In this user guide, we will learn to interface HTU21D Temperature and Humidity Sensor module with ESP32 development module. Firstly, we will introduce you to HTU21D sensor including its pinout, features, and interfacing with the ESP32 board. Secondly, we will install the Adafruit HTU21D library in Arduino IDE to access the sensor data. Lastly, we see two examples to display temperature and humidity values on a serial monitor and on the SSD1306 OLED display.
We have a similar guid with Arduino Uno:
HTU21D Sensor Module Introduction
The HTU21D temperature and sensor module comes in a compact user-friendly size that features the HTU21D chip from MEAS Switzerland. This chip is in fact the temperature and humidity sensor that offers various functions and is extremely simple to use with a microcontroller. The sensor is able to measure temperature readings in the range of -40 to 125°C with a good accuracy of ±0.3°C. Similarly, the HTU21D chip also offers a humidity sensor which offers an operating humidity range of 0-100% with a typical accuracy of 2%.
The GY-213V-HTU21D Sensor Module features a 3.3V voltage regulator and level translator. Therefore, it is very convenient to use with a microcontroller such as Arduino, ESP32, STM32 etc. This sensor is a great choice to be used with microcontrollers for temperature and humidity measurements e.g. thermostats, weather stations, etc. Moreover, due to its low power consumption (less than 0.5mA for measuring sensor data and less than 0.14µA when in sleep mode) it is very suitable to use in devices that use a battery.

The HTU21D Sensor Module is shown below which encases the HTU21D sensor chip and built-in 4.7k ohm pull-up resistors for I2C communication. It is a 3.3V sensor which uses inline logic level converters or 10k resistors to limit the 5V signals.
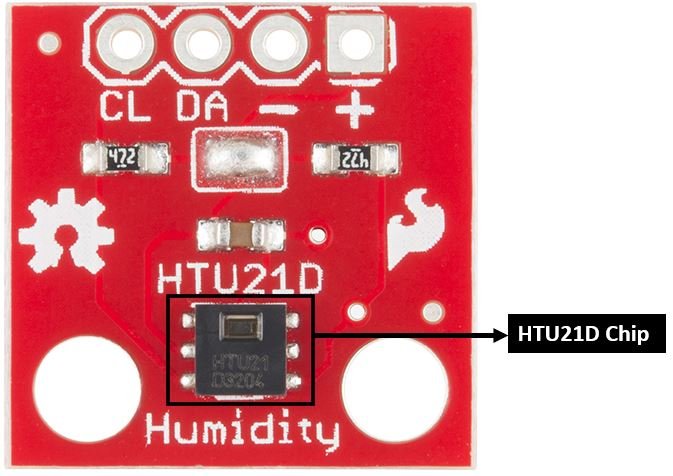
I2C Interface
The HTU21D Sensor Module uses I2C communication protocol to transmit sensor data to the microcontroller. The sensor module features the SCL (serial clock) and SDA (serial data) pins which connect with the I2C interface of ESP32 or other sensors supporting the same interface.
The HTU21D sensor module however comes with a single fixed I2C addresses which is 0x40. In order to communicate with several HTU21D sensors on the same bus, a multiplexer is needed.
To use multiple I2C devices on the same bus, the 4.7k ohm resistors on the HTU21D sensor module are disabled.
HTU21D Sensor Module Pinout
The following figure shows the pinout diagrams of both types of HTU21D sensor modules available in the market. Both consist of four pins.
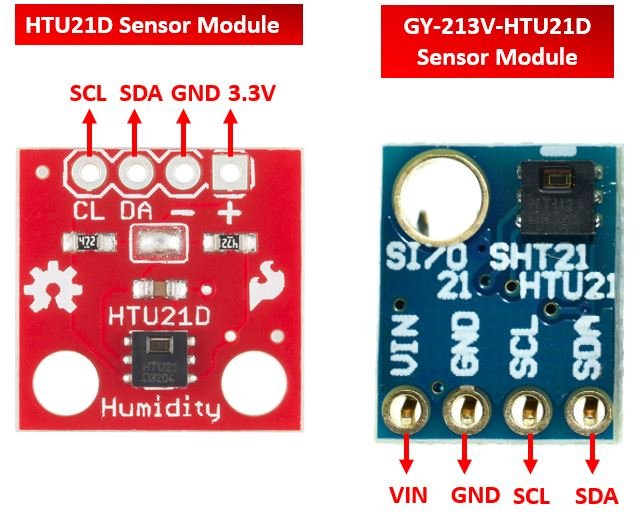
The following lists the pinout of the HTU21D sensor module and their brief description.
