In this Arduino project, we will create a home automation system that will control home appliances via infrared IR transmitter and receiver. Using an IR remote/ TV remote we will be able to control devices wirelessly from a distance.

IR Remote Controlled Home Automation System Overview
We will require the following components for this project.
Required Components:
- Arduino Uno: We will use Arduino due to its easy use. It also provides several digital pins to interface with the LCD, IR, and relay module at the same time. It is very friendly when you prototyping any project.
- 4 Channel Relay Modules: The module we will use in this project is HL-54S. It switches on and off using a 5V logic signal from Arduino. It can handle up to 250VAC and 10A. These modules have 4 relays so we can control 4 AC devices or appliances.
- IR remote and receiver: IR remote sends an infrared signal which is received by the IR receiver. Each button on the remote has its unique value. We will use the VS1838 IR remote in this project.
- 16×2 LCD: LCD will be used to display the states of the bulbs in our project. We will use 16×2 LCD because it is easy to interface with Arduino and very cheap in price. 10k potentiometer is used to control the contrast of the display.
- AC bulbs with holders: AC bulbs are used to represent devices and appliances. Because it is easy to handle and very useful when you are prototyping any AC project. In the final product, you can just replace it with an AC socket to control.
- AC wire with plug: Use good quality wire when working with higher voltages. It is always good to use electrical tape to cover connections.
- External 5 volt supply: 5-volt dc supply is required to switch the relay ON and OFF. Otherwise, it does not work. Also do not supply 5v from Arduino.
How does IR Remote Controlled Home Automation Work?
In this IR remote-controlled home automation system, Arduino Uno is used as the brain of our circuit.
- The IR receiver receives a signal from the remote.
- These signals are read by the Arduino and compared with defined values in the code.
- If the values are the same then it performs its corresponding operation like turning on and off the relay or displaying messages on LCD.
You can view the operations constituting the IR remote control home automation system in the block diagram below

Connection Diagram of IR Remote Controlled Home Automation Project
In this section, we will explain the connections of the various components specified above with Arduino Uno to form the home automation system.
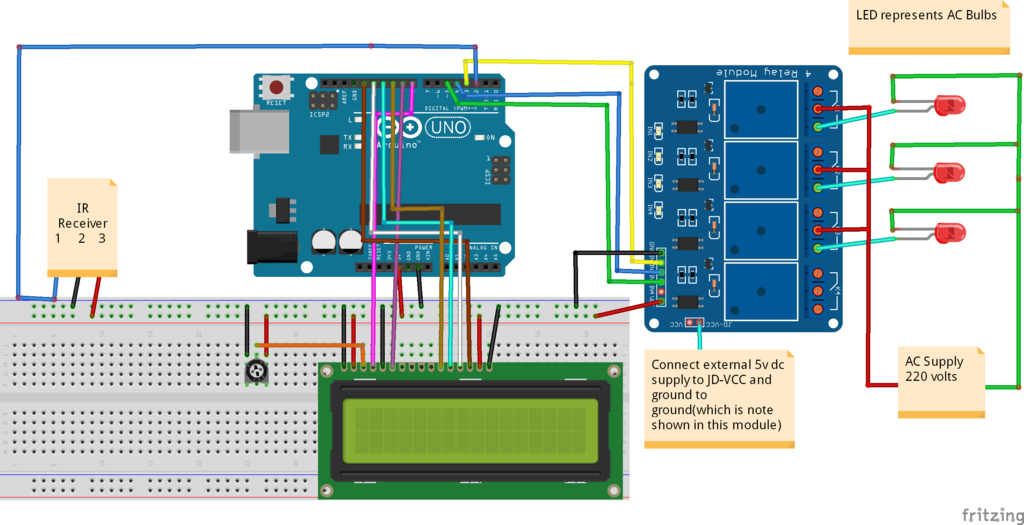
Let us discuss them one by one.
Arduino with Receiver
As mentioned before, we will use VS1838 IR receiver for this project. The figure below shows its pinout.

The VS1838 has three terminals which we saw above in the pinout. The first terminal is the signal pin that will be connected with a digital pin of Arduino. The ground pin of the receiver, which is the middle terminal, is connected with the ground pin of Arduino. The third terminal is VCC, powered by 5V from Arduino.
The table below shows the connections that we will be using:
IR Receiver VS1838 | Arduino Uno |
---|---|
Signal | Pin 2 |
GND | GND |
VCC | 5V |
Arduino with 16×2 LCD
We will use a 16×2 LCD in our project to display the states of the bulbs. To connect a 16×2 LCD with Arduino we will require an additional 10k potentiometer as well.
