x03,0x04,0x78,0x04,0x03,0x00,0x00},
{0x00,0x61,0x51,0x49,0x45,0x43,0x00,0x00},
{0x00,0x7F,0x41,0x41,0x00,0x00,0x00,0x00},
{0x00,0x02,0x04,0x08,0x10,0x20,0x00,0x00},
{0x00,0x41,0x41,0x7F,0x00,0x00,0x00,0x00},
{0x00,0x04,0x02,0x01,0x02,0x04,0x00,0x00},
{0x00,0x80,0x80,0x80,0x80,0x80,0x00,0x00},
{0x00,0x01,0x02,0x04,0x00,0x00,0x00,0x00},
{0x00,0x20,0x54,0x54,0x54,0x78,0x00,0x00},
{0x00,0x7F,0x48,0x44,0x44,0x38,0x00,0x00},
{0x00,0x38,0x44,0x44,0x28,0x00,0x00,0x00},
{0x00,0x38,0x44,0x44,0x48,0x7F,0x00,0x00},
{0x00,0x38,0x54,0x54,0x54,0x18,0x00,0x00},
{0x00,0x08,0x7E,0x09,0x02,0x00,0x00,0x00},
{0x00,0x18,0xA4,0xA4,0xA4,0x7C,0x00,0x00},
{0x00,0x7F,0x08,0x04,0x04,0x78,0x00,0x00},
{0x00,0x00,0x7D,0x00,0x00,0x00,0x00,0x00},
{0x00,0x80,0x84,0x7D,0x00,0x00,0x00,0x00},
{0x00,0x7F,0x10,0x28,0x44,0x00,0x00,0x00},
{0x00,0x41,0x7F,0x40,0x00,0x00,0x00,0x00},
{0x00,0x7C,0x04,0x18,0x04,0x78,0x00,0x00},
{0x00,0x7C,0x08,0x04,0x7C,0x00,0x00,0x00},
{0x00,0x38,0x44,0x44,0x38,0x00,0x00,0x00},
{0x00,0xFC,0x24,0x24,0x18,0x00,0x00,0x00},
{0x00,0x18,0x24,0x24,0xFC,0x00,0x00,0x00},
{0x00,0x00,0x7C,0x08,0x04,0x00,0x00,0x00},
{0x00,0x48,0x54,0x54,0x24,0x00,0x00,0x00},
{0x00,0x04,0x7F,0x44,0x00,0x00,0x00,0x00},
{0x00,0x3C,0x40,0x40,0x7C,0x00,0x00,0x00},
{0x00,0x1C,0x20,0x40,0x20,0x1C,0x00,0x00},
{0x00,0x3C,0x40,0x30,0x40,0x3C,0x00,0x00},
{0x00,0x44,0x28,0x10,0x28,0x44,0x00,0x00},
{0x00,0x1C,0xA0,0xA0,0x7C,0x00,0x00,0x00},
{0x00,0x44,0x64,0x54,0x4C,0x44,0x00,0x00},
{0x00,0x08,0x36,0x41,0x00,0x00,0x00,0x00},
{0x00,0x00,0x7F,0x00,0x00,0x00,0x00,0x00},
{0x00,0x41,0x36,0x08,0x00,0x00,0x00,0x00},
{0x00,0x02,0x01,0x01,0x02,0x01,0x00,0x00},
{0x00,0x02,0x05,0x05,0x02,0x00,0x00,0x00},
};
// Define OLED dimensions
#define OLED_WIDTH 128
#define OLED_HEIGHT 64
#define slaveaddress 0x3C
// Define command macros
#define OLED_SETCONTRAST 0x81
#define OLED_DISPLAYALLON_RESUME 0xA4
#define OLED_DISPLAYALLON 0xA5
#define OLED_NORMALDISPLAY 0xA6
#define OLED_INVERTDISPLAY 0xA7
#define OLED_DISPLAYOFF 0xAE
#define OLED_DISPLAYON 0xAF
#define OLED_SETDISPLAYOFFSET 0xD3
#define OLED_SETCOMPINS 0xDA
#define OLED_SETVCOMDETECT 0xDB
#define OLED_SETDISPLAYCLOCKDIV 0xD5
#define OLED_SETPRECHARGE 0xD9
#define OLED_SETMULTIPLEX 0xA8
#define OLED_SETLOWCOLUMN 0x00
#define OLED_SETHIGHCOLUMN 0x10
#define OLED_SETSTARTLINE 0x40
#define OLED_MEMORYMODE 0x20
#define OLED_COLUMNADDR 0x21
#define OLED_PAGEADDR 0x22
#define OLED_COMSCANINC 0xC0
#define OLED_COMSCANDEC 0xC8
#define OLED_SEGREMAP 0xA0
#define OLED_CHARGEPUMP 0x8D
// Function declarations
void OLED_Command( uint8_t temp);
void OLED_Data( uint8_t temp);
void OLED_Init();
void OLED_YX(unsigned char Row, unsigned char Column); // *warning!* max 4 rows
void OLED_PutChar( char ch );
void OLED_Clear();
void OLED_Write_String( char *s );
void OLED_Write_Integer(uint8_t i);
void OLED_Write_Float(float f);
void Delay_ms(int time_ms);
// Function prototypes initialize, tranmit and rea functions
void I2C3_Init ( void );
static int I2C_wait_till_done(void);
char I2C3_Write_Multiple(int slave_address, char slave_memory_address, int bytes_count, uint8_t* data);
char I2C3_Wr(int slaveAddr, char memAddr, uint8_t data);
int main(void)
{
I2C3_Init();
OLED_Init();
OLED_Clear();
// variables for counting
int count = 0;
float dec = 0.0;
while ( 1 ) {
/////////////////////
// Strings