HTU21D Module Pin | Description |
---|---|
VIN | This is the pin that supplies power to the sensor module. Connect it with 3.3V in case of HTU21 Sensor Module and 3.3V or Vin pin of ESP32 incase of GY-213V-HTU21D Sensor Module. |
GND | This is the ground pin for providing the common ground between the devices. |
SCL | This is the serial clock pin which generates the clock signal. |
SDA | This is the serial data pin which is used to send and receive data |
Specifications
The table below shows the specifications of the HTU21D temperature and humidity sensor.
Power Supply | 3.3-5.5V (for GY-213V-HTU21D Sensor Module) 1.5-3.6V (for HTU21 Sensor Module) |
Humidity Range | 0 – 100 %RH |
Humidity Accuracy | ±2% over the range of 5% to 95% RH |
Temperature Range | -40˚C to +125˚C |
Temperature Accuracy | ±0.3˚C at 25°C |
Response time | 5s |
Measurement Time | 50ms |
Interface HTU21D Module with ESP32
In this section, let us show you how to connect the HTU21D sensor module with ESP32 board.

You will need the following components
- ESP32 development board
- HTU21D Sensor Module
- Jumper wires
The connection of HTU21D with the ESP32 board is super simple. Connect the VCC terminal of HTU21D module with 3.3V of ESP32, ground with the ground (common ground), SCL of the sensor with SCL pin of ESP32, and SDA of the sensor with the SDA pin of ESP32.
The I2C pin in ESP32 for SDA is GPIO21 and for SCL is GPIO22. The connections between the two devices can be seen in the table below.
ESP32 | HTU21D Module |
---|---|
3.3V | Vin |
GPIO21 | SDA |
GPIO22 | SCL |
GND | GND |
Follow the schematic diagram below for the ESP32 module and connect them accordingly.

Install HTU21D Arduino Library
We will use Arduino IDE to program our ESP32 development board. Thus, you should have the latest version of Arduino IDE. Additionally, you also need to install the ESP32 plugin. If your IDE does not have the plugin installed you can visit the link below:
Installing ESP32 library in Arduino IDE and upload code.
As we are connecting the HTU21D sensor module with ESP32, therefore, we will have to install the libraries to access the sensor data. We will require two libraries for this project:
- Adafruit HTU21D
- Adafruit BusIO
We will use the Library Manager in our Arduino IDE to install the latest versions of the libraries. Open your Arduino IDE and go to Sketch > Include Libraries > Manage Libraries. Type HTU21D in the search bar and install the latest version.

Type Adafruit BusIO in the search bar and install it as well.

Arduino Code – Get HTU21D Readings on Arduino Serial Monitor
This sketch displays current temperature and humidity readings on Arduino serial monitor after every half second.
#include <Wire.h>
#include "Adafruit_HTU21DF.h"
Adafruit_HTU21DF htu = Adafruit_HTU21DF();
void setup() {
Serial.begin(115200);
if (!htu.begin()) {
Serial.println("Check circuit. HTU21D not found!");
while (1);
}
}
void loop() {
float temp = htu.readTemperature();
float hum = htu.readHumidity();
Serial.print("Temperature(°C): ");
Serial.print(temp);
Serial.print("\t\t");
Serial.print("Humidity(%): ");
Serial.println(hum);
delay(500);
}
How the Code Works?
Now, let us understand how each part of the code works.
The code starts with including all the necessary libraries which are needed for the proper functionality of the code. The Wire.h will allow us to communicate through the I2C protocol. The Adafruit_HTU21DF.h library will enable us to initialize and read the HTU21D sensor.
#include <Wire.h>
#include "Adafruit_HTU21DF.h"
Next we create the Adafruit_HTU21DF object called ‘htu.’
Adafruit_HTU21DF htu = Adafruit_HTU21DF();
setup()
Inside the setup() function, we start the serial communication at a baud rate of 115200 and then initialize the HTU21D sensor. If the sensor initialization is not a success, a relevant message will be printed on the serial monitor.
void setup() {
Serial.begin(115200);
if (!htu.begin()) {
Serial.println("Check circuit. HTU21D not found!");
while (1);
}
}
loop()
Inside the loop() function, the ESP32 first obtains the HTU21D sensor readings after every half second and stores them in their respective float variables, ‘temp’ for temperature and ‘hum’ for humidity. Temperature readings are acquired through htu.readTemperature(). Humidity readings are accessed using the htu.readHumidity() function. These readings are printed in the ESP-IDF terminal.
void loop() {
float temp = htu.readTemperature();
float hum = htu.readHumidity();
Serial.print("Temperature(°C): ");
Serial.print(temp);
Serial.print("\t\t");
Serial.print("Humidity(%): ");
Serial.println(hum);
delay(500);
}
Demonstration
Choose the correct board and COM port before uploading your code to the ESP32 board. Go to Tools > Board and select ESP32 Dev Module.
