In this Arduino project, we will create a home automation system that will control home appliances via PC or laptop through a serial port. The method we use in this project is serial communication with a computer. The serial monitor of Arduino IDE will be used to send commands that will control the electronic devices by switching them on and off.
In the modern era, everyone wants ease of life with fewer difficulties. Nowadays Home automation or Smart homes improve living standards. They give us the access to switch on and off any device or appliance easily and efficiently. There are various ways to communicate with devices like IR remote, GSM module, Bluetooth, Wifi and through serial communication with the computer.

PC Based Home Automation System Overview
We will require the following components for this project.
Required Components:
- Arduino Uno: We will use Arduino due to its easy use. It also provides several digital pins to interface with the LCD and relay module at the same time. It is very friendly when you prototyping any project.
- 4 Channel Relay Modules: The module we will use in this project is HL-54S. It switches on and off using a 5V logic signal from Arduino. It can handle up to 250VAC and 10A. These modules have 4 relays so we can control 4 AC devices or appliances.
- 16×2 LCD: LCD is used to display the project name, a list of commands which to be entered then it asks to give any command and shows the status of the command which is entered. We use 16×2 LCD because it is easy to interface with Arduino and very cheap in price. 10k potentiometer is used to control the contrast of display
- AC bulbs with holders: AC bulbs are used to represent devices and appliances. Because it is easy to handle and very useful when you are prototyping any AC project. In the final product, you can just replace it with an AC socket to control.
- AC wire with plug: Use good quality wire when working with higher voltages. It is always good to use electrical tape to cover connections.
- External 5 volt supply: 5-volt dc supply is required to switch the relay ON and OFF. Otherwise, it does not work. Also do not supply 5v from Arduino.
How does PC Based Home Automation Work?
You can view the operations constituting the PC based home automation system in the block diagram below:

- In this PC based home automation system, Arduino Uno is used as the brain of our circuit.
- The 16×2 LCD is used to show messages.
- 4 channel relay module is used to switch devices on and off.
- A computer is used to do serial communication with Arduino.
- We will use AC bulbs instead of devices. For demonstration purposes, we have used three AC lamps. We will send commands from PC to Arduino to turn on and turn off these devices.
Connection Diagram of PC Controlled Home Automation Project
In this section, we will explain the connections of the various components specified above with Arduino Uno to form the home automation system.

Let us discuss them one by one.
Arduino with 16×2 LCD
We will use a 16×2 LCD in our project to display the states of the bulbs. To connect a 16×2 LCD with Arduino we will require an additional 10k potentiometer as well.
We have a dedicated tutorial regarding interfacing 16×2 LCD display with Arduino with some example sketches. Have a look at it before proceeding further for a better understanding of the LCD.
Recommended Reading: 16×2 LCD Interfacing with Arduino – Explained with Example Codes
There are two types of pins on the whole 16×2 LCD module. Some pins are used to send to 16×2 LCD and some are command pins. In other words, every pin has a role in controlling a single pixel on the display.16 x 2 LCD has sixteen columns and two rows. That means, it can display sixteen characters per row and it has two such rows.
Pinout
The diagram shows the pin configuration of a 16×2 LCD display. It has sixteen pins.
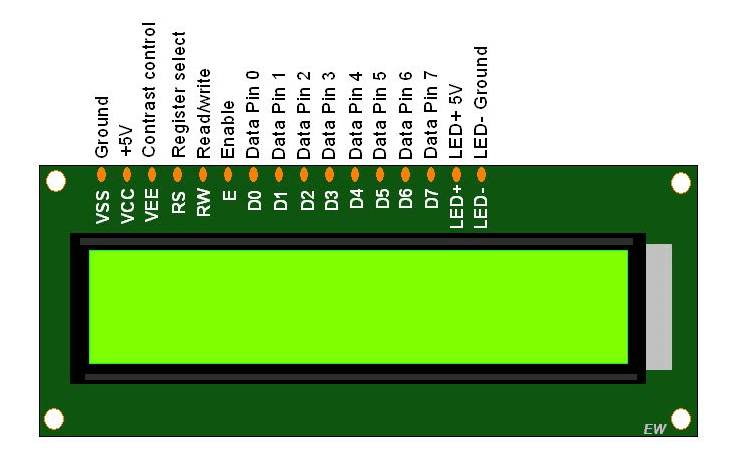
- D0 – D7: Pin number 7-14 are data bus lines that are used to send data from Arduino which you want to display on LCD. With these 8 data lines, data can be transferred either in an 8-bit format or in a 4-bit format. In a 4-bit format, only upper four bits (D4-D7) are used to send data from Arduino to LCD. The full byte is transmitted in two successive transmissions. A 4-bit format is used to save GPIO pins of Arduino. Because fewer GPIO pins of Arduino will be required to transfer data.
- Contrast Select (VEE): It will help to control the contrast of PIXELS according to the 16X2 LCD light.
- RS: This pin is known as a register select pin. It helps to toggle the command/data register.
- R/W: The signal on this pin will decide whether it is going to read from LCD or write on it.
- EN: Enable pin will help to transfer the instruction from the data pins and another command pin to the LCD. It acts as permission to internal registers.
- VSS: It’s a ground pin for common grounds.
- VCC: The power pin will use for voltage input to the 16X2 LCD.
Arduino connections with 16×2 LCD
We are using the following connections as described below. Refer to the schematic diagram to have a clearer idea of the connections.
16×2 LCD | Arduino |
D4 – D7 | Pin 10,11,12,13 |
Enable | Pin 9 |
RS | Pin 8 |
RW | GND |
VEE | 10k POT (Middle Leg) |
VSS | GND |
VCC | +5V |
LED+ | +5V |
LED- | GND |
We have an Arduino library for easy communication between LCDs called the LiquidCrystal library. It is an inbuilt library by Arduino Adafruit version.
Arduino with Channel Relay modules
The relay module for Arduino is one of the most powerful applications for Arduino as it can be used to control both A.C and D.C devices by simply controlling the relay by giving 5V. A relay is basically a switch which is operated electrically by an electromagnet. A relay can be used to control high voltage electronic devices such as motors as well as low voltage electronic devices such as a light bulb or a fan.
We will be using the 4 relay Arduino module in our home automation project.

- External 5 volt to JD VCC.
- Ground to ground.
- IN1 to Pin 3.
- IN2 to Pin 4.
- IN3 to Pin 5.
- VCC to Arduino 5V.
- Connect one end of all bulbs to normally open terminal of relays.
- One end of 220VAC to all common terminals of the relay and another end with another terminal of bulbs.
Working PC Controlled Home Automation using Arduino
We will send a specified command through the Arduino serial monitor. The Arduino will fetch the desired instruction from the message and perform the required operation such as turn the devices ON/OFF and display their status on the LCD.
For demonstration purposes, we will use different colored bulbs: white, blue and green to depict a light, fan and tv.
The table below shows the commands that we will send using the Arduino serial and its corresponding functions that will occur:
Command | Function |
---|---|
FAN ON | The fan will turn on. In our case, the blue bulb will turn on. |
FAN OFF | The fan will turn off. In our case, the blue bulb will turn off. |
LIGHT ON | The light will turn on. In our case, the white bulb will turn on. |
LIGHT OFF | The light will turn off. In our case, the white bulb will turn off. |
TV ON | The tv will turn on. In our case, the green bulb will turn on. |
TV OFF | The tv will turn off. In our case, the green bulb will turn off. |
PC Controlled Home Automation System Arduino Code
We will use Arduino IDE to program our Arduino board. Open your Arduino IDE and go to File > New to open a new file. Copy the code given below in that file and save it.
#include <LiquidCrystal.h>
#include<string.h>
LiquidCrystal lcd(8, 9, 10, 11, 12, 13);
#define light 3
#define fan 4
#define TV 5
char temp;
char str[10];
char i = 0;
void setup()
{
lcd.begin(16, 2);
Serial.begin(9600);
pinMode(light, OUTPUT);
pinMode(fan, OUTPUT);
pinMode(TV, OUTPUT);
digitalWrite(light, HIGH);
digitalWrite(fan, HIGH);
digitalWrite(TV, HIGH);
lcd.print("MICROCONTROLLERS ");
lcd.setCursor(0, 1);
lcd.print(" LAB ");
delay(2000);
lcd.clear();
lcd.print("HOME AUTOMATION ");
lcd.setCursor(0, 1);
lcd.print(" USING PC ");
delay(2000);
lcd.clear();
lcd.print("1. LIGHT ON");
lcd.setCursor(0, 1);
lcd.print("2. LIGHT OFF");
delay(2000);
lcd.clear();
lcd.print("3. FAN ON");
lcd.setCursor(0, 1);
lcd.print("4. FAN OFF");
delay(2000);
lcd.clear();
lcd.print("5. TV ON");
lcd.setCursor(0, 1);
lcd.print("6. TV OFF");
delay(2000);
defualt();
delay(1000);
}
void loop()
{
if (temp == 1)
{
if ((strncmp(str, "FAN ON", 6)) == 0)
{
lcd.clear();
digitalWrite(fan, LOW);
lcd.clear();
lcd.print("Fan Turned On");
delay(3000);
defualt();
}
else if (strncmp(str, "FAN OFF", 7) == 0)
{
digitalWrite(fan, HIGH);
lcd.clear();
lcd.print("Fan Turned OFF");
delay(3000);
defualt();
}
else if (strncmp(str, "LIGHT ON", 8) == 0)
{
digitalWrite(light, LOW);
lcd.clear();
lcd.print("Light Turned ON");
delay(3000);
defualt();
}
else if (strncmp(str, "LIGHT OFF", 9) == 0)
{
digitalWrite(light, HIGH);
lcd.clear();
lcd.print("Light Turned OFF");
delay(3000);
defualt();
}
else if (strncmp(str, "TV ON", 5) == 0)
{
digitalWrite(TV, LOW);
lcd.clear();
lcd.print("TV Turned ON");
delay(3000);
defualt();
}
else if (strncmp(str, "TV OFF", 6) == 0)
{
digitalWrite(TV, HIGH);
lcd.clear();
lcd.print("TV Turned OFF");
delay(3000);
defualt();
}
else
{
lcd.clear();
lcd.print(" Invalid Input");
lcd.setCursor(0, 1);
lcd.print(" Try Again ");
delay(3000);
defualt();
}
}
}
void serialEvent()
{
while (Serial.available())
{
char Inchar = Serial.read();
str[i] = Inchar;
i++;
lcd.print(Inchar);
delay(50);
if (Inchar == 0x0d)
{
temp = 1;
//Inchar=0;
}
}
}
void defualt()
{
lcd.clear();
lcd.print("Enter UR Choice");
lcd.setCursor(0, 1);
lcd.cursor();
i = 0;
temp = 0;
}
How the Code Works?
We will start by including the necessary libraries required for this project as shown below:
#include <LiquidCrystal.h>
#include<string.h>
Next, we declare the Arduino pins that are connected with the LCD. To define connections, we use the following line of code. This line creates a LiquidCrystal object and lcd is a name of the object that we are going to use to call LCD functions. You can also use any other name.
LiquidCrystal lcd(rs, en, d4, d5, d6, d7)
In our case, the pins are 8, 9, 10, 11, 12, and 13 respectively.
LiquidCrystal lcd(8, 9, 10, 11, 12, 13);
Specify the Arduino pins connected with IN1, IN2 and IN3 of 4 Channel Relay Module. These pins will control light, fan and tv. For demonstration purposes we will use different colored bulbs to depict the electronic devices.
#define light 3
#define fan 4
#define TV 5
Moreover, we will declare some char variables that we will use later on to monitor the states of the devices.
char temp;
char str[10];
char i = 0;
setup()
Open the serial communication at a baud rate of 9600.
Serial.begin(9600);
Next, we use lcd.begin() routine to define the size of an LCD. The first argument to this function is a number of rows and the second argument is a number of columns. For instance, this line declares the size as 16 columns and 2 rows. That means 16×2 size.
lcd.begin(16, 2);
Configure the pins connected with the electronic devices as output pins. This is done by using the pinMode() function. Specify the pin as the first parameter and the mode (OUTPUT/INPUT) as the second parameter.
pinMode(light, OUTPUT);
pinMode(fan, OUTPUT);
pinMode(TV, OUTPUT);
Moreover, set the value of the pins connected with the electronic devices to HIGH by using digitalWrite() function. Specify the pin as the first parameter and the value as the second parameter. This will ensure that all the bulbs are off initially.
digitalWrite(light, HIGH);
digitalWrite(fan, HIGH);
digitalWrite(TV, HIGH);
Print a series of messages on the LCD display with delays in between. The LCD will display the commands required to control the electronic devices.
lcd.print("MICROCONTROLLERS ");
lcd.setCursor(0, 1);
lcd.print(" LAB ");
delay(2000);
lcd.clear();
lcd.print("HOME AUTOMATION ");
lcd.setCursor(0, 1);
lcd.print(" USING PC ");
delay(2000);
lcd.clear();
lcd.print("1. LIGHT ON");
lcd.setCursor(0, 1);
lcd.print("2. LIGHT OFF");
delay(2000);
lcd.clear();
lcd.print("3. FAN ON");
lcd.setCursor(0, 1);
lcd.print("4. FAN OFF");
delay(2000);
lcd.clear();
lcd.print("5. TV ON");
lcd.setCursor(0, 1);
lcd.print("6. TV OFF");
Moreover, we will call the default() function as well.
delay(2000);
defualt();
delay(1000);
This function clears the LCD screen and prompts the user to enter their choice by displaying a relevant message. Additionally, the variable ‘i’ and ‘temp’ are both set to zero.
