By default, the hostname of ESP32 based development boards is espressif. But we can change the default hostname by using WiFi.setHostname() function. In this tutorial, we will learn to set the custom hostname for the ESP32 board using Arduino IDE.
In Arduino IDE, we can change the hostname of ESP32 by calling WiFi.setHostname(YOUR_NEW_HOSTNAME);
before WiFi.begin();
What is Hostname?
A hostname is a label or a unique name that is assigned to a device connected to a computer network. It’s used to identify the device within the network, making it easier for users to access and communicate with that device. Hostnames are often used in the context of the Domain Name System (DNS), which translates human-readable hostnames into IP addresses that computers can use to locate and communicate with each other over the internet or a local network.
Changing ESP32 Hostname
The default hostname of ESP32 is “expressif”. However, if you are working with multiple ESP32 devices and want to differentiate them by their name while using them in soft access point mode, you can change the hostname of these devices.
For example, if you have multiple nodes in your IoT-based project such as temperature, humidity, pressure, devices control, and light control node, you can customize the hostname for each node.
As you may know, the Arduino WiFi.h library for ESP32 provides all the necessary methods to configure the boards in station and access point mode.
The WiFi.h library also provides a function or method to set a custom hostname for ESP32 devices.
ESP32 Code to Get Default Hostname
In the Arduino framework ESP32, you can use the WiFi library to get the default hostname of the ESP32 devices. Here’s an example code snippet that demonstrates how to retrieve and print the default hostname:
#include <WiFi.h>
void setup() {
Serial.begin(115200);
delay(1000);
// Initialize WiFi
WiFi.begin("your_wifi_ssid", "your_wifi_password");
// Wait for WiFi connection
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
// Print the default hostname
Serial.print("Default Hostname: ");
Serial.println(WiFi.getHostname());
}
void loop() {
// Your main code here
}
Replace “your_wifi_ssid” and “your_wifi_password” with your actual WiFi credentials. The WiFi.getHostname() function retrieves the default hostname set for the ESP32 module.
Create Custom Hostname ESP32
First, define a custom name for your node using a string as follows.
String hostname= "ESP32 Humidity Node";
Let’s say we want to set the name of ESP32 as “ESP32 Humidity Node”. Therefore, we create a string variable (hostname_hum). A variable name can be any name of your choice.
The WiFi.setHostname() function is used to set a custom hostname. You should call this function before wiFi.begin().
WiFi.config(INADDR_NONE, INADDR_NONE, INADDR_NONE, INADDR_NONE);
WiFi.setHostname(hostname.c_str()); //define hostname
ESP32 Code
This code establishes an ESP32 connection to a WiFi network and sets a custom hostname. Copy the following sketch to Arduino and upload it to ESP32.
#include <WiFi.h>
// Replace with your network credentials (STATION)
const char* ssid = "REPLACE_WITH_YOUR_SSID";
const char* password = "REPLACE_WITH_YOUR_PASSWORD";
String hostname = "ESP32 Humidity Node";
void initWiFi() {
WiFi.mode(WIFI_STA);
WiFi.config(INADDR_NONE, INADDR_NONE, INADDR_NONE, INADDR_NONE);
WiFi.setHostname(hostname.c_str()); //define hostname
//wifi_station_set_hostname( hostname.c_str() );
WiFi.begin(ssid, password);
Serial.print("Connecting to WiFi ..");
while (WiFi.status() != WL_CONNECTED) {
Serial.print('.');
delay(1000);
}
Serial.println(WiFi.localIP());
}
void setup() {
Serial.begin(115200);
initWiFi();
Serial.print("New Hostname: ");
Serial.println(WiFi.getHostname());
}
void loop() {
// put your main code here, to run repeatedly:
}
How Does Code Work?
The #include line at the beginning of the code includes the WiFi library, which provides functions for connecting to WiFi networks and managing network-related tasks.
#include <WiFi.h>
The lines const char* ssid = “REPLACE_WITH_YOUR_SSID”; and const char* password = “REPLACE_WITH_YOUR_PASSWORD”; define the WiFi network’s SSID (name) and password. You need to replace these placeholders with your actual network credentials.
Hostname Definition
The line String hostname = “ESP32 Humidity Node”; defines a custom hostname for the ESP32 device. In this case, the hostname is set to “ESP32 Humidity Node”. You can change this value to give your device a different hostname.
WiFi Initialization (initWiFi function):
The initWiFi() function is responsible for setting up the WiFi connection and configuring the hostname. Here’s what happens inside this function:
WiFi.mode(WIFI_STA);: Configures the ESP32 to operate in a station (client) mode, connecting to a WiFi network.
WiFi.config(INADDR_NONE, INADDR_NONE, INADDR_NONE, INADDR_NONE);: This line configures the WiFi IP settings. It sets all address fields to INADDR_NONE, indicating that the ESP32 should obtain its IP settings through DHCP.