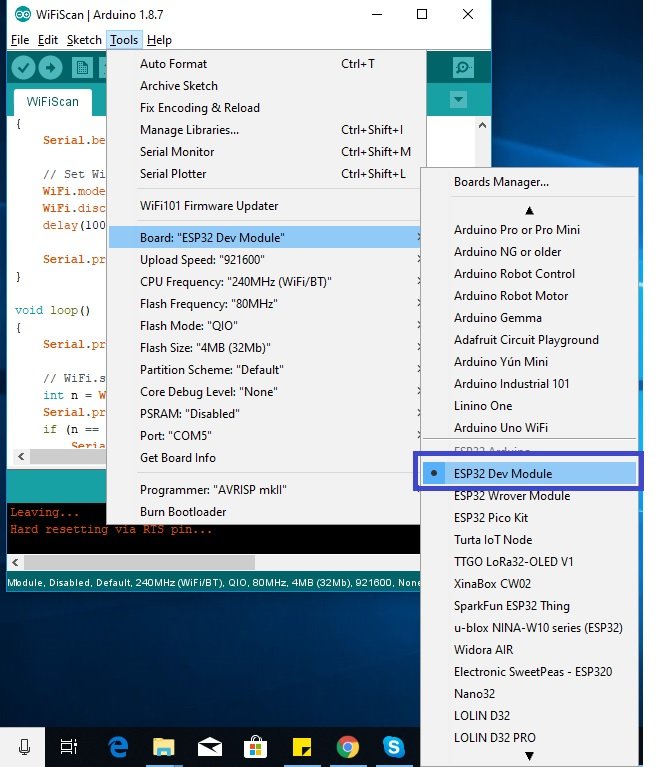
Next, go to Tools > Port and select the appropriate port through which your board is connected.
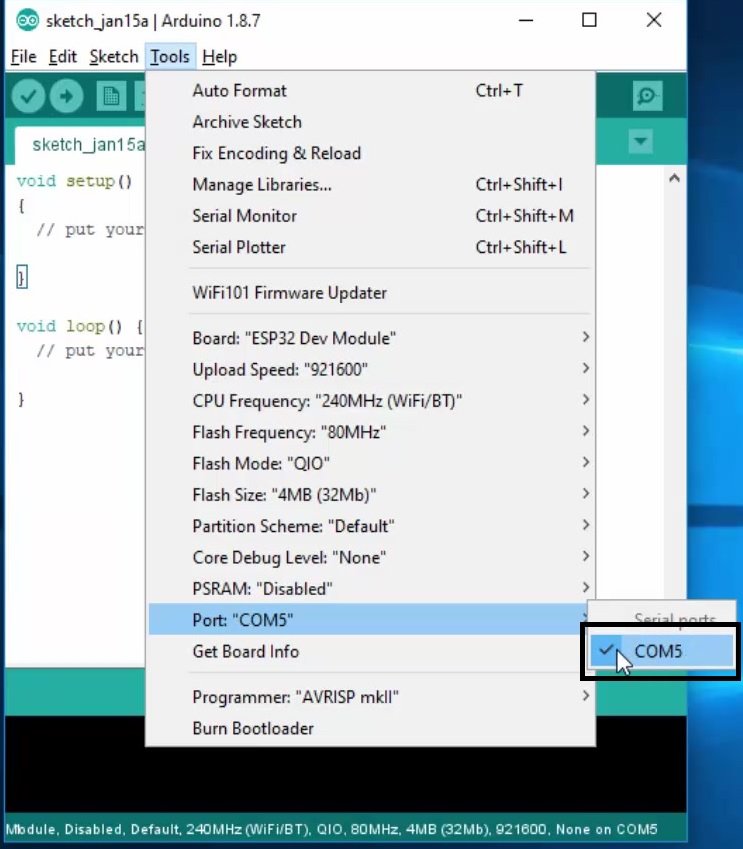
Click on the upload button to upload the code into the ESP32 board. After you have uploaded your code to the board press its ENABLE button.
