In this tutorial, you will learn how to assign a fixed or static IP address to ESP32. To assign a static IP address to ESP32, we need to set local IP, gateway, subnet, primary and secondary DNS. You must be wondering what is meant by assigning a static or fixed IP address to EP32. When you create a web server with ESP32, we always access the web server through an IP address. But whenever we restart or reboot ESP32, we possibly get a new IP address. WiFi network usually assigns a new IP address to ESP32 board. Therefore, we can assign a static or fix IP address to our board. Shortly, you will be able to do fix the IP address of ESP32 with a very easy method.
What is an IP Address?
An IP address, short for Internet Protocol address, is a numerical label assigned to each device connected to your router for Internet connectivity. It serves two main purposes: identifying the host or network interface and providing the location of the device in the network. By using an IP address, devices can send and receive data over the Internet, enabling communication between computers, servers, and other networked devices worldwide.
IP addresses are used to facilitate the routing of data packets across the Internet. They consist of a series of numbers separated by periods (e.g., 192.168.0.1) and are typically represented in either IPv4 (Internet Protocol version 4) or IPv6 (Internet Protocol version 6) format.
IP addresses can be either static or dynamic. A static IP address remains fixed and is manually assigned to a device, whereas a dynamic IP address is automatically assigned by a router using DHCP protocol and may change over time.
How DHCP Server Assigns IP Address to Network Devices?
A DHCP server (Dynamic Host Configuration Protocol server) in a Wi-Fi router serves an essential role in managing and allocating IP addresses to devices on a local network. The DHCP server assigns IP addresses dynamically to devices that connect to the Wi-Fi network. When a device connects to the network and requests an IP address, the DHCP server assigns an available address from the pool. Along with IP addresses, the DHCP server can provide additional configuration parameters to devices. These parameters include:
- Subnet mask
- Default gateway
- DNS server addresses
The DHCP server pushes these parameters to connected devices, enabling them to configure their network settings automatically.
To assign a static IP address to ESP32, we need to configure the above parameter settings on the ESP32 manually and the DHCP server will not configure those network settings. Hence, to assign a static IP address we must know the above given parameters before connecting ESP32 to a Wi-Fi network.
How to assign static/Fix IP Address
For an illustration of how to assign a static IP address to ESP32, we use the example of an ESP32 Web server with DHT11/DHT22. We will use the same sketch use in that tutorial except adding the functionality of assigning fix IP address. In order to understand the code given below, I suggest you check the ESP32 Web server with DHT11/DHT22 interfacing tutorial. In this tutorial, I will only explain those functions that are used for assigning fix IP addresses.
#include <WiFi.h>
#include <Wire.h>
#include "DHT.h"
// Uncomment one of the lines below for whatever DHT sensor type you're using!
//#define DHTTYPE DHT11 // DHT 11
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321
//DHT Sensor;
uint8_t DHTPin = 4;
// Initialize DHT sensor.
DHT dht(DHTPin, DHTTYPE);
float Temperature;
float Humidity;
// Replace with your network credentials
const char* ssid = "Enter your WiFi name here"; // Enter SSID here
const char* password = "Enter your WiFi password herer"; //Enter Password here
// Set web server port number to 80
WiFiServer server(80);
// Variable to store the HTTP request
String header;
// it wil set the static IP address to 192, 168, 1, 184
IPAddress local_IP(192, 168, 1, 184);
//it wil set the gateway static IP address to 192, 168, 1,1
IPAddress gateway(192, 168, 1, 1);
// Following three settings are optional
IPAddress subnet(255, 255, 0, 0);
IPAddress primaryDNS(8, 8, 8, 8);
IPAddress secondaryDNS(8, 8, 4, 4);
void setup() {
Serial.begin(115200);
pinMode(DHTPin, INPUT);
dht.begin();
// This part of code will try create static IP address
if (!WiFi.config(local_IP, gateway, subnet, primaryDNS, secondaryDNS)) {
Serial.println("STA Failed to configure");
}
// Connect to Wi-Fi network with SSID and password
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
// Print local IP address and start web server
Serial.println("");
Serial.println("WiFi connected.");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
server.begin();
}
void loop()
{
Temperature = dht.readTemperature(); // Gets the values of the temperature
Humidity = dht.readHumidity(); // Gets the values of the humidity
WiFiClient client = server.available(); // Listen for incoming clients
if (client)
{ // If a new client connects,
String request = client.readStringUntil('\r');
