If you’re new to Raspberry Pi Pico and want to explore its GPIO capabilities using MicroPython, then you’ve come to the right place. In this tutorial, we will show how to use GPIO pins of Raspberry Pi Pico using MicroPython firmware. Firstly, we will learn how to use GPIO pins as digital output pins. For demonstration purposes, we will connect LEDs with GPIO pins of Raspberry Pi Pico and control them. At the end, we will go through examples of LED blinking and LED chaser using MicroPython and Thonny IDE.
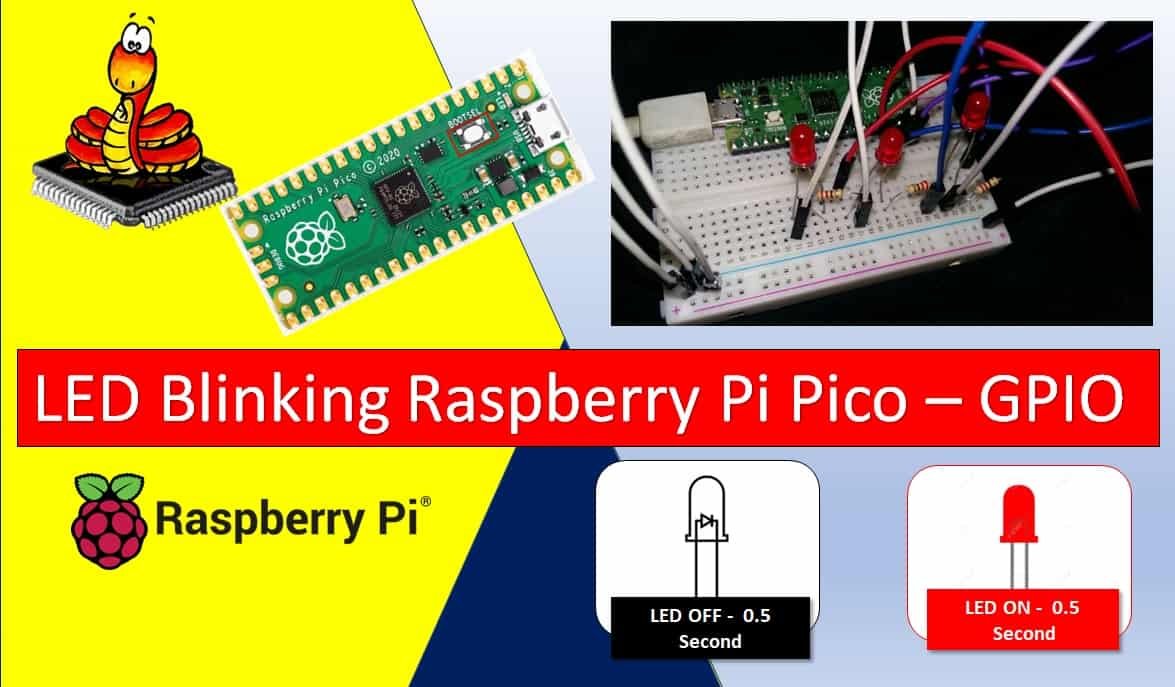
Prerequisites
In order to start, make sure you have performed the following procedures already:
- Download and install the latest version of Python 3 on your PC.
- Downloaded and installed the latest version of Thonny IDE.
- Set up MicroPython in Raspberry Pi Pico.
If you want to know how to perform the steps mentioned above, you can follow this step-by-step guide:
If you are using uPyCraft IDE, you can check this getting started guide:
After following the above-mentioned steps, we will now learn how to blink a single LED with the Raspberry Pi Pico board using MicroPython and the Thonny IDE. To better understand the connections, let’s familiarize ourselves with the GPIOs of the Raspberry Pi Pico.
What are GPIOs?
GPIO stands for General Purpose Input/Output. GPIO pins are digital signal pins found on microcontrollers and single-board computers like the Raspberry Pi Pico. These pins can be configured to either input or output mode and can be used to communicate with other hardware devices or sensors.