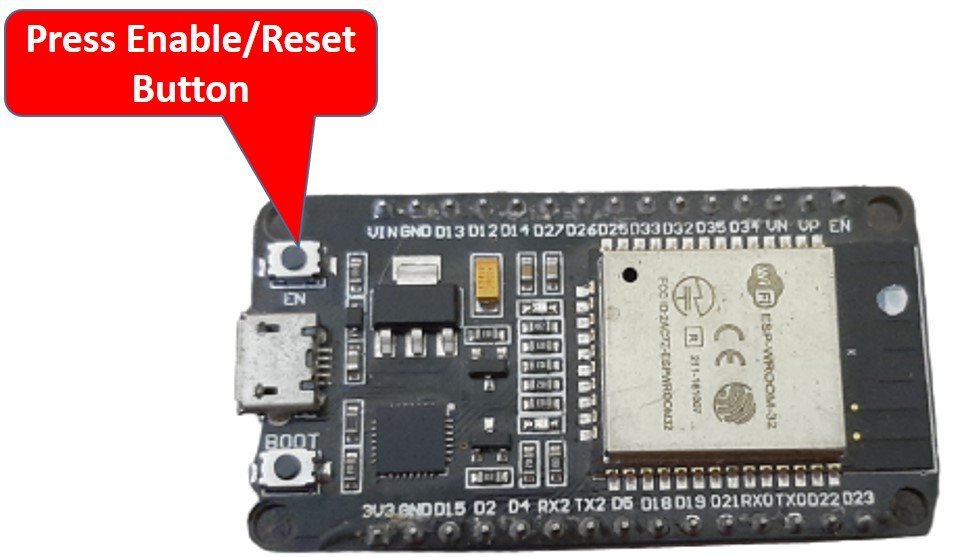
In your Arduino IDE, open up the serial monitor and set the baud rate to 115200. The serial monitor will start displaying the temperature and humidity readings along with the units. New readings will be displayed after every half second.

Display HTU21D Sensor values on OLED Display
In this section, we will see how to display HTU21D sensor readings on a 0.96 SSD1306 OLED display using Arduino IDE and ESP32.
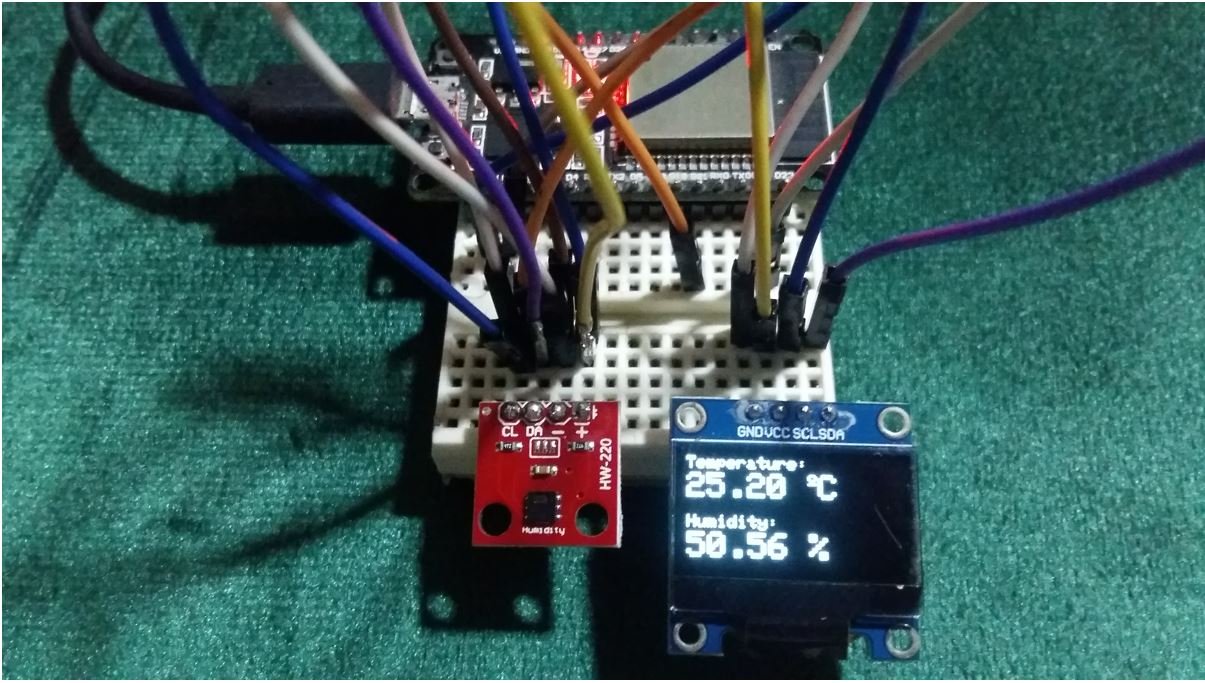
Installing SSD1306 OLED Library in Arduino IDE
To use the OLED display in our project, we have to install the Adafruit SSD 1306 library in Arduino IDE. Follow the steps below to successfully install it.
