In this tutorial, we will learn how to interface MAX6675 thermocouple module with Arduino and how to use it to measure temperature. The guide includes a brief introduction of the module with its pinout and connection diagram with Arduino. Additionally, we will show you two Arduino sketches where we will be able to access the temperature readings from this module and display them on the Serial Monitor as well as on an OLED.
MAX6675 Thermocouple
The MAX6675 module comes with a K-type thermocouple along with a driver and amplifier used to measure temperature in the range of 0°C to 1024°C. This is one of the ideal choices for measuring higher temperature readings which the other temperature sensors such as LM35 and others. This module consists of the MAX6675 chip which measures the temperature via the K-type thermocouple. The module uses an SPI interface to communicate with the microcontroller and transfer the output temperature readings.
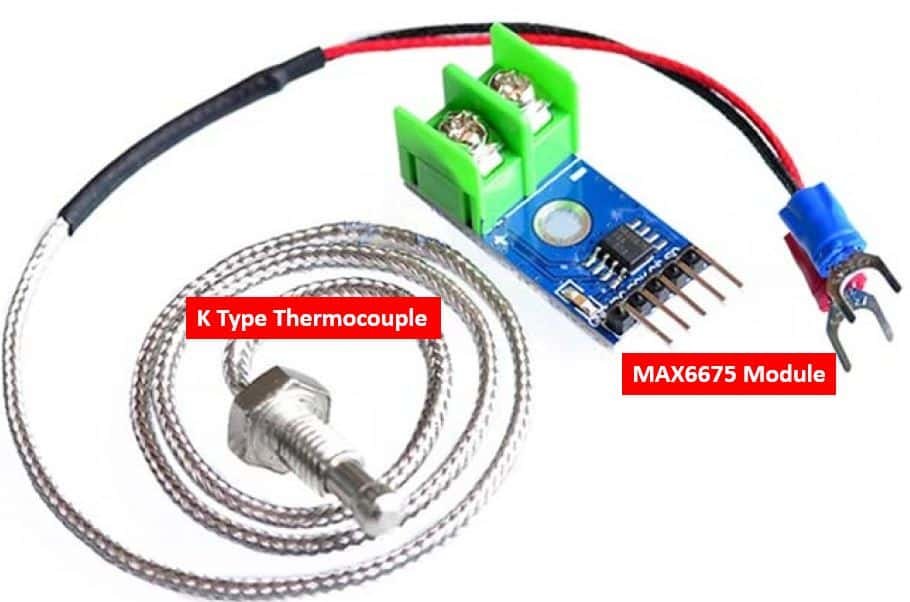
Below you can view the specifications of this module:
- 12- bit resolution
- Accuracy of 8 LSBs
- Resolution of 0.25°C
- Operating Voltage is 5V
- Operating Current is 50mA
Thermocouple
The thermocouple is made with two different material wires. One end of these wires is connected together for making the junction. This junction is placed in that specific environment or object whose temperature we want to measure. When the temperature is changed then two different materials start to deform, as a result, the resistance is changed. Actually, its output is a millivolt signal whose voltages are changed when resistance is changed. This change in voltages could be measured easily with the help of thermocouples.
Working Principle
The working principle of Thermocouple is based on the Seebect effect. According to Seebeck, when two different metals are connected together for making two junctions then electromotive force would be developed at two junctions. The amount of this force would be different with metal material combinations.

According to the figure above, two different metals such as metal A and B are connected together for making two junctions. They are called ‘measuring end’ and ‘reference end’. Remember, two junctions are necessary for making any thermocouple. Temperature of the reference end is known but the measuring end is unknown. When this unknown temperature end is placed at that place where we want to measure the temperature. In this condition, if both ends would be at the same temperature level then there would be no EMF generated. So the net current in the whole circuit would be also zero.