In input mode, GPIO pins can be used to read the state of external devices such as buttons, switches, or sensors. They can detect whether the device is in an on or off state, or detect changes in the state of the device.
In output mode, GPIO pins can produce electrical signals that can control other devices like LEDs, motors, or relays. By controlling the state of the pin, you can turn devices on or off, or send different patterns of signals to achieve various functionalities.
GPIO pins offer flexibility and allow users to interface with a wide range of hardware components, making them essential for various applications in the world of embedded systems and electronics.
Raspberry Pi Pico GPIO Pins
Raspberry Pi Pico exposes 26 multi-function GPIO pins from a total of 36 GPIO pins available in the RP2040 microcontroller. Out of these 26 pins, 23 pins are digital pins, and only 3 pins have Analog read capability. These digital pins are marked as GP0, GP1, and up to GP22. GP23, GP24, and GP25 are not exposed on the pinout. Therefore cannot be used. GP26, GP27, and GP28 are the next digital pins available. These 26 GPIO pins can be used both in digital input and digital output mode.
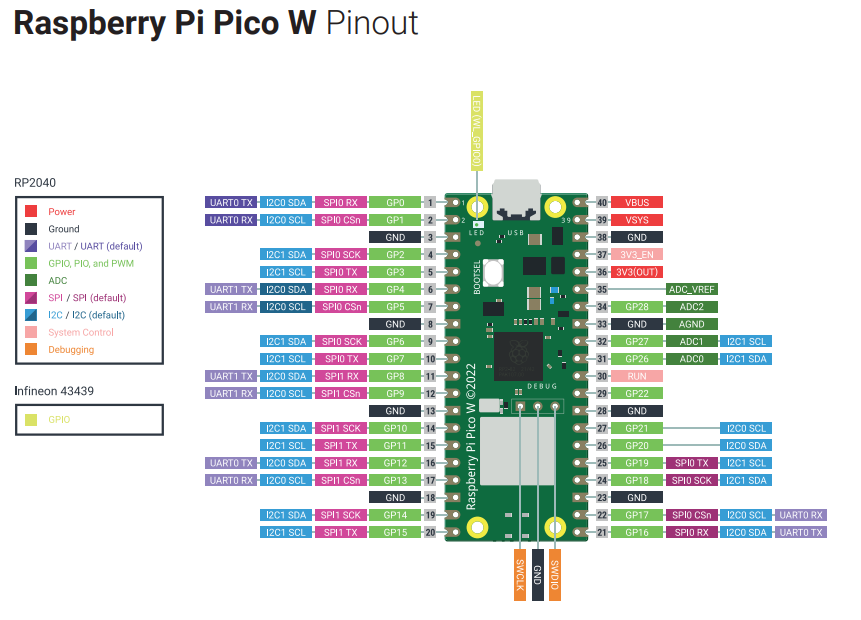
Recommended Reading: Raspberry Pi Pico – Getting Started Guide
MicroPython Digital Outputs
To set Raspberry Pi Pico pins as digital output using MicroPython, you can follow these steps:
Import the necessary modules:
from machine import Pin
The Pin
module is required to interact with the GPIO pins.
- Create an instance of the
Pin
class for the desired pin:
led= Pin(pin_number, Pin.OUT)
Replace pin_number
with the actual pin number you want to configure. For example, if you want to set pin 13 as a digital output, you would use Pin(13, Pin.OUT)
.
- Set the pin value to control the output:
led.value(1) # Set the pin high (output voltage)
Blinking LED Example Raspberry Pi Pico
Now we will start our project to blink an LED using Raspberry Pi Pico in MicroPython with the help of Thonny IDE.

In order to perform this project, we need the following equipment:
- Breadboard
- One LED
- One 220 ohm resistor
- Connecting Wires
- Raspberry Pi Pico board
LED Blinking Connection Raspberry Pi Pico
Now make connections of Raspberry Pi Pico with an LED. Connect GPIO13 of Raspberry Pi Pico with an LED anode terminal through a 220 ohm current limiting resistor. Also, make sure to connect cathode terminal of an LED with the ground pin of the board.
Connect the components as shown in the schematic diagram below:
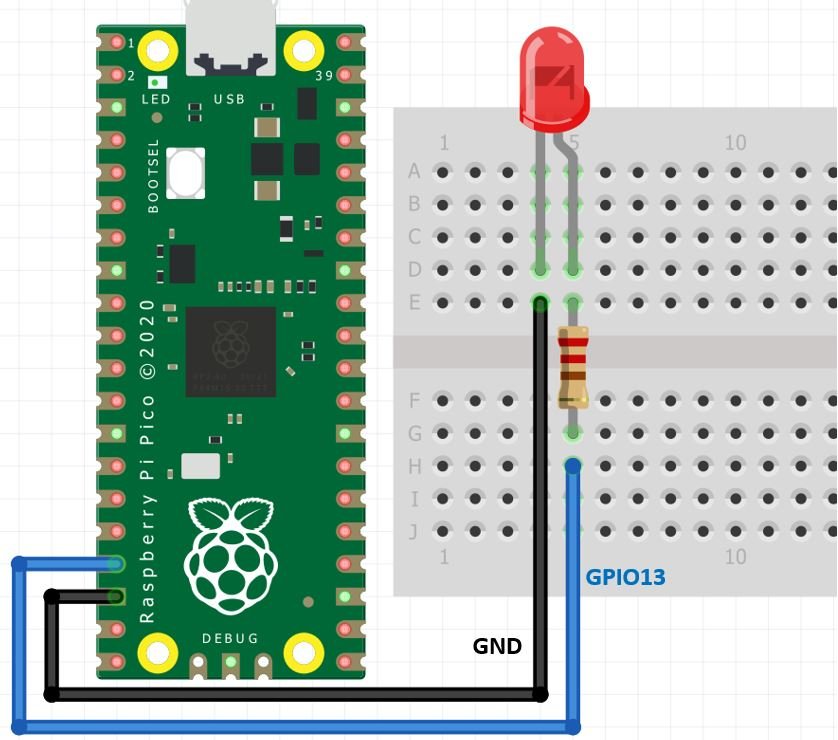
LED | Raspberry Pi Pico |
---|---|
Cathode (-) | GND |
Anode (+) | GPIO13 |
LED Blinking MicroPython Script
Now let’s create an LED blinking script for Raspberry Pi Pico MicroPython.
First, create a new file by clicking on File > New in Thonny IDE.
Copy this code to the new opened untitled window:
import time
from machine import Pin
led=Pin(13,Pin.OUT) #create LED object from pin13,Set Pin13 to output
while True:
led.value(1) #Set led turn on
time.sleep(0.5)
led.value(0) #Set led turn off
time.sleep(0.5)

After that click on the “Save” icon. This gives two options to save this file either to your computer or directly to your devices such as Raspberry Pi Pico. That means we can also directly upload files to a device. But for now, save it on your computer. You can select any location in your computer where you want to save this file. Save it as blink.py
You can use any other name as well just make sure it ends in .py
Now click on the “Run” button to upload code to Raspberry Pi Pico. Before uploading code make sure the correct board is selected.

How Code Works?
In this section, we will see how codes work.
Importing MicroPython GPIO and Time Libraries
In MicroPython, a machine module contains classes of different peripherals of a microcontroller such as GPIO, ADC, PWM, I2C, SPI, etc. In this tutorial, we are using GPIO pins of Raspberry Pi Pico. Therefore, we will import GPIO class from a machine module. Hence, here we import the Pin class from the machine module. We can create multiple instances of the Pin class to configure different GPIO pins of Raspberry Pi Pico.
from machine import Pin
Adding Delays
The time module in MicroPython contains different methods such as delay, sleep, etc. For example, we can use a sleep() method to insert delays into our MicroPython script.
import time
Creating Instance of Pin Class
As mentioned earlier, we can create multiple objects of an “led” class which we defined above. In this line, we create an instance of the Pin object “led”. But user can give any name to an object such as led13, led_13, etc.
led=Pin(13, Pin.OUT)
The Pin() class takes three parameters as shown below:
Pin(Pin_number, pin_mode, pull, value)
- First argument is a pin number to which we want to configure in different modes such as input, output, etc.