Open Arduino IDE and click on Sketch > Library > Manage Libraries. The following window will open up.
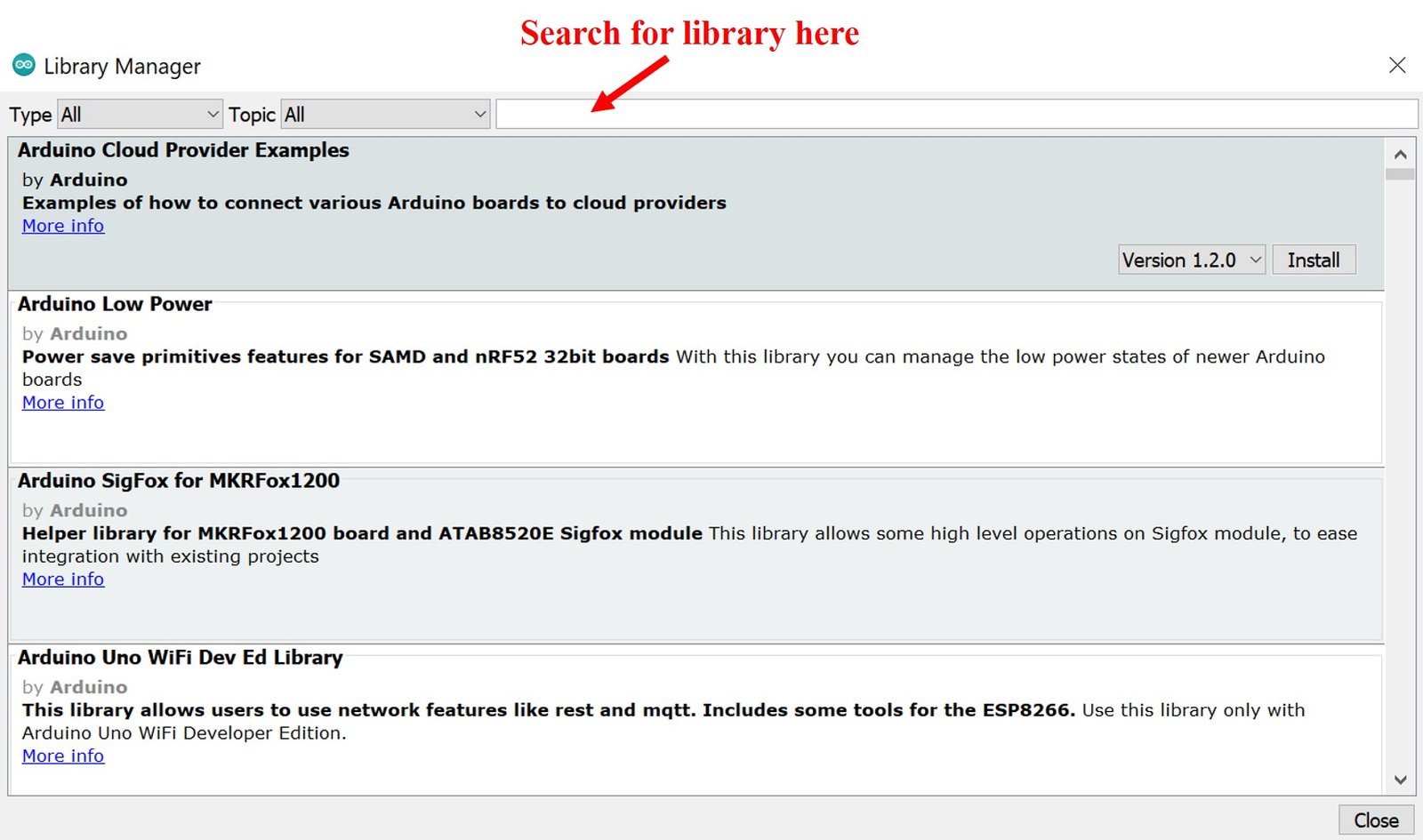
Type ‘SSD1306’ in the search tab and install the Adafruit SSD1306 OLED library.

Schematic – OLED with ESP32 and HTU21D

You will need the following components
- ESP32 development board
- HTU21D Sensor Module
- SSD1306 OLED
- Breadboard
- Jumper wires
The table below shows the terminals of the three devices which should be connected together.