We have a dedicated tutorial regarding interfacing 16×2 LCD display with Arduino with some example sketches. Have a look at it before proceeding further for a better understanding of the LCD.
Recommended Reading: 16×2 LCD Interfacing with Arduino – Explained with Example Codes
There are two types of pins on the whole 16×2 LCD module. Some pins are used to send to 16×2 LCD and some are command pins. In other words, every pin has a role in controlling a single pixel on the display.16 x 2 LCD has sixteen columns and two rows. That means, it can display sixteen characters per row and it has two such rows.
Pinout
The diagram shows the pin configuration of a 16×2 LCD display. It has sixteen pins.
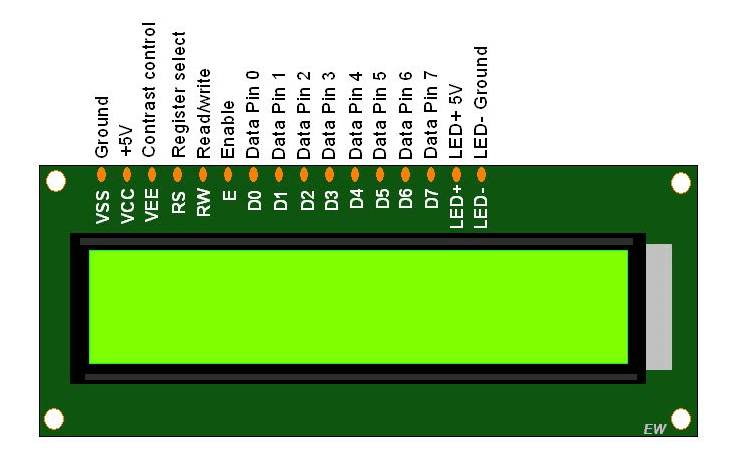
- D0 – D7: Pin number 7-14 are data bus lines that are used to send data from Arduino which you want to display on LCD. With these 8 data lines, data can be transferred either in an 8-bit format or in a 4-bit format. In a 4-bit format, only upper four bits (D4-D7) are used to send data from Arduino to LCD. The full byte is transmitted in two successive transmissions. A 4-bit format is used to save GPIO pins of Arduino. Because fewer GPIO pins of Arduino will be required to transfer data.
- Contrast Select (VEE): It will help to control the contrast of PIXELS according to the 16X2 LCD light.
- RS: This pin is known as a register select pin. It helps to toggle the command/data register.
- R/W: The signal on this pin will decide whether it is going to read from LCD or write on it.
- EN: Enable pin will help to transfer the instruction from the data pins and another command pin to the LCD. It acts as permission to internal registers.
- VSS: It’s a ground pin for common grounds.
- VCC: The power pin will use for voltage input to the 16X2 LCD.
Arduino connections with 16×2 LCD
We are using the following connections as described below. Refer to the schematic diagram to have a clearer idea of the connections.
16×2 LCD | Arduino |
---|---|
D4 – D7 | Pin 10,11,12,13 |
Enable | Pin 9 |
RS | Pin 8 |
RW | GND |
VEE | 10k POT (Middle Leg) |
VSS | GND |
VCC | +5V |
LED+ | +5V |
LED- | GND |
We have an Arduino library for easy communication between LCDs called the LiquidCrystal library. It is an inbuilt library by Arduino Adafruit version.
Arduino with Channel Relay modules
The relay module for Arduino is one of the most powerful applications for Arduino as it can be used to control both A.C and D.C devices by simply controlling the relay by giving 5V. A relay is basically a switch which is operated electrically by an electromagnet. A relay can be used to control high voltage electronic devices such as motors as well as low voltage electronic devices such as a light bulb or a fan.
We will be using the 4 relay Arduino module in our home automation project.

- External 5 volt to JD VCC.
- Ground to ground.
- IN1 to Pin 3.
- IN2 to Pin 4.
- IN3 to Pin5.
- VCC to Arduino 5v.
- Connect one end of all bulbs to the normally open terminal of relays.
- One end of 220VAC to all common terminals of relay and other ends with other terminals of light lamps.
IR Remote Controlled Home Automation Arduino Code
We will use Arduino IDE to program our Arduino board.
Installing IRremote Library
Before we start coding, we will need to install the IRremote library necessary for this project.