client.println("HTTP/1.1 200 OK");
client.println("Content-type:text/html");
client.println("Connection: close");
client.println();
// Display the HTML web page
client.println("<!DOCTYPE html><html>");
client.println("<head><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">");
client.println("<link rel=\"icon\" href=\"data:,\">");
client.println("<meta http-equiv=\"refresh\" content=\"2\">");
client.println("<script>\n");
client.println("setInterval(loadDoc,200);\n");
client.println("function loadDoc() {\n");
client.println("var xhttp = new XMLHttpRequest();\n");
client.println("xhttp.onreadystatechange = function() {\n");
client.println("if (this.readyState == 4 && this.status == 200) {\n");
client.println("document.getElementById(\"webpage\").innerHTML =this.responseText}\n");
client.println("};\n");
client.println("xhttp.open(\"GET\", \"/\", true);\n");
client.println("xhttp.send();\n");
client.println("}\n");
client.println("</script>\n)");
// CSS to style the table
client.println("<style>body { text-align: center; font-family: \"Arial\", Arial;}");
client.println("table { border-collapse: collapse; width:40%; margin-left:auto; margin-right:auto;border-spacing: 2px;background-color: white;border: 4px solid green; }");
client.println("th { padding: 20px; background-color: #008000; color: white; }");
client.println("tr { border: 5px solid green; padding: 2px; }");
client.println("tr:hover { background-color:yellow; }");
client.println("td { border:4px; padding: 12px; }");
client.println(".sensor { color:white; font-weight: bold; background-color: #bcbcbc; padding: 1px; }");
// Web Page Heading
client.println("</style></head><body><h1>ESP32 Web Server Reading sensor values</h1>");
client.println("<h2>DHT11/DHT22</h2>");
client.println("<h2>Microcontrollerslab.com</h2>");
client.println("<table><tr><th>MEASUREMENT</th><th>VALUE</th></tr>");
client.println("<tr><td>Temp. Celsius</td><td><span class=\"sensor\">");
client.println(dht.readTemperature());
client.println(" *C</span></td></tr>");
client.println("<tr><td>Temp. Fahrenheit</td><td><span class=\"sensor\">");
client.println(1.8 * dht.readTemperature() + 32);
client.println(" *F</span></td></tr>");
client.println("<tr><td>Humidity</td><td><span class=\"sensor\">");
client.println(dht.readHumidity());
client.println(" %</span></td></tr>");
client.println("</body></html>");
client.println();
Serial.println("Client disconnected.");
Serial.println("");
}
}
- Now copy this code to your Arduino IDE.
- Before uploading this code to ESP32 board, you need to enter SSID name and Password in these lines that are your WiFi name and password.
// change it with your WiFi use name and password
const char* ssid = "Enter your WiFi name here"; // Enter SSID here
const char* password = "Enter your WiFi password herer"; //Enter Password here
As mentioned earlier, all code is the same as used in DHT11 interfacing with ESP32 tutorial except the code which adds the functionality of fixing the IP address of ESP32. Now, we will see how to add this functionality.
ESP32 Assigning Static IP Address Code
- Above the setup() function, these lines are used to assign a static IP address to the ESP32 development board.
- PAddress local_IP() functions assign the IP address of 192, 168, 10, 47 through a gateway of 192, 168, 2 2.
- You can choose the IP address and gateway address to whatever value you want according to the available list of IP addresses in your local area network.
- Last three lines set the subnet, primary DNS and secondary DNS for network settings. I recommend you do not change these values unless you really understand the concepts of networking.
- primaryDNS and secondaryDNS functions are optional here. you can delete them if you want.
// it wil set the static IP address to 192, 168, 10, 47
IPAddress local_IP(192, 168, 10, 47);
//it wil set the gateway static IP address to 192, 168, 2,2
IPAddress gateway(192, 168, 2 ,2);
// Following three settings are optional
IPAddress subnet(255, 255, 0, 0);
IPAddress primaryDNS(8, 8, 8, 8);
IPAddress secondaryDNS(8, 8, 4, 4);
Inside the setup() function, WiFi.config() function used to fix the IP address according to above given parameters such as local_IP, gateway, subnet, primaryDNS, secondaryDNS.
If the IP address is available in your local area network, it will assign this IP address. Otherwise, you will see the message “TA Failed to configure” on the serial monitor.
// This part of code will try create static IP address
if (!WiFi.config(local_IP, gateway, subnet, primaryDNS, secondaryDNS))
{
Serial.println("STA Failed to configure");
}
Now your ESP32 sketch is ready to be uploaded. Click on the upload button in your Arduino IDE.
After uploading the code, open the serial monitor of Arduino IDE. You will see this message on a serial monitor which shows you the IP address you assigned.
This is the same IP address that we mentioned inside the sketch and the local area network has assigned the same IP address to the ESP32 board.