OLED Display | ESP32 | HTU21D Sensor Module |
---|---|---|
VCC | 3.3V | VIN |
GND | GND | GND |
SCL | GPIO22 | SCL |
SDA | GPIO21 | SDA |
Assemble the circuit as shown in the schematic diagram below:

As you can see above, we have connected all the VCC terminals with 3.3V pin of ESP32. The SCL terminals are connected with GPIO22 and the SDA terminals are connected with GPIO21. The grounds are also common.
Arduino Sketch to Display HTU21D Readings on OLED
Copy the following code to your Arduino IDE and upload it to the ESP32 board after assembling the above circuit diagram.
#include <Wire.h>
#include "Adafruit_HTU21DF.h"
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
Adafruit_HTU21DF htu = Adafruit_HTU21DF();
Adafruit_SSD1306 display = Adafruit_SSD1306(128, 64, &Wire, -1);
void setup() {
Serial.begin(115200);
if (!htu.begin()) {
Serial.println("Check circuit. HTU21D not found!");
while (1);
}
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
// init done
display.display();
delay(100);
display.clearDisplay();
display.display();
display.setTextColor(WHITE);
}
void loop() {
float temp = htu.readTemperature();
float hum = htu.readHumidity();
Serial.print("Temperature(°C): ");
Serial.print(temp);
Serial.print("\t\t");
Serial.print("Humidity(%): ");
Serial.println(hum);
display.setCursor(0,0);
display.clearDisplay();
display.setTextSize(1);
display.setCursor(0,0);
display.print("Temperature: ");
display.setTextSize(2);
display.setCursor(0,10);
display.print(temp);
display.print(" ");
display.setTextSize(1);
display.cp437(true);
display.write(167);
display.setTextSize(2);
display.print("C");
// display humidity
display.setTextSize(1);
display.setCursor(0, 35);
display.print("Humidity: ");
display.setTextSize(2);
display.setCursor(0, 45);
display.print(hum);
display.print(" %");
display.display();
delay(500);
}
How the Code Works?
The maximum portion of the code is the same as we have discussed earlier except for the OLED display part. Therefore, we will only explain the OLED display part here.
We will first include all the required libraries for the HTU21D sensor as well as the OLED display which we just installed before.
#include <Wire.h>
#include "Adafruit_HTU21DF.h"
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
Now, we will create another object named ‘display’ which will be handling the OLED display. Also, define the size of the OLED display by passing arguments to the Adafruit_SSD1306() function.
Adafruit_SSD1306 display = Adafruit_SSD1306(128, 64, &Wire, -1);
Initialize the OLED display by calling the begin() method on the display object.
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
Next, we will clear the OLED screen by calling clearDisplay() function. Also, we set the color of the text using setTextColor() function and pass WHITE as an argument. If we have a dark background, we will display our text in white and if we have a bright background then we will display the text in black.
display.clearDisplay();
display.display();
display.setTextColor(WHITE);
Display Readings on the OLED
Inside the loop() function, we obtain the HTU21D sensor readings and print them on the OLED display.
We display the temperature and humidity values on the serial monitor on the OLED one by one. This block of code displays HTU21D sensor values on OLED and update the values after every half second.
display.setCursor(0,0);
display.clearDisplay();
display.setTextSize(1);
display.setCursor(0,0);
display.print("Temperature: ");
display.setTextSize(2);
display.setCursor(0,10);
display.print(temp);
display.print(" ");
display.setTextSize(1);
display.cp437(true);
display.write(167);
display.setTextSize(2);
display.print("C");
// display humidity
display.setTextSize(1);
display.setCursor(0, 35);
display.print("Humidity: ");
display.setTextSize(2);
display.setCursor(0, 45);
display.print(hum);
display.print(" %");
display.display();
delay(500);
In the above code, the setTextSize() function is used to set the size of the font. We used low size for simple text such as “Temperature” and “Humidity” and high size font to display actual temperature and humidity readings. We will use the setCursor() function to denote the x and the y-axis position from where the text should start. Finally, the print() function writes the text on the defined position.
Demonstration
Choose the correct board and COM port before uploading your code to the ESP32 board. Go to Tools > Board and select ESP32 Dev Module.