void defualt()
{
lcd.clear();
lcd.print("Enter UR Choice");
lcd.setCursor(0, 1);
lcd.cursor();
i = 0;
temp = 0;
}
loop()
Inside the infinite loop() we first check if the temporary variable we created is ‘1’ or not. If it is then we will use a series of if statements to check whether the string received in the buffer is same as that of a predefined string. In the first case, if ‘FAN ON’ command was sent, then turn the fan on. Otherwise, if ‘FAN OFF’ command was sent, then turn the fan off. Likewise, if ‘LIGHT ON’ command was sent then turn the light ON. Otherwise, if ‘LIGHT OFF’ command was sent, then turn the light OFF. Likewise, if ‘TV ON’ command was sent then turn the tv ON. Otherwise, if the ‘TV OFF’ command was sent, then turn the tv OFF. Moreover, if an invalid command is sent then the LCD will display a relevant message.
The LCD will continuously display messages about the state of the respective appliance as it changes at each step. Moreover, the default() function will be called at each step as well.
void loop()
{
if (temp == 1)
{
if ((strncmp(str, "FAN ON", 6)) == 0)
{
lcd.clear();
digitalWrite(fan, LOW);
lcd.clear();
lcd.print("Fan Turned On");
delay(3000);
defualt();
}
else if (strncmp(str, "FAN OFF", 7) == 0)
{
digitalWrite(fan, HIGH);
lcd.clear();
lcd.print("Fan Turned OFF");
delay(3000);
defualt();
}
else if (strncmp(str, "LIGHT ON", 8) == 0)
{
digitalWrite(light, LOW);
lcd.clear();
lcd.print("Light Turned ON");
delay(3000);
defualt();
}
else if (strncmp(str, "LIGHT OFF", 9) == 0)
{
digitalWrite(light, HIGH);
lcd.clear();
lcd.print("Light Turned OFF");
delay(3000);
defualt();
}
else if (strncmp(str, "TV ON", 5) == 0)
{
digitalWrite(TV, LOW);
lcd.clear();
lcd.print("TV Turned ON");
delay(3000);
defualt();
}
else if (strncmp(str, "TV OFF", 6) == 0)
{
digitalWrite(TV, HIGH);
lcd.clear();
lcd.print("TV Turned OFF");
delay(3000);
defualt();
}
else
{
lcd.clear();
lcd.print(" Invalid Input");
lcd.setCursor(0, 1);
lcd.print(" Try Again ");
delay(3000);
defualt();
}
}
}
serialEvent()
This function is automatically called at the end of loop() when there is serial data available in the buffer. In our case, while the serial data is available in the buffer then the characters are added to the array str. The LCD will display the command entered by the user. Then after a slight delay, if the available character is ‘0x0d’ which indicates a Newline character, then the temporary variable will be set to 1.
void serialEvent()
{
while (Serial.available())
{
char Inchar = Serial.read();
str[i] = Inchar;
i++;
lcd.print(Inchar);
delay(50);
if (Inchar == 0x0d)
{
temp = 1;
}
}
}
Demonstration

Make sure you choose the correct board and COM port before uploading your code to the board. Go to Tools > Board and select Arduino UNO. Next, go to Tools > Port and select the appropriate port through which your board is connected.
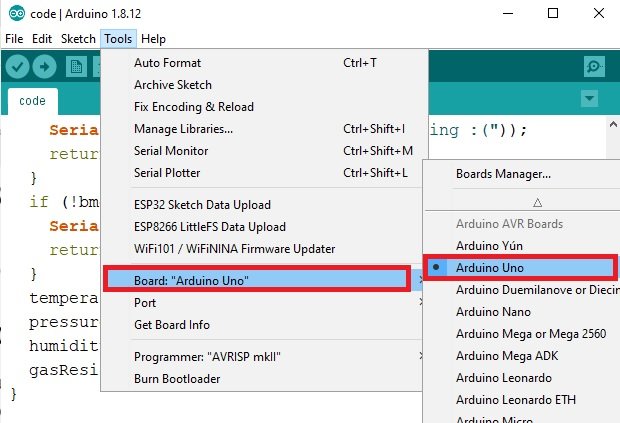
Click on the upload button to upload the code to the board.
After you have uploaded your code to the development board, open the serial monitor and set the baud rate to 9600. Now type valid commands that we set in code to control the electronic devices. Simultaneously, the LCD will also display the current state of the device.
You can watch this video for demo:
You may also like to read:
- WiFi based Home Automation System over cloud using Arduino
- Ethernet based Home Automation using Arduino – IOT
- Bluetooth Based Home Automation project using Arduino
- GSM Based Home Automation project using Arduino
- Voice Controlled Home Automation using Arduino
- IR Remote Controlled Home Automation System using Arduino