Setting Hostname
WiFi.setHostname(hostname.c_str());: This line sets the previously defined hostname as the device’s hostname. The .c_str() method converts the String object to a C-style string required by the WiFi library.
WiFi.begin(ssid, password);: Initiates the connection to the WiFi network using the provided SSID and password.
The following lines (Serial.print(“Connecting to WiFi ..”);, while (WiFi.status() != WL_CONNECTED), and delay(1000);) show a progress indicator while the ESP32 is trying to connect to the WiFi network.
Demonstration
Upload the above example code which will change the Hostname of ESP32 to “ESP32 Humidity Node”. After uploading the code, restart your ESP32 board and click on the reset button as shown:
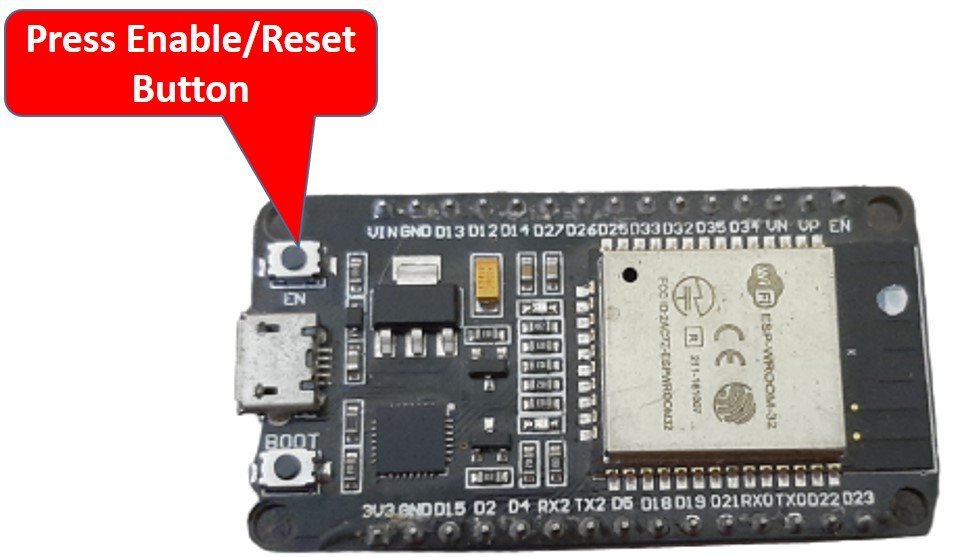
Now open your router settings page and see the hostname assigned to ESP32. It will be changed to “ESP32 Humidity Node”.
Note: If the hostname is not changing for you, in that case, you should restart your WiFi router.
If you don’t know how to check your router network settings page, you can install a Fing Android application to view the hostname of your ESP32 device.
Conclusion
In short, in this tutorial, we have learned how to change the default hostname of ESP32 devices. Changing default hostname becomes handly in IoT base projects when we have multiple nodes in our IoT project and we want to identify each node with its unique hostname.
You may also like to read:
- ESP32/ESP8266 Momentary Switch Web Server: Control GPIO Outputs
- Telegram ESP32 and ESP8266: Control GPIOs and LEDs using Arduino IDE
- ESP32 Bluetooth Low Energy (BLE) using Arduino IDE
- Reconnect ESP32 to WIFI after Lost Connection (Solved)
- Use ESP32 Bluetooth Classic with Arduino IDE
- ESP32/ESP8266 Web Server to Control Outputs with a Timer (Pulse Width)
- Save Data to ESP32 Flash Permanently using Preferences Library
- HTTP GET using ESP32 and Arduino IDE (OpenWeatherMap.org and ThingSpeak)
- ESP32 HTTP POST using Arduino IDE (ThingSpeak and IFTTT)
Hello,
Thanks for this.
I’ve tested and did not work for me.
However, I did some changes :
1/ removed WiFi.config(INADDR_NONE, INADDR_NONE, INADDR_NONE, INADDR_NONE);
as this made the esp to receive 255.255.255.255 address!
2/ moved WiFi.setHostname(hostname.c_str()); before WiFi.mode(WIFI_STA);
now all is fine.
Not sure this has an effect, but I’m using the event driven wifi WiFi.onEvent(WiFiEventStatic); to manage connection.
Hope this can help.
thanks, you’re comment/update works for me
Thank, we will check this and update article accordingly.
Hey,
I tried the normal code first and then your solution, but nothing seems to make it work for me :(. Do you have any suggestions on what to do?
With an ESP32-S the above mentioned solution did not work.
However when changing the order of of code to this:
const char* newHostname = “ESP32-Test”;
WiFi.setHostname(newHostname); //define hostname
WiFi.mode(WIFI_STA); // station mode
WiFi.begin(ssid, password);
the newHostname was accepted and reacable via ping ESP32-Test