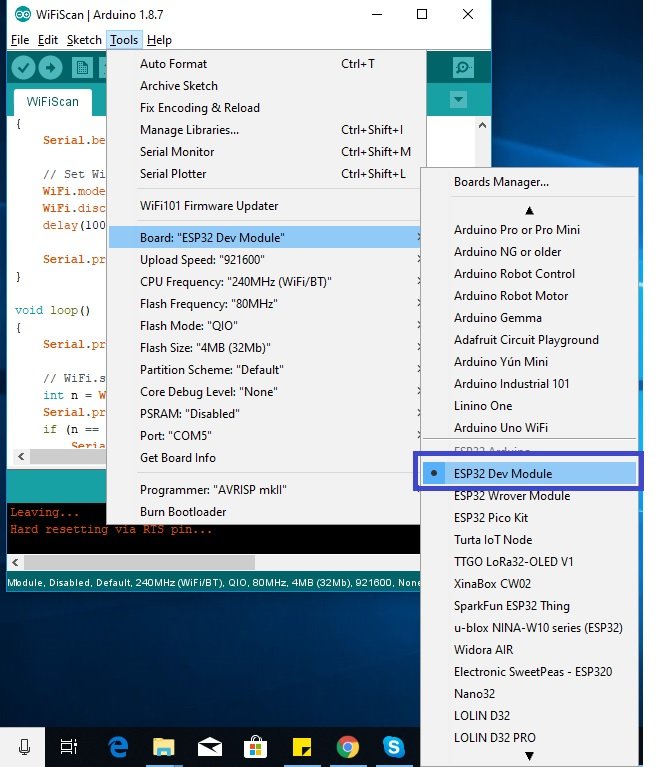
Next, go to Tools > Port and select the appropriate port through which your board is connected.
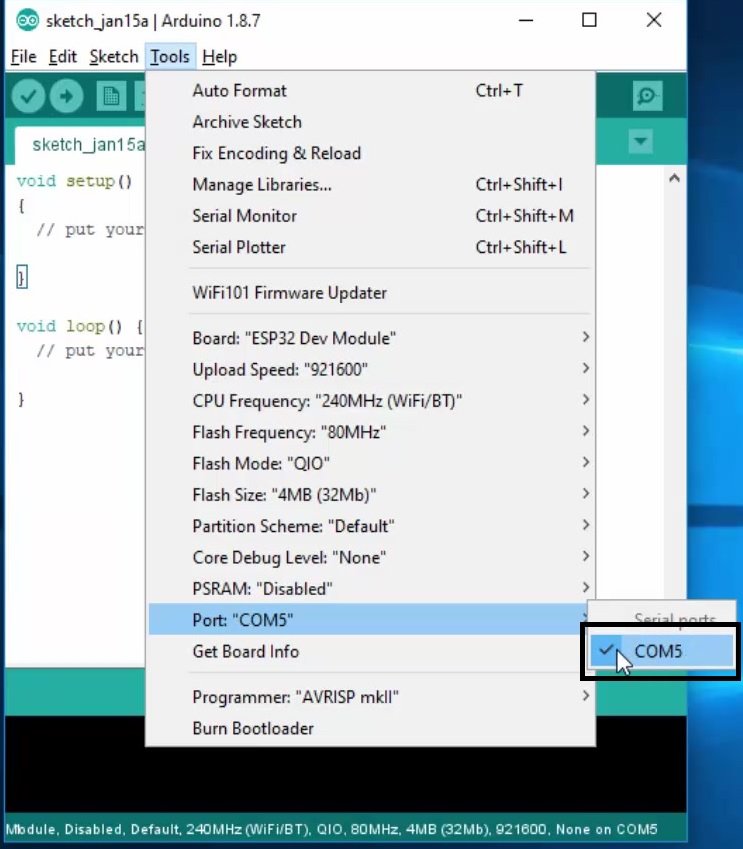
Click on the upload button to upload the code into the ESP32 board. After you have uploaded your code to the board press its ENABLE button.