Similarly, if both ends are at different temperature level then the EMF would generate and current will be also flowing in the whole circuit. The value of this EMF or current also depends on thermocouple metal material as well as the temperature of both ends. By measuring the value of this current or EMF the user can easily find out the temperature of that specific place.
There are eight different types of thermocouples currently in the market with respect to their material. We use K Type Thermocouple with MAX6675 module. These K-type thermocouples (Nickel-Chromium / Nickel-Alumel) are less costly, more accurate, more reliable, and the most common type thermocouple. It has a wide range of temperatures such as –454 to 2,300F.
Pinout MAX6675
The diagram below shows the pinout of MAX6675 module:

It consists of a total of 7 pins where two are used to connect the thermocouple positive and negative leads, three for the SPI interface, and two for the power and ground.
Pin Name | Function |
---|---|
GND | This is the ground pin of the module. |
VCC | This is the VCC pin used to supply power to the module in the range of 3-5.5V |
SCK | This is the SPI clock pin. |
CS | This is the SPI Chip select pin. |
SO | This is the serial output pin (data out). |
Thermocouple+ (TH+) | This is where we will connect the positive lead of the thermocouple. |
Thermocouple- (TH-) | This is where we will connect the negative lead of the thermocouple. |
Interfacing MAX6675 Thermocouple with Arduino
We will require the following components for this project.
Required Components:
- Arduino
- MAX6675 module with thermocouple
- Connecting Wires
Assemble the devices as shown in the schematic diagram below:

We will connect 5 pins of the MAX6675 module with Arduino. These include the VCC, GND, SCK, CS and SO pins present on the same side. The VCC will be connected with the 5V pin from the Arduino. GND of both the devices will be in common. The default SPI GPIO pins of Arduino are being used to connect with each of the remaining SPI terminals of the MAX6675 module. The figure below shows the default SPI pins of Arduino.

The table below shows the connections between Arduino and the MAX6675 module:
Arduino | MAX6675 |
GND | GND |
5V | VCC |
GPIO13 | SCK |
GPIO10 | CS |
GPIO12 | SO |
Now follow the connections between the two devices and connect them accordingly. We have used the same connections as specified above. All devices will have their grounds in common.
The thermocouple will be connected at the TH+ and TH- terminals.
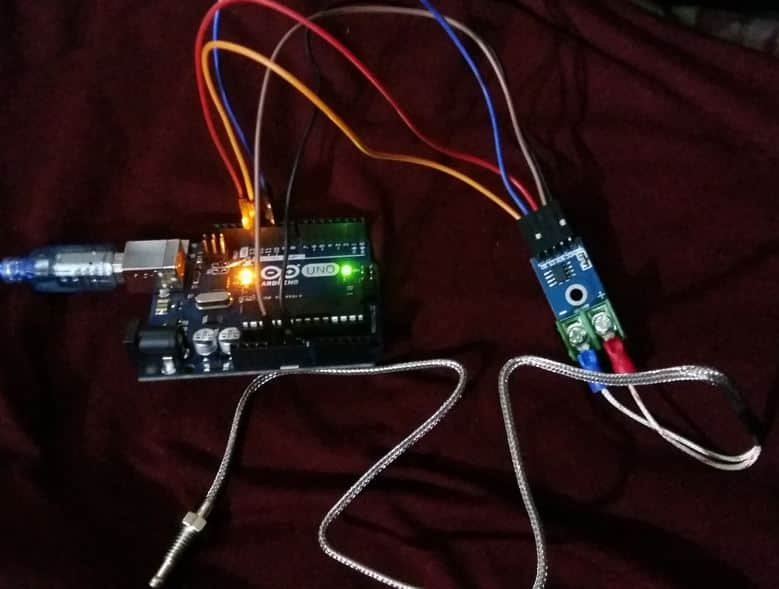
Installing MAX6675 Thermocouple Library
We have an Arduino library for MAX6675 which can be easily used to get values of temperature. Now let’s see how to install the MAX6675 library in Arduino. This library is provided by Adafruit.