- Second argument defines the pin mode such as digital input (Pin.IN), digital output (Pin.OUT), open-drain (OPEN_DRAIN).
- Third argument specifies if we want to enable pull-up and pull-down internal resistor of a GPIO pin. These pull-up and pull-down resistors become handy when we want to use GPIO pins as a digital input pin (PULL_UP, or PULL_DOWN).
- Last argument defines the initial state of GPIO pin as either active high (1) or active low (0). But, by default, the GPIO initial state on reset is 0 (active low).
This is an infinite loop which keeps on running endlessly.
while True: //infinite loop
Setting GPIO Pin State
Pin class contains a function known as value() which is used to set the state of GPIO pins. Passing 1 to this function will set the GPIO pin to an active high state. Similarly, passing 0 to this routine sets the GPIO pin to an active low state. Here, we have set the GPIO13 to an active high state by accessing a value() routine through an object “led”.

led.value(1) // LED is turned on
This gives a delay of 0.5 seconds before the Led turns off again.
time.sleep(0.5) //Delay of 0.5 seconds
Here, we have set the GPIO13 to an active low state by accessing a value() routine through an object “led”.
led.value(0) //LED is turned off
This gives a delay of 0.5 seconds before the Led turns on again, therefore, producing the blinking effect.
time.sleep(0.5) //Delay of 0.5 seconds
Demonstration
After we have saved the code press the Run button to upload the file to your Raspberry Pi Pico module.
You will see an LED blinking at a rate of one second as shown below:
Alternate Method
MicroPython Pin class also provides a method to set GPIO pins to active high or active low state without using any input such parameter know as led.on() and led.off(), where led is an object of a class Pin. This example shows the use of these methods.
import time
from machine import Pin
led=Pin(13,Pin.OUT) #create LED object from pin13,Set Pin13 to output
while True:
led.on() #Set led turn on
time.sleep(0.5)
led.off() #Set led turn off
time.sleep(0.5)
Chasing Three LEDs using Raspberry Pi Pico
Now we will learn how to perform a chasing effect using three LEDs using the given modules in MicroPython. In other words, we will learn to use multiple GPIO pins of the pico board as output pins.

In order to perform this project we need the following equipment:
- Breadboard
- Three LEDs
- Three 300 ohm resistors
- Connecting Wires
- Raspberry Pi Pico
Connect the components as shown in the schematic diagram below:

LED Chaser Script MicroPython
from machine import Pin
from time import sleep
led_13 = Pin(13, Pin.OUT)
led_14 = Pin(14, Pin.OUT)
led_12 = Pin(12, Pin.OUT)
while True:
led_12.value(0)
led_13.value(1)
led_14.value(0)
sleep(0.5)
led_12.value(1)
led_13.value(0)
led_14.value(0)
sleep(0.5)
led_12.value(0)
led_13.value(0)
led_14.value(1)
sleep(0.5)
To see the demo of the above code, save this code to a new file in Thonny IDE and upload it to your board.
Conclusion
In conclusion, this tutorial provided guide on how to use the GPIO (General Purpose Input/Output) pins of the Raspberry Pi Pico using MicroPython firmware. It lists the steps to configure GPIO pins as digital outputs and demonstrated examples of LED blinking and LED chaser projects using MicroPython and the Thonny IDE. By following this tutorial, readers will gain understanding of how to use the GPIO pins of the Raspberry Pi Pico as digital output pins.
You may also like to read:
I am trying to use the piSquared which I understand as a pico deritive. I get an error when I try to use “from machine import Pin”. The error that I’m getting is “ImportError: can’t import name pin”. I also tried to po BUT GET THE SAME ERROR.
any help would be appreciated.