Now even if you restart or reboot your ESP32 board, you will always get the same IP address and you can access the ESP32 web server with the same IP address.
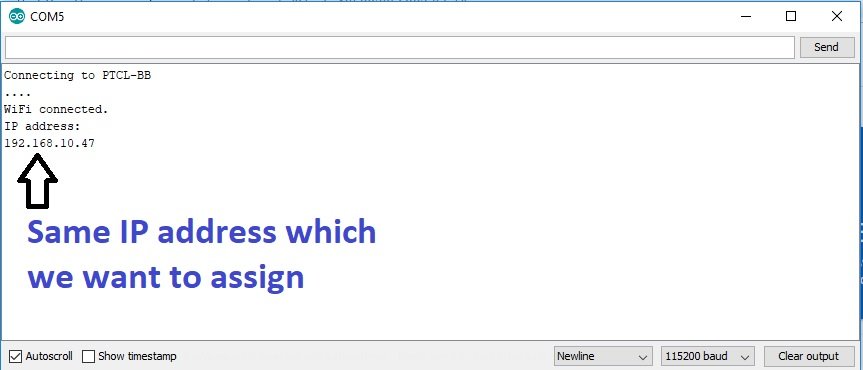
Static IP address library
This section provides details on functions used to assign static IP.
- WiFi.config(): This function is used to configure a fixed IP address and also modify the DNS, gateway, and subnet addresses for ESP32 board. WiFi.begin() function automatically assigns an IP address to devices from the available list of IP addresses in your network.
- If you call WiFi.config() before WiFi.begin(), it will force the .begin to assign IP address according to define IP address inside .config().
Static IP Address Applications
Static IP addresses have various applications in different scenarios. Here are some common use cases for static IP addresses:
ESP Web Servers: Static IP addresses are commonly used for hosting servers such as web servers, FTP servers, email servers, or game servers. A static IP address ensures that the server is always reachable at a consistent address, making it easier for users or clients to connect to the services provided by the server.
Remote Access: Static IP addresses are useful when you need to remotely access devices or networks. For example, if you want to set up remote desktop access to a computer or establish a virtual private network (VPN) connection, a static IP address ensures a stable connection without the need to constantly update IP address settings.
Networked Devices: Certain networked devices or Internet of Things (IoT) devices may require a static IP address for configuration and management purposes. This ensures that the devices are consistently accessible on the network, making it easier to interact with or control them.
Port Forwarding: Static IP addresses are often required for port forwarding on routers. Port forwarding allows external devices or services to connect to specific ports on a device within a local network. By using a static IP address, you can reliably configure port forwarding rules since the IP address remains constant.
Security and Access Control: Static IP addresses can be used for security and access control purposes. For example, in a firewall or network access control system, specific IP addresses may be whitelisted or granted special permissions for enhanced security.
VPN Services: When setting up a virtual private network (VPN) server or subscribing to a VPN service, a static IP address may be required to ensure consistent access and connectivity.
These are just a few examples of how static IP addresses are used in different applications. In general, static IP addresses are advantageous when consistent and reliable connectivity is needed, or when specific network configurations and services rely on fixed IP addresses.
I hope you enjoyed this tutorial, you may also like to check other ESP32 tutorials and projects:
This is exactly what I needed to set a fix IP to my project!
Thank you very much!
after assigning the static ip, server stops responding
you need to check your router settings. There is nothing wrong with the program. Your router settings are not supporting static IP address
It might be worth to make some changes at the router settings? I had been modified my own a long time ago as follow: reduced the DHCP address range leaving a certain range for fixed Ip addressed devices. My default DHCP range was 192.168.1.1/24 – 192.168.1.253/24 while the default gateway (router’s IP) 192.168.1.254/24 and the reduced DHCP range is 192.168.1.1/24 – 192.168.1.200/24. (If somebody don’t know what the /24 means: it’s referring to the (default) masking value as 255.255.255.0
I had the same problem. As a matter fact, there is an issue with the WiFi library that had to be solved but apparently it is not.
The workaround I have implemented and it works is the following:
1. comment the following code:
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(“.”);
}
2. replace it with:
delay (15000);
That means the code does not wait for the WL_CONNECTED status, instead, it waits for 15 seconds and afterwards, it considers connected. It works well most of the times, won’t work well in cases of outages when the router needs some time to boot up.
Dear Mihai
Thanks for posting the solution. We will surely update the article with your recommendation.
Is it the value of “IPAddress subnet(255, 255, 0, 0);” right? The fix local IP address here is a Class C, where the default subnet mask should be 255.255.255.0