To install the library, we will use the Arduino Library Manager. Open your Arduino IDE and go to Sketch > Include Libraries > Manage Libraries. Type ‘max6675’ in the search bar and install the latest version.

Arduino Sketch: Accessing Temperature Readings
Open your Arduino IDE and go to File > New. A new file will open. Copy the code given below in that file and save it.
This code will use max6675.h library provided by Adafruit. We will display the temperature (degree Celsius) in the Serial Monitor.
#include "max6675.h"
int SO = 12;
int CS = 10;
int sck = 13;
MAX6675 module(sck, CS, SO);
void setup() {
Serial.begin(115200);
}
void loop() {
float temperature = module.readCelsius();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(F("°C "));
delay(1000);
}
How the Code Works?
We will start off by including the MAX6675 library that we previously installed.
#include "max6675.h"
Then we will create three integer variables each to hold the GPIO pins connected with the SPI pins: SO, SCK and CS. We have used the Arduino default SPI pins.
int SO = 12;
int CS = 10;
int sck = 13;
Next we will create an instance of MAX6675 called module() and pass the sck, CS and SO variables as parameters inside it.
MAX6675 module(sck, CS, SO);
Inside the setup() function, we will initialize the serial communication with the baud rate of 115200
void setup() {
Serial.begin(115200);
}
Inside the loop() function, we will first obtain the temperature by readCelsius() method on the module instance and save the reading in a floating variable called ‘temperature.’ This will get printed in the serial monitor along with its unit after a delay of every 1 second.
void loop() {
float temperature = module.readCelsius();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(F("°C "));
delay(1000);
}
Demonstration
Now upload the Arduino sketch to the Arduino board. Choose the correct board and COM port before uploading your code to the Arduino board. Go to Tools > Board and select Arduino Module.
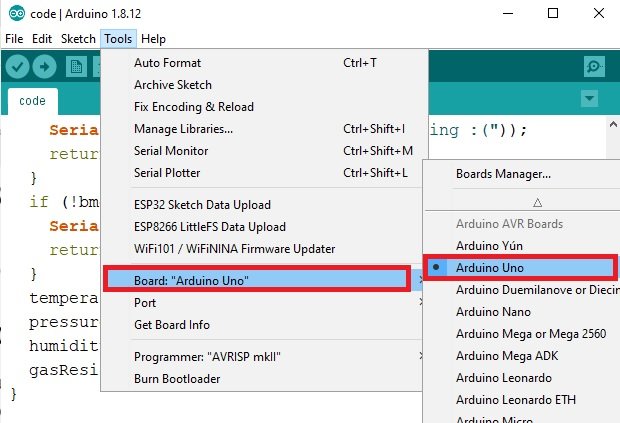
Next, go to Tools > Port and select the appropriate port through which your board is connected.
Click on the upload button to upload the code into the Arduino board. After you have uploaded your code to the board, press its RESET button.
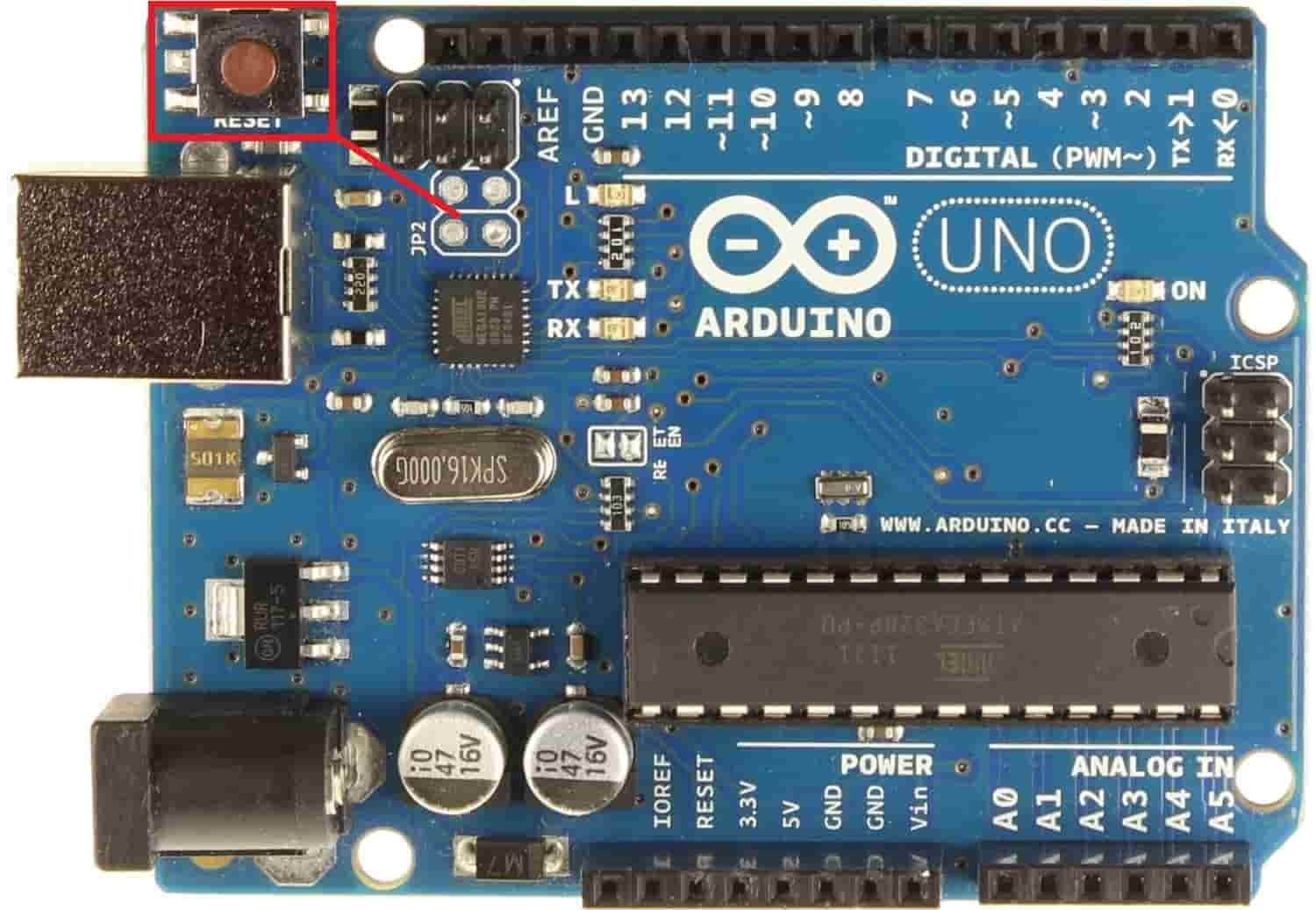
Now open the serial monitor in Arduino IDE, you will see temperature readings getting displayed after every one second.

Arduino Display MAX6675 Thermocouple Readings on OLED
In this section, we will see how to display the temperature readings of MAX6675 on a 0.96 SSD1306 OLED display using Arduino IDE and Arduino.
Installing SSD1306 OLED Library in Arduino IDE
To use the OLED display in our project, we have to install the Adafruit SSD1306 OLED library in Arduino IDE. Follow the steps below to successfully install it.
Open Arduino IDE and click on Sketch > Library > Manage Libraries. Type ‘SSD1306’ in the search tab and install the Adafruit SSD1306 OLED library.

We will also require the Adafruit GFX library which is a dependency for SSD1306. Type ‘Adafruit GFX’ in the search tab and install it as well.
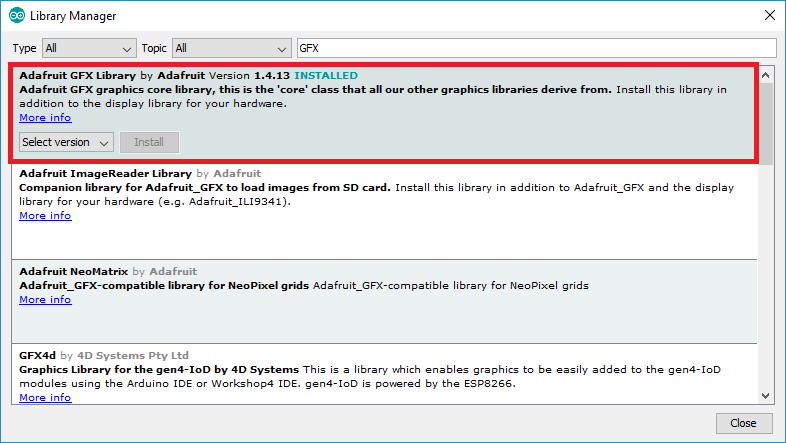
You may like this in-depth guide on OLED interfacing with Arduino:
Schematic – OLED with Arduino and MAX6675 Thermocouple
This section shows how to connect an Arduino board with MAX6675 and an OLED display. Follow the connections as described in the two tables below.
The tables below show the terminals of the three devices which should be connected together.
OLED Display | Arduino |
VCC | 5V |
GND | GND |
SCL | A5 |
SDA | A4 |
Arduino | MAX6675 K-type thermocouple |
GND | GND |
5 V | VCC |
GPIO10 | CS |
GPIO12 | SO |
GPIO13 | SCK |
Assemble the circuit as shown in the schematic diagram below:

We will connect the VCC terminal of the OLED display with 5V which will be in common with the Arduino board and MAX6675 VCC. SCL of the display will be connected with the SCL pin of the Arduino board and the SDA of the display will be connected with the SDA of the Arduino.

Arduino Sketch to Display MAX6675 Readings on OLED
Copy the following code to your Arduino IDE and upload it to the Arduino after assembling the above circuit diagram.
#include "max6675.h"
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
int SO = 12;
int CS = 10;
int sck = 13;
MAX6675 module(sck, CS, SO);
Adafruit_SSD1306 display = Adafruit_SSD1306(128, 64, &Wire, -1);
void setup() {
Serial.begin(115200);
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
delay(2000);
display.clearDisplay();
display.setTextColor(WHITE);
}
void loop() {
float temperature = module.readCelsius();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(F("°C "));
display.setTextSize(1);
display.setCursor(0,10);
display.print("Temperature: ");
display.setTextSize(2);
display.setCursor(0,30);
display.print(temperature);
display.print(" ");
display.setTextSize(1);
display.cp437(true);
display.write(167);
display.setTextSize(2);
display.print("C");
display.display();
delay(1000);
display.clearDisplay();
}
How the Code Works?
We will first include all the required libraries for MAX6675 as well as the OLED display which we just installed before.
#include "max6675.h"
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
Then we will create three integer variables each to hold the GPIO pins connected with the SPI pins: SO, SCK and CS. We have used the Arduino default SPI pins.
int SO = 12;
int CS = 10;
int sck = 13;
Next we will create an instance of MAX6675 called module() and pass the sck, CS and SO variables as parameters inside it.
MAX6675 module(sck, CS, SO);
Now, we will create an object named display which will be handling the OLED display and specifying the width, height, I2C instance (&Wire), and -1 as parameters inside it.’ -1′ specifies that the OLED display which we are using does not have a RESET pin. If you are using the RESET pin then specify the GPIO through which you are connecting it with your development board.
Adafruit_SSD1306 display = Adafruit_SSD1306(128, 64, &Wire, -1);
setup()
Inside the setup() function, we will initialize the serial communication with the baud rate of 115200
Serial.begin(115200);
Moreover, we will also initialize the OLED display by using display.begin(). Make sure you specify the correct address of your display. In our case, it is 0X3C.