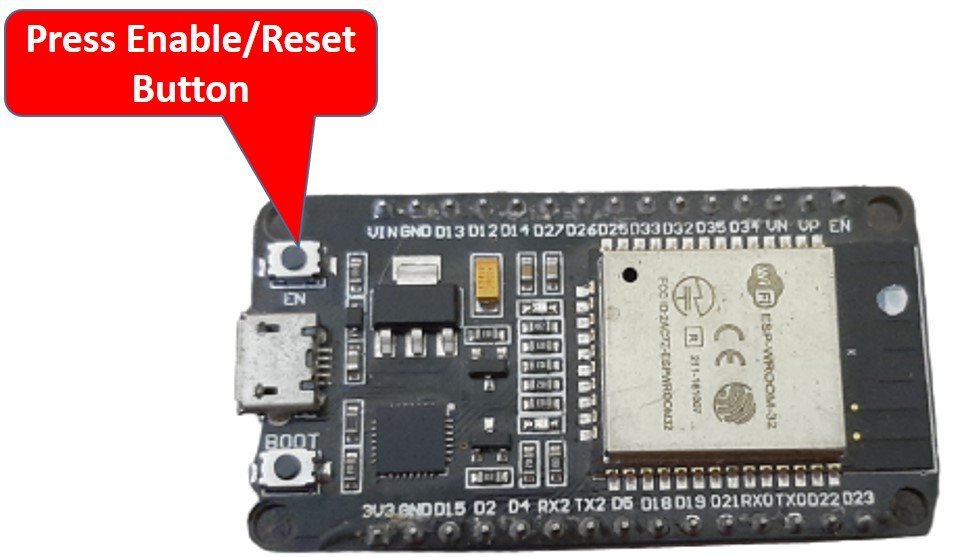
Once the code is successfully uploaded to the board, the OLED will start displaying the readings.
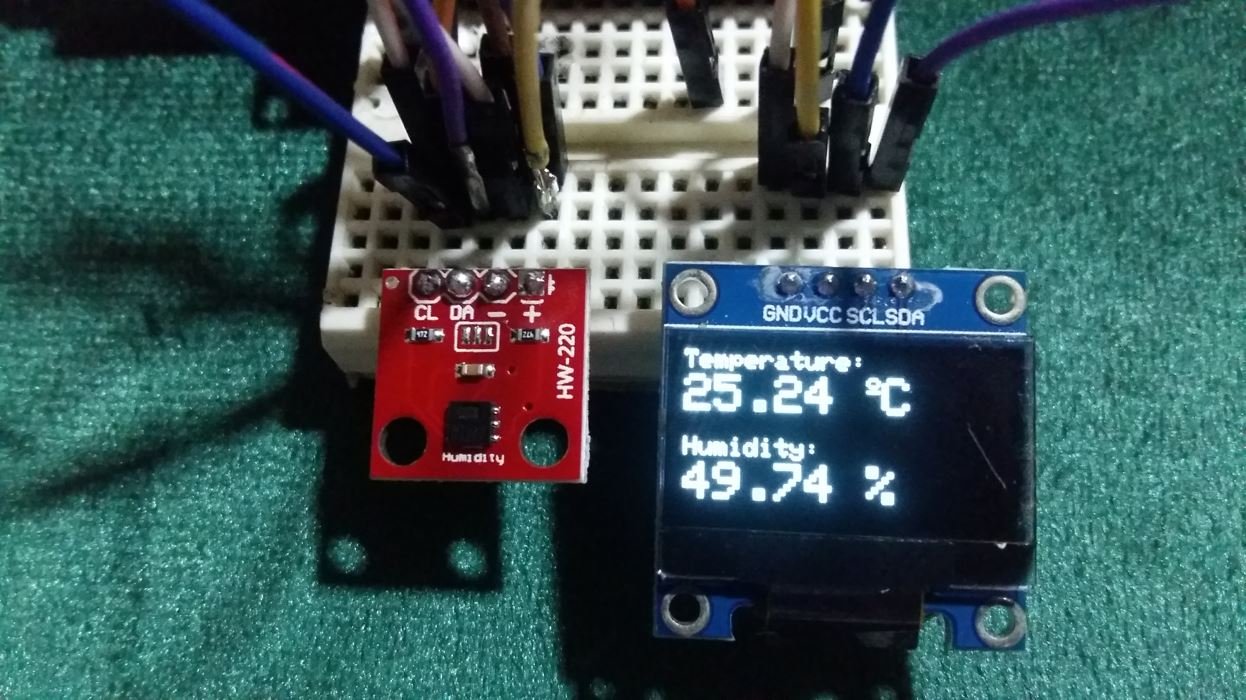
Video demo:
You may also like to read:
- Interface SHT31 Temperature & Humidity Sensor with ESP32
- ADS1115 I2C external ADC with ESP32 in Arduino IDE
- ESP32 with MPU6050 Accelerometer, Gyroscope, and Temperature Sensor ( Arduino IDE)
- BME680 with ESP32 using Arduino IDE (Gas, Pressure, Temperature, Humidity)
- Single and Multiple DS18B20 with ESP32: Display Readings on OLED
- BME280 with ESP32 – Display Values on OLED ( Arduino IDE)
- DHT11 DHT22 with ESP32 – Display Readings on OLED
je suis très intéressé, par ces merveilleux Tutos.