In this tutorial, we will learn how to build an android app that will enable us to send messages to Arduino via Bluetooth to be displayed on a 16×2 LCD. This android app will be created with MIT App Inventor. The transmission of the message will be through the HC-05 Bluetooth module.
Send Messages to Arduino using Android App Project Overview

We will create an Arduino IoT app through HC-05 and MIT App Inventor to display messages on a 16×2 LCD. The android app features a text box which allows the user to type a message to be sent to Arduino. The ‘Send Message’ button will be used to send the message to Arduino via Bluetooth. The message will then be displayed on a 16×2 LCD connected with Arduino.
To enable Bluetooth connectivity, there is a button saying ‘Connect Bluetooth’ that opens a List picker which opens up Bluetooth devices currently paired with the android phone. You can select HC-05 Bluetooth module from the list. Another label saying Bluetooth is Connected or disconnected is also shown on the screen.
We will require the following for our project:
Hardware Required:
- Arduino UNO
- 16×2 LCD
- Potentiometer
- HC-05 Module
- Connecting Wires
- Breadboard
Software Required:
- Arduino IDE
- MIT App Inventor
- Android Smartphone with MIT AI2 Companion installed
Liquid Crystal Display Introduction
As its name suggests LCDs are electronics devices that are used to display texts, custom characters, and numbers. Although, we can also display pictures on LCDs. But results will be not comparable to graphical LCDs (GLCDs). GLCDs are used to display images. Coming back to LCDs, they are available in different sizes and features. For example, 16×2, 20×2, 16×1 are different sizes available.
Types
They come in two types serial and parallel LCDs. Serial type uses serial communication, such as the UART module, to interface with Arduino. They are easier to interface with Arduino than parallel LCDs. But they are expensive. In contrast, parallel LCDs, for example, Hitachi HD44780, are exceptionally used and interfacing with Arduino is done using digital I/O pins of Arduino. For instance, Hitachi HD44780 type 16×2 LCD can be interfaced with Arduino using 4-8 data lines and few control pins of the display. In this tutorial, we will be using 16×2 parallel LCD only. Because it is the most popular choice among the Arduino community and embedded application developers.
16×2 LCD Introduction
There are two types pins on the whole 16×2 LCD module. Some pins are used to send to 16×2 LCD and some are command pins. In other words, every pin has a role in controlling a single pixel on the display.
16 x 2 LCD has sixteen columns and two rows. That means, it can display sixteen characters per row and it has two such rows. Similarly, 20×4 LCD has four rows and 20 columns. That means, it can display 20 characters per row.
Pinout Diagram
The diagram shows the pin configuration of 16×2 display. It has sixteen pins.
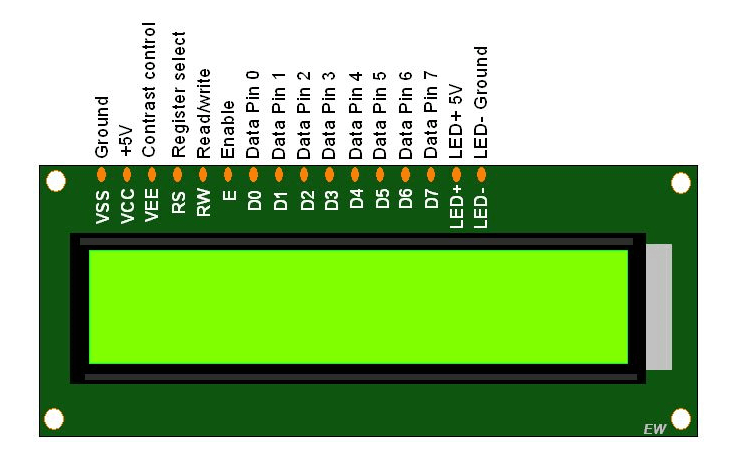
- D0 – D7: Pin number 7-14 are data bus lines that are used to send data from Arduino which you want to display on LCD. With these 8 data lines, data can be transferred either in an 8-bit format or in a 4-bit format. In a 4-bit format, only upper four bits (D4-D7) are used to send data from Arduino to LCD. The full byte is transmitted in two successive transmissions. A 4-bit format is used to save GPIO pins of Arduino. Because fewer GPIO pins of Arduino will be required to transfer data.
- Contrast Select (VEE): It will help to control the contrast of PIXELS according to the 16X2 LCD light.
- RS: This pin is known as a register select pin. It helps to toggle the command/data register.
- R/W: The signal on this pin will decide whether it is going to read from LCD or write on it.
- EN: Enable pin will help to transfer the instruction from the data pins and another command pin to the LCD. It act as permission to internal registers.
- VSS: It’s a ground pin for common grounds.
- VCC: The power pin will use for voltage input to the 16X2 LCD.
In Arduino, we don’t need to worry about controlling these data lines and control registers. Because Arduino provides rich library resources. LCD library comes with Arduino IDE by default when we install it.
Interfacing 16×2 LCD and HC-05 module with Arduino board