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
delay(2000);
Then, we will clear the buffer by using clearDisplay() on the Adafruit_SSD1306 object. Additionally, we will set the colour of the text as white.
display.clearDisplay();
display.setTextColor(WHITE);
loop()
Inside the loop() function, we will first obtain the temperature by readCelsius() method on the module instance and save the reading in a floating variable called ‘temperature.’ This will get printed in the serial monitor along with its unit after a delay of every 1 second.
float temperature = module.readCelsius();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(F("°C "));
The setTextSize() function is used to set the size of font. We used low size for simple text such as “Temperature” and high size font to display actual temperature readings. The setCursor() method defined where we want to display our text on 128×64 OLED. Finally, the print() functions write the text on the defined position. We will display the updated temperature reading along with its unit on the OLED screen after every second.
display.setTextSize(1);
display.setCursor(0,10);
display.print("Temperature: ");
display.setTextSize(2);
display.setCursor(0,30);
display.print(temperature);
display.print(" ");
display.setTextSize(1);
display.cp437(true);
display.write(167);
display.setTextSize(2);
display.print("C");
display.display();
delay(1000);
display.clearDisplay();
Demonstration
To see the demonstration of the above code, upload the code to Arduino. But, before uploading code, make sure to select the Arduino from Tools > Board and also select the correct COM port to which the Arduino board is connected from Tools > Port.
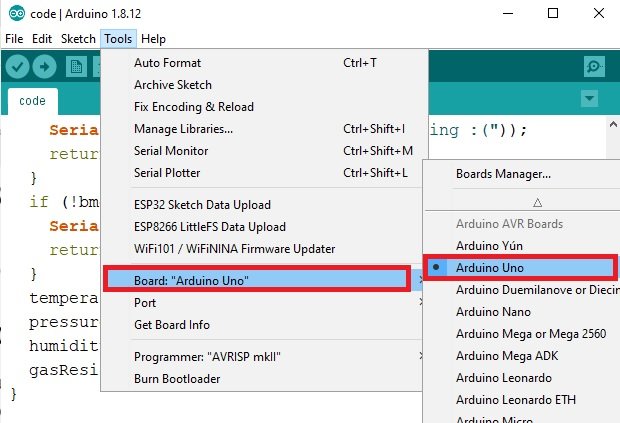
Once the code is uploaded to Arduino, the OLED will start displaying the temperature readings in degrees Celsius after every second.

Video demo:
Other sensors guides with Arduino:
- TCS230/TCS3200 Color Sensor with Arduino
- Rotary Encoder Module with Arduino
- HC-SR04 Ultrasonic Sensor Interfacing with Arduino
- Interfacing Multiple DS18B20 with Arduino: Display Readings on OLED
- DHT11 DHT22 with Arduino
- Interface TMP36 Temperature Sensor with Arduino
- BME280 with Arduino: Display Readings on OLED ( Arduino IDE)
HI ,THANK YOU FOR YOUR EXAMPLE OF K TYPE MAX6675
IF I WANT TO MAKE MULTIPLE OF MAX 6675 3 SET , 1 OLED DISPLAY 6 PINE AND ARDUINO UNO WHAT COULD BE THE CODE ?
THANKS FOR YOUR ASSISTANT.
My stupdig sensor is WAY too high or too low maybe nbacause with the teawater it was low but now high the air
This type K thermocouple provided with MAX6675
has a blue lead (crimp color and wire insulation) and a red lead (crimp color and wire insulation.
I see that in the photo the blue lead is connected to -, red lead to +. This seems wrong–all thermocouple types use red lead as – (negative)
Please enlighten us all, thank you.
I have not seen anywhere connections that you mentioned. It worked perfectly fine for us by connecting blue lead with – and red with +.
Minus temp is not working
How to read temp below 0??
Now it stop at 0