To install the library, we will use the Arduino Library Manager. Open your Arduino IDE and go to Sketch > Include Libraries > Manage Libraries. Type ‘IRremote’ in the search bar and install the latest version.

Arduino Sketch
Open your Arduino IDE and go to File > New to open a new file. Copy the code given below in that file and save it.
Each button on the IR remote has a unique value. So we can use each button separately. We used three buttons labelled as “1. 2. 3.” Each button has its own performance in the code. When we will press the button from remote, it will send infrared signal which then is received by the IR receiver. Arduino will read the signal and perform the corresponding operation like switch on and off appliances and display status on LCD.
When we press button one “1” from the remote Arduino reads the value and compares it with the value defined in code which is “16724175.” If it is equal than Arduino will switch on the first relay and the bulb will light up. If it is pressed again the bulb will light off. Other two buttons work in a similarly.
#include <LiquidCrystal.h>
#include<string.h>
#include <boarddefs.h>
#include <IRremote.h>
#include <IRremoteInt.h>
#include <ir_Lego_PF_BitStreamEncoder.h>
LiquidCrystal lcd(8, 9, 10, 11, 12, 13);
#define bulb1 3
#define bulb2 4
#define bulb3 5
int RECV_PIN = 2;
int itsON[] = {1,1,1,1};
#define button1 16724175
#define button2 16718055
#define button3 16743045
IRrecv irrecv(RECV_PIN);
decode_results results;
char temp;
char str[10];
char i=0;
void setup()
{
irrecv.enableIRIn();
lcd.begin(16, 2);
Serial.begin(9600);
pinMode(bulb1, OUTPUT);
pinMode(bulb2, OUTPUT);
pinMode(bulb3, OUTPUT);
digitalWrite(bulb1, HIGH);
digitalWrite(bulb2, HIGH);
digitalWrite(bulb3, HIGH);
lcd.print("MICROCONTROLLERS ");
lcd.setCursor(0,1);
lcd.print(" LAB ");
delay(2000);
lcd.clear();
lcd.print("HOME AUTOMATION ");
lcd.setCursor(0,1);
lcd.print(" USING IR ");
delay(2000);
lcd.clear();
lcd.print("1. Bulb 1");
lcd.setCursor(0,1);
lcd.print("2. Bulb 2");
delay(2000);
lcd.clear();
lcd.print("3. Bulb 3");
delay(2000);
lcd.clear();
lcd.print("Bulb 1 2 3 ");
lcd.setCursor(0,1);
lcd.print(" OFF OFF OFF");
}
void loop() {
lcd.setCursor(0,0);
lcd.print("Bulb 1 2 3 ");
if (irrecv.decode(&results)) {
unsigned int value = results.value;
switch(value) {
case button1:
if(itsON[1] == 1) {
digitalWrite(bulb1, LOW);
itsON[1] = 0;
lcd.setCursor(4,1);
lcd.print("ON ");
}
else {
digitalWrite(bulb1, HIGH);
itsON[1] = 1;
lcd.setCursor(4,1);
lcd.print("OFF");
}
break;
case button2:
if(itsON[2] == 1) {
digitalWrite(bulb2, LOW);
itsON[2] = 0;
lcd.setCursor(8,1);
lcd.print("ON ");
} else {
digitalWrite(bulb2, HIGH);
itsON[2] = 1;
lcd.setCursor(8,1);
lcd.print("OFF");
}
break;
case button3:
if(itsON[3] == 1) {
digitalWrite(bulb3, LOW);
itsON[3] = 0;
lcd.setCursor(12,1);
lcd.print("ON ");
} else {
digitalWrite(bulb3, HIGH);
itsON[3] = 1;
lcd.setCursor(12,1);
lcd.print("OFF ");
}
break;
}
Serial.println(value); // you can comment this line
irrecv.resume(); // Receive the next value
}
}
How the Code Works?
We will start by including all the necessary libraries required for this project as shown below:
#include <LiquidCrystal.h>
#include<string.h>
#include <boarddefs.h>
#include <IRremote.h>
#include <IRremoteInt.h>
#include <ir_Lego_PF_BitStreamEncoder.h>
Next we declare the Arduino pins that are connected with the LCD. To define connections, we use the following line of code. This line creates a LiquidCrystal object and lcd is a name of the object that we are going to use to call LCD functions. You can also use any other name.
LiquidCrystal lcd(rs, en, d4, d5, d6, d7)
In our case, the pins are 8, 9, 10, 11, 12, and 13 respectively.