We are using the following connections for 16×2 LCD and Arduino as described below. Refer to the schematic diagram to have a clearer idea of the connections.
16×2 LCD | Arduino |
---|---|
D4 – D7 | Pin 5,6,7,8 |
Enable | Pin 4 |
RS | Pin 3 |
RW | GND |
VEE | 10k POT (Middle Leg) |
VSS | GND |
VCC | +5V |
LED+ | +5V |
LED- | GND |
Follow the connections to connect HC-05 module with Arduino:
- Bluetooth Tx with Arduino UNO Rx (D0)
- Bluetooth Rx with Arduino UNO Tx (D1)
- Bluetooth VCC with Arduino UNO +5V
- Bluetooth GND with Arduino UNO GND
The schematic diagram below shows the connection between 16×2 LCD, HC-05 and Arduino UNO.

NOTE: Make sure you plug out the Tx and Rx pins out of Arduino before uploading the program. After uploading the program connect them back. Otherwise, you can get an error.
You may also like to read:
Arduino Sketch Display Messages
Open your Arduino IDE and go to File > New to open a new file. Copy the code given below in that file.
#include <LiquidCrystal.h>
#define maxChar 32
char message[maxChar]; // stores the message
char readChar; // reads each character
byte index = 0; // defines the position into your array
int i;
LiquidCrystal lcd(3, 4, 5, 6, 7, 8);
void setup() {
Serial.begin(9600);
lcd.begin(16, 2);
delay(1000);
}
void loop() {
if (Serial.available()) {
for (i = 0; i < 31; i++) {
message[i] = '\0';
}
index = 0;
}
while (Serial.available() > 0) {
//the message can have up to 100 characters
if (index < (maxChar - 1)) {
readChar = Serial.read(); // Reads a character
message[index] = readChar; // Stores the character in message array
index++; // Increment position
message[index] = '\0'; // Delete the last position
}
}
lcd.clear();
lcd.print(message);
for (int positionCounter = 0; positionCounter < i / 2; positionCounter++) {
delay(750);
lcd.scrollDisplayLeft();
}
}
How the Code Works?
We will start by including the library required for our 16×2 LCD as shown below:
#include <LiquidCrystal.h>
Next, we declare the Arduino pins that are connected with the LCD. To define connections, we use the following line of code. This line creates a LiquidCrystal object and lcd is a name of the object that we are going to use to call LCD functions. You can also use any other name.
LiquidCrystal lcd(rs, en, d4, d5, d6, d7)
In our case, the pins are 3, 4, 5, 6, 7, and 8 respectively.
LiquidCrystal lcd(3, 4, 5, 6, 7, 8);
Inside the setup() function, we will open a serial connection at a baud rate of 9600. Next, we use lcd.begin() routine to define the size of an LCD. The first argument to this function is a number of rows and the second argument is a number of columns.
void setup() {
Serial.begin(9600);
lcd.begin(16, 2);
delay(1000);
}
Inside the loop() function, we will first check if data is available in the serial port. If data is available then we will first reset our message array. After that, read the characters in the serial port and and store them in the array message. Then display the message on the LCD in a scroll left manner.
void loop() {
if (Serial.available()) {
for (i = 0; i < 31; i++) {
message[i] = '\0';
}
index = 0;
}
while (Serial.available() > 0) {
//the message can have up to 100 characters
if (index < (maxChar - 1)) {
readChar = Serial.read();
message[index] = readChar;
index++; // Increment position
message[index] = '\0'; // Delete the last position
}
}
lcd.clear();
lcd.print(message);
for (int positionCounter = 0; positionCounter < i / 2; positionCounter++) {
delay(750);
lcd.scrollDisplayLeft();
}
}
Building App with MIT APP Inventor
MIT App Inventor is an incredible web application that helps users build interesting Android applications. It is a block-based programming tool through which users can create fully functional apps for Android devices such as smartphones, tablets e.tc. Often termed beginner-friendly, even people with no prior experience in programming can easily learn how to use this application efficiently. We will use this tool to build our Arduino app as well.
Steps to Create Android App
Go to the following website: and click the ‘Create Apps!’ button.

You will be redirected to a new window where you will be asked to sign in with your email account. After signing in you will have to accept the terms as shown below.
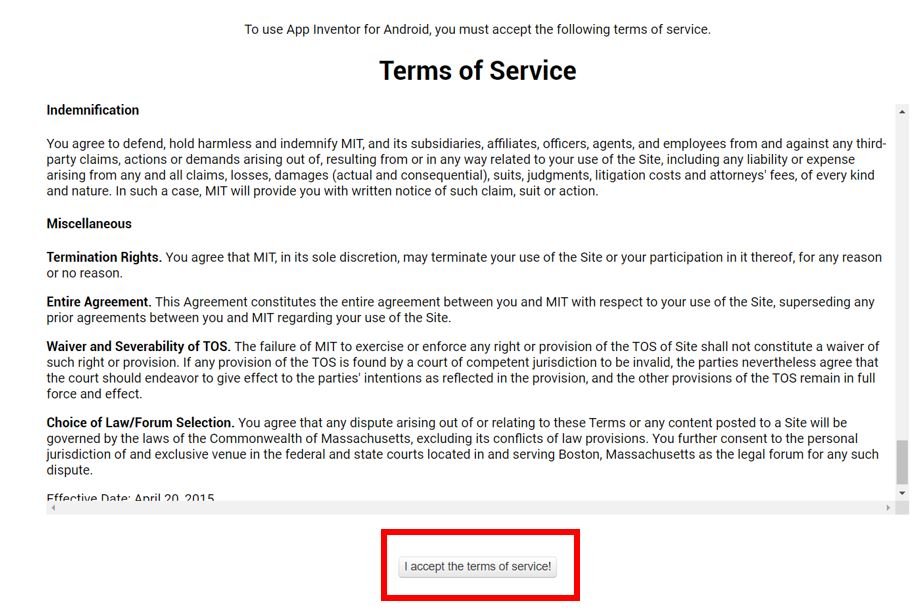
You will receive a welcome message. You can visit the link to get more information on how to install the inventor on your smartphone. Press ‘Continue’ to proceed. If you want, you can have a look at the tutorials being specified. Otherwise, go to ‘Start a blank project’ to start your app invention.

You will be asked to specify your project name. Choose an appropriate name. Click ‘ok.’

The following page will open. It is known as the Designer. This is where we will design the user interface of our app. We can add text, buttons, images and various other functionalities to our app by dragging and dropping components from the palette to the viewer. Then we will set their attributes through the ‘Properties’ column.
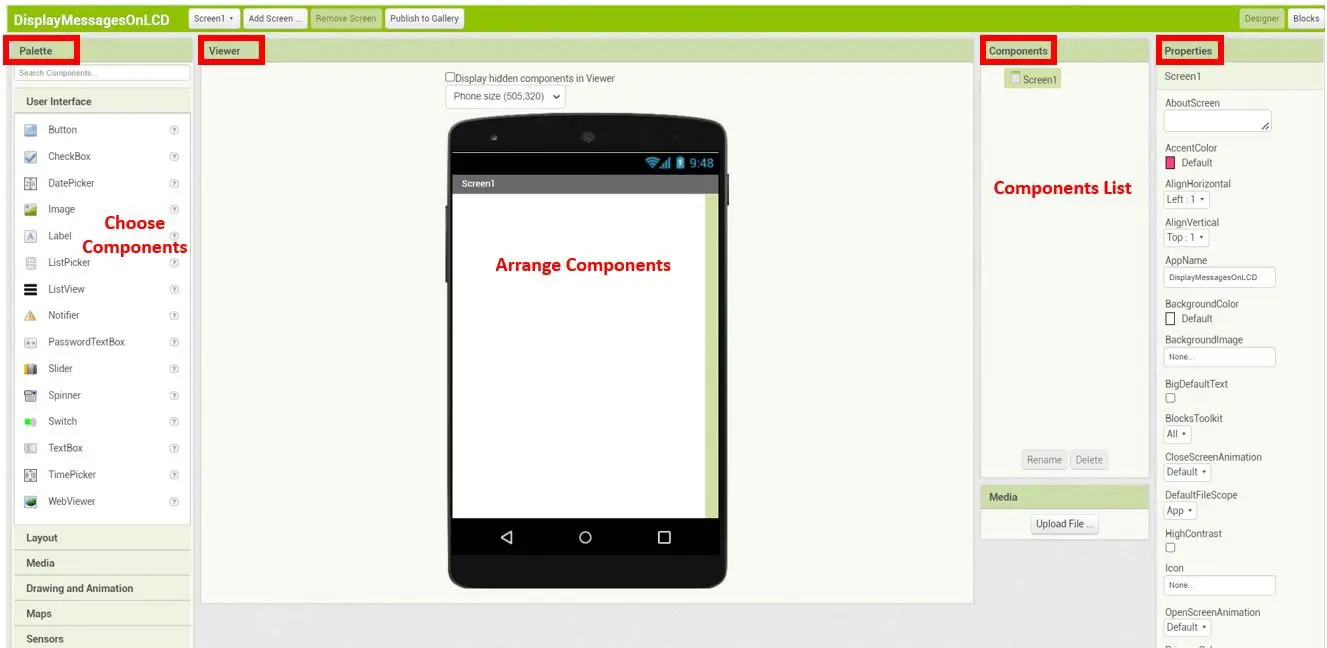
Creating User Interface
Go to Palette > User Interface and head over to ‘TextBox.’ Now drag and drop it on the Viewer side. Now you will be able to view it in the viewer as well as in the components list. We have renamed it ‘TextBoxMessage’ as shown in the Components sections. The Properties section shows the properties we have set for this arrangement.
Keep in mind the viewer will show you the final look of your app which will appear on your smartphone.

Next select VerticalArrangement from the Layout. Rename it ‘VerticalArrangementButtons‘ and set the Properties as shown below.
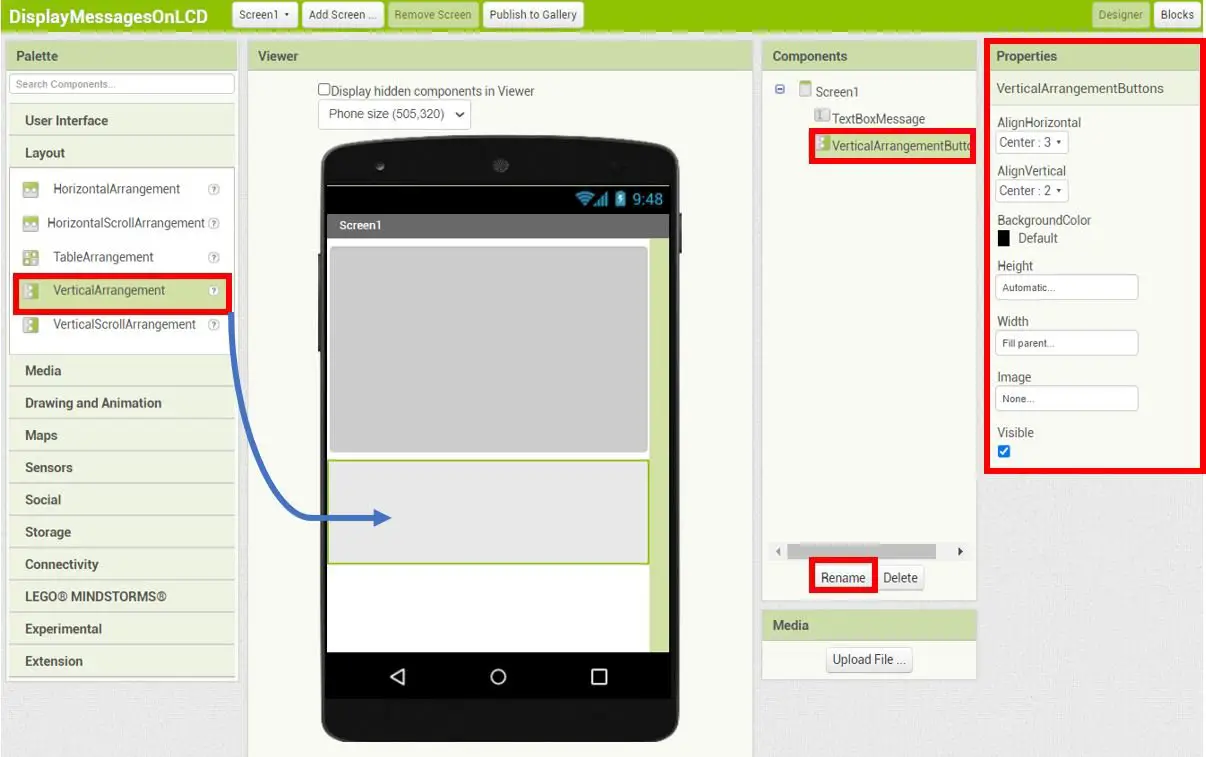
Go to User Interface > Button and drag and drop a button in the VerticalArrangementButtons arrangement. Rename the button as ‘SendMessageButton.‘ Moreover, change the properties of the button as well. This button will send the message entered by the user in the text box to Arduino.

Next add a label beneath the SendMessageButton and rename it as ‘BluetoothConnection‘ using the rename button found in the Components section.
Head over to the ‘Properties’ to change the text, font, font size and color of the this label. We have set the FontSize to 14, FontTypeface to serif, Height to Automatic, Width to Automatic, Text to Disconnected, TextAlignment to center : 1 and TextColor to None.

For the Connect Bluetooth button we will add a List Picker. Go to User Interface > ListPicker and drag and drop it beneath the BluetoothConnection label. Go to the ‘Properties’ of the ListPicker and set them as follows:

Add Bluetooth Client and Clock to MIT App Inventor
Go to Palette > Connectivity and click and drag BluetoothClient in the viewer. This is a non-visible component of our app and will let us connect it with our Bluetooth module.
Next head over to Palette > Sensors and drag and drop clock in the viewer. Rename it as ‘BluetoothClock.’ This is also a non-visible component of the app used for monitoring the time.

Blocks Layout
Now click on the ‘Blocks’ button found at the top of the window. A new editor will open up. Here we will design how our app will respond. We will assemble blocks in the workspace by clicking and dragging them.

Firstly, assemble the blocks for the Bluetooth connection as shown below.

Next, assemble the blocks for SendMessageButton as shown below. When the Send Message button is clicked, the text in the TextBoxMessage will be sent to Arduino.

For more information regarding building your app in MIT App Inventor, follow this guide: App Inventor Tutorials.
Setting up App Inventor on Smartphone
Now, as we have built our app in MIT app inventor and have also written its code let’s move ahead and install the App Inventor in our smartphone. Through this, we will be able to access our app on our smartphone from anywhere around the world. Follow the steps closely.
Firstly, go to Play Store and install ‘MIT AI2 Companion.’

After you installed the application, open it. You will have to either scan a QR code or type a 6-character code.

To access these, go to MIT App Inventor main page where we initially built our app. Go to Build > Android App (.apk). After a few moments your barcode will get generated. You can either download the .apk file or scan the barcode using the MIT App Inventor.

After installing the .apk file on our android phone, we will be able to view the screen which we created.
Now, we will demonstrate the project.
Demonstration
Choose the correct board and COM port before uploading your code to the board.
Go to Tools > Board and select Arduino Module.

Next, go to Tools > Port and select the appropriate port through which your board is connected.
Click on the upload button to upload the code into the Arduino development board. After you have uploaded your code to the Arduino development board press its ENABLE button.

Open the MIT Companion app on your smartphone. Pair your android phone with HC-05 module. Now press the ‘Connect Bluetooth’ button.

Select HC-05 Bluetooth module to connect with.


Now type a message in the textbox and click the Send Message button. The LCD will display the message automatically.

Video demo:
You may also like to read:
- Arduino RGB LED Control using Android App with MIT App Inventor
- Control ESP32 over Internet using Android App with MIT App Inventor
- IoT CCTV Camera using ESP32 CAM & Blynk App – Live Streaming
- Arduino Send Sensor Readings to Android app with MIT App Inventor
- Control ESP8266 Outputs using Blynk App and Arduino IDE
- ESP32 Send Sensor Readings to Google Firebase and Display on Android app
Thank you for the insight. Please how do I clear the text from the textbox when it sent with the app
how have you create schematic
How do i make the app when i use ESP8266? I don’t know the blocks on mit app inventor on sending and receiving from a webserver