///////////////////
OLED_YX( 0, 0 );
OLED_Write_String( "OLED SSD1306" );
Delay_ms(1000);
OLED_YX(1, 0);
OLED_Write_String ("TM4C123");
Delay_ms(1000);
/////////////////////
// Integer Count
////////////////////
for (count = 0; count <= 100; count++){
OLED_YX(2, 0 );
OLED_Write_String( "Count:" );
OLED_YX(2, 8);
OLED_Write_Integer(count);
Delay_ms(100);
}
OLED_Clear();
Delay_ms(100);
}
}
// I2C intialization and GPIO alternate function configuration
void I2C3_Init ( void )
{
SYSCTL->RCGCGPIO |= 0x00000008 ; // Enable the clock for port D
SYSCTL->RCGCI2C |= 0x00000008 ; // Enable the clock for I2C 3
GPIOD->DEN |= 0x03; // Assert DEN for port D
// Configure Port D pins 0 and 1 as I2C 3
GPIOD->AFSEL |= 0x00000003 ;
GPIOD->PCTL |= 0x00000033 ;
GPIOD->ODR |= 0x00000002 ; // SDA (PD1 ) pin as open darin
I2C3->MCR = 0x0010 ; // Enable I2C 3 master function
/* Configure I2C 3 clock frequency
(1 + TIME_PERIOD ) = SYS_CLK /(2*
( SCL_LP + SCL_HP ) * I2C_CLK_Freq )
TIME_PERIOD = 16 ,000 ,000/(2(6+4) *100000) - 1 = 7 */
I2C3->MTPR = 0x07 ;
}
/* wait untill I2C Master module is busy */
/* and if not busy and no error return 0 */
static int I2C_wait_till_done(void)
{
while(I2C3->MCS & 1); /* wait until I2C master is not busy */
return I2C3->MCS & 0xE; /* return I2C error code, 0 if no error*/
}
char I2C3_Wr(int slaveAddr, char memAddr, uint8_t data)
{
char error;
/* send slave address and starting address */
I2C3->MSA = slaveAddr << 1;
I2C3->MDR = memAddr;
I2C3->MCS = 3; /* S-(saddr+w)-ACK-maddr-ACK */
error = I2C_wait_till_done(); /* wait until write is complete */
if (error) return error;
/* send data */
I2C3->MDR = data;
I2C3->MCS = 5; /* -data-ACK-P */
error = I2C_wait_till_done(); /* wait until write is complete */
while(I2C3->MCS & 0x40); /* wait until bus is not busy */
error = I2C3->MCS & 0xE;
if (error) return error;
return 0; /* no error */
}
// Receive one byte of data from I2C slave device
char I2C3_Write_Multiple(int slave_address, char slave_memory_address, int bytes_count, uint8_t* data)
{
char error;
if (bytes_count <= 0)
return -1; /* no write was performed */
/* send slave address and starting address */
I2C3->MSA = slave_address << 1;
I2C3->MDR = slave_memory_address;
I2C3->MCS = 3; /* S-(saddr+w)-ACK-maddr-ACK */
error = I2C_wait_till_done(); /* wait until write is complete */
if (error) return error;
/* send data one byte at a time */
while (bytes_count > 1)
{
I2C3->MDR = *data++; /* write the next byte */
I2C3->MCS = 1; /* -data-ACK- */
error = I2C_wait_till_done();
if (error) return error;
bytes_count--;
}
/* send last byte and a STOP */
I2C3->MDR = *data++; /* write the last byte */
I2C3->MCS = 5; /* -data-ACK-P */
error = I2C_wait_till_done();
while(I2C3->MCS & 0x40); /* wait until bus is not busy */
if (error) return error;
return 0; /* no error */
}
//OLED
void OLED_Command( uint8_t temp){
I2C3_Wr(0x3C,0x00,temp);
}
/*******************************************************************************
* Function: void OLED_Data ( uint8_t temp)
*
* Returns: Nothing
*
* Description: sends data to the OLED
*
******************************************************************************/
void OLED_Data( uint8_t temp){
I2C3_Wr(0x3C,0x40,temp);
}
/*******************************************************************************
* Function: void OLED_Init ()
*
* Returns: Nothing
*
* Description: Initializes OLED
*
******************************************************************************/
void OLED_Init() {
OLED_Command(OLED_DISPLAYOFF); // 0xAE
OLED_Command(OLED_SETDISPLAYCLOCKDIV); // 0xD5
OLED_Command(0x80); // the suggested ratio 0x80
OLED_Command(OLED_SETMULTIPLEX); // 0xA8
OLED_Command(0x1F);
OLED_Command(OLED_SETDISPLAYOFFSET); // 0xD3
OLED_Command(0x0); // no offset
OLED_Command(OLED_SETSTARTLINE | 0x0); // line #0
OLED_Command(OLED_CHARGEPUMP); // 0x8D
OLED_Command(0xAF);
OLED_Command(OLED_MEMORYMODE); // 0x20
OLED_Command(0x00); // 0x0 act like ks0108
OLED_Command(OLED_SEGREMAP | 0x1);
OLED_Command(OLED_COMSCANDEC);
OLED_Command(OLED_SETCOMPINS); // 0xDA
OLED_Command(0x02);
OLED_Command(OLED_SETCONTRAST); // 0x81
OLED_Command(0x8F);
OLED_Command(OLED_SETPRECHARGE); // 0xd9
OLED_Command(0xF1);
OLED_Command(OLED_SETVCOMDETECT); // 0xDB
OLED_Command(0x40);
OLED_Command(OLED_DISPLAYALLON_RESUME);// 0xA4
OLED_Command(OLED_NORMALDISPLAY); // 0xA6
OLED_Command(OLED_DISPLAYON); //--turn on oled panel
}
/*******************************************************************************
* Function: void OLED_YX(unsigned char Row, unsigned char Column)
*
* Returns: Nothing
*
* Description: Sets the X and Y coordinates
*
******************************************************************************/
void OLED_YX(unsigned char Row, unsigned char Column)
{
OLED_Command( 0xB0 + Row);
OLED_Command( 0x00 + (8*Column & 0x0F) );
OLED_Command( 0x10 + ((8*Column>>4)&0x0F) );
}
/*******************************************************************************
* Function: void OLED_PutChar(char ch)
*
* Returns: Nothing
*
* Description: Writes a character to the OLED
*
******************************************************************************/
void OLED_PutChar(char ch )
{
if ( ( ch < 32 ) || ( ch > 127 ) ){
ch = ' ';
}
const uint8_t *base = &OledFont[ch - 32][0];
uint8_t bytes[9];
//bytes[0] = 0x40;
memmove( bytes + 1, base, 8 );
I2C3_Write_Multiple(0x3C,0x40,9,bytes);
}
/*******************************************************************************
* Function: void OLED_Clear()
*
* Returns: Nothing
*
* Description: Clears the OLED
*
******************************************************************************/
void OLED_Clear()
{
for ( uint16_t row = 0; row < 8; row++ ) {
for ( uint16_t col = 0; col < 16; col++ ) {
OLED_YX( row, col );
OLED_PutChar(' ');
}
}
}
/*******************************************************************************
* Function: void OLED_Write_String( char *s )
*
* Returns: Nothing
*
* Description: Writes a string to the OLED
*
******************************************************************************/
void OLED_Write_String( char *s )
{
while (*s) OLED_PutChar( *s++);
}
/*******************************************************************************
* Function: void OLED_Write_Integer ( uint8_t i )
*
* Returns: Nothing
*
* Description: Writes an integer to the OLED
*
******************************************************************************/
void OLED_Write_Integer(uint8_t i)
{
char s[20];
sprintf( s, "%d", i );
OLED_Write_String( s );
OLED_Write_String( " " );
}
/*******************************************************************************
* Function: void OLED_Write_Float( float f )
*
* Returns: Nothing
*
* Description: Writes a float to the OLED
*
******************************************************************************/
void OLED_Write_Float(float f)
{
char* buf11;
int status;
sprintf( buf11, "%f", f );
OLED_Write_String(buf11);
OLED_Write_String( " " );
}
void Delay_ms(int time_ms)
{
int i, j;
for(i = 0 ; i < time_ms; i++)
for(j = 0; j < 3180; j++)
{} /* excute NOP for 1ms */
}
void SystemInit(void)
{
SCB->CPACR |= 0x00f00000;
}
Hardware Demo
Related OLED tutorials:
- DC Motor Speed and Direction Control with TM4C123
- Stepper Motor Interfacing with TM4C123 Tiva Launchpad
- SG-90 Servo Motor Interfacing with TM4C123 Launchpad
- HC-05 Bluetooth Interfacing with TM4C123G Tiva C Launchpad
- 4×4 Keypad Interfacing with TM4C123 Tiva Launchpad
- LCD Interfacing with TM4C123 Tiva LaunchPad
- TM4C123 Timer as a Counter in Input-Edge Count Mode
Do I need to change anything to work in the code composer? I’m putting everything in the main code and it doesn’t work
Hi, we haven’t tried it with code composer studio. But ideally, it should work in code composer studio also if CCS uses same registers name convension.
Can you share which error you are getting?
How do I interface bitmap images on this OLED using the same microcontroller?
Hi ,
This code is working in Keil IDE but not in ccs studio because CCS studio i am not getting reference to enable FPU
void SystemInit(void)
{
SCB->CPACR |= 0x00f00000;
}
This code is writtent and tested in Keil IDE not with CCS studio
First off thanks, saved me sometime. Code mostly worked for 128×64 but it appears (I rewrote the I2C routines)
void OLED_PutChar(char ch ) should only transfer 8 bytes like this:
I2C3_Write_Multiple(0x3C,0x40,8,bytes);
I changed the Initialization parameters as follows for 128×64:
void OLED_Init() {
OLED_Command(OLED_DISPLAYOFF); // 0xAE
OLED_Command(OLED_SETDISPLAYCLOCKDIV); // 0xD5
OLED_Command(0x80); // the suggested ratio 0x80
OLED_Command(OLED_SETMULTIPLEX); // 0xA8
// OLED_Command(0x1F);
OLED_Command(0x3F);
OLED_Command(OLED_SETDISPLAYOFFSET); // 0xD3
OLED_Command(0x0); // no offset
OLED_Command(OLED_SETSTARTLINE | 0x0); // line #0
OLED_Command(OLED_CHARGEPUMP); // 0x8D
OLED_Command(0x14);
OLED_Command(OLED_MEMORYMODE); // 0x20
OLED_Command(0x00); // 0x0 act like ks0108
OLED_Command(OLED_SEGREMAP | 0x1);
OLED_Command(OLED_COMSCANDEC);
OLED_Command(OLED_SETCOMPINS); // 0xDA
OLED_Command(0x12); // Was 0x02
OLED_Command(OLED_SETCONTRAST); // 0x81
OLED_Command(0xcF); // 0xcf
OLED_Command(OLED_SETPRECHARGE); // 0xd9
OLED_Command(0xF1);
OLED_Command(OLED_SETVCOMDETECT); // 0xDB
OLED_Command(0x40);
OLED_Command(OLED_DISPLAYALLON_RESUME);// 0xA4
OLED_Command(OLED_NORMALDISPLAY); // 0xA6
OLED_Command(OLED_DEACTIVATE_SCROLL);
OLED_Command(OLED_DISPLAYON); //–turn on oled panel
}
I also added a 8×16 font and a numbers font 16×32 which makes it a more useful display.
Paul
i have having error
74 warnings generated.
compiling code1.c…
linking…
.\Objects\code1.axf: Error: L6200E: Symbol SystemInit multiply defined (by system_tm4c123.o and code1.o).
Not enough information to list image symbols.
Not enough information to list load addresses in the image map.
Finished: 2 information, 0 warning and 1 error messages.
“.\Objects\code1.axf” – 1 Error(s), 118 Warning(s).
Target not created.
Build Time Elapsed: 00:00:01