LiquidCrystal lcd(8, 9, 10, 11, 12, 13);
Specify the Arduino pins connected with IN1, IN2 and IN3 of 4 Channel Relay Module.
#define bulb1 3
#define bulb2 4
#define bulb3 5
Create an array itsON[] that holds the current states of the bulbs. Initially all are OFF.
int itsON[] = {1,1,1,1};
Specify the Arduino pin connected with the receiver module’s output signal pin. It is pin 2 in our case.
int RECV_PIN = 2;
IRrecv irrecv(RECV_PIN);
Define the three buttons with their set values. Here button ‘1’ is defined as 16724175. The button ‘2’ is 16718055 and button ‘3’ is 16743045.
#define button1 16724175
#define button2 16718055
#define button3 16743045
setup()
Inside the setup() function we will first start the initialization to receive IR signals.
irrecv.enableIRIn();
Next, we use lcd.begin() routine to define the size of an LCD. The first argument to this function is a number of rows and the second argument is a number of columns. For instance, this line declares the size as 16 columns and 2 rows. That means 16×2 size.
lcd.begin(16, 2);
Open the serial communication at a baud rate of 9600.
Serial.begin(9600);
Configure bulb1, bulb2, bulb3 pins as output pins by using pinMode() function. Specify the pin as the first parameter and the mode as the second parameter.
pinMode(bulb1, OUTPUT);
pinMode(bulb2, OUTPUT);
pinMode(bulb3, OUTPUT);
Initially set their value to HIGH by using digitalWrite() function. Specify the pin as the first parameter and the value as the second parameter.
digitalWrite(bulb1, HIGH);
digitalWrite(bulb2, HIGH);
digitalWrite(bulb3, HIGH);
Print a series of messages on the LCD display with delays in between. Initially all the three bulbs are off hence it will be displayed on the LCD.
lcd.print("MICROCONTROLLERS ");
lcd.setCursor(0,1);
lcd.print(" LAB ");
delay(2000);
lcd.clear();
lcd.print("HOME AUTOMATION ");
lcd.setCursor(0,1);
lcd.print(" USING IR ");
delay(2000);
lcd.clear();
lcd.print("1. Bulb 1");
lcd.setCursor(0,1);
lcd.print("2. Bulb 2");
delay(2000);
lcd.clear();
lcd.print("3. Bulb 3");
delay(2000);
lcd.clear();
lcd.print("Bulb 1 2 3 ");
lcd.setCursor(0,1);
lcd.print(" OFF OFF OFF");
loop()
Inside the infinite loop() we first check if the decoded received IR signal stored in the variable results is present. If it is then save that signal in the variable ‘value.’ Next we will use a switch statement to check which button was pressed from the remote. If the first button was pressed and bulb1 was OFF then turn it ON. Otherwise keep it OFF. Likewise, if the second button was pressed and bulb2 was OFF then turn it ON. Otherwise keep it OFF. Similarly, if the third button was pressed and bulb3 was OFF then turn it ON. Otherwise keep it OFF. The LCD will display the new states of the bulbs as they change.
void loop() {
lcd.setCursor(0,0);
lcd.print("Bulb 1 2 3 ");
if (irrecv.decode(&results)) {
unsigned int value = results.value;
switch(value) {
case button1:
if(itsON[1] == 1) {
digitalWrite(bulb1, LOW);
itsON[1] = 0;
lcd.setCursor(4,1);
lcd.print("ON ");
}
else {
digitalWrite(bulb1, HIGH);
itsON[1] = 1;
lcd.setCursor(4,1);
lcd.print("OFF");
}
break;
case button2:
if(itsON[2] == 1) {
digitalWrite(bulb2, LOW);
itsON[2] = 0;
lcd.setCursor(8,1);
lcd.print("ON ");
} else {
digitalWrite(bulb2, HIGH);
itsON[2] = 1;
lcd.setCursor(8,1);
lcd.print("OFF");
}
break;
case button3:
if(itsON[3] == 1) {
digitalWrite(bulb3, LOW);
itsON[3] = 0;
lcd.setCursor(12,1);
lcd.print("ON ");
} else {
digitalWrite(bulb3, HIGH);
itsON[3] = 1;
lcd.setCursor(12,1);
lcd.print("OFF ");
}
break;
}
Serial.println(value); // you can comment this line
irrecv.resume(); // Receive the next value
}
}
Video Demonstration